Table of contents
How to integrate with the SharePoint API via Python
.jpg)
Microsoft SharePoint is more than a file storage system—it's a powerful hub for collaboration, document management, internal workflows, and team communication.
You can also improve your product and your internal workflows by integrating with SharePoint’s API—from powering your product’s enterprise AI search functionality to enabling employees to automatically create folders for certain types of recorded calls.
No matter your integration use case(s) with Sharepoint, we’ll help you get started by walking through how you can build to Sharepoint’s API endpoints via Python.
Prerequisites
Before you begin, you’ll need the following:
- A Microsoft 365 (SharePoint Online) account. Make sure you have access to a SharePoint Online environment, including permissions to read, upload, and manage files. You or your SharePoint administrator must also have sufficient permissions to register applications within Microsoft Entra ID and grant API access to SharePoint resources
- Python 3.x installed. Verify that Python 3 and the pip package manager are installed and configured on your system
Installation and authentication with the SharePoint API in Python
To interact seamlessly with SharePoint through Python, you need a specialized library: Office365-REST-Python-Client.
This library simplifies authentication, abstracts away HTTP requests, and provides easy-to-use functions for interacting with SharePoint files, lists, and metadata.
Install it with the following command:
Always consider installing packages within a Python virtual environment (venv, virtualenv, or conda). This isolates your project's dependencies and ensures consistent versions across development, testing, and production environments.
https://www.merge.dev/blog/file-storage-api?blog-related=image
App-based authentication
To securely access SharePoint resources via the API, you'll use app-based authentication. This method leverages a dedicated application identity (defined by a client ID and client secret) to connect to SharePoint.
App-based authentication is designed for automation and integration, helping keep user credentials private while giving you more control over permissions. It lets you give your Python app the access it actually needs, minimizing risk and supporting current enterprise security standards.
Here's how to set up app-based authentication:
Create a new Microsoft Entra ID app by logging into the Azure Portal and navigating to Microsoft Entra ID > App Registrations:

Select New registration, enter your app name, leave all other fields in their default state, and click the Register button to register a new application.
To get your client ID, inside your app, find the Overview screen and click Essentials:

Find the Application (client) ID value and save it. This is your client ID.
Then, generate a client secret by navigating to Certificates & secrets under the Manage accordion:
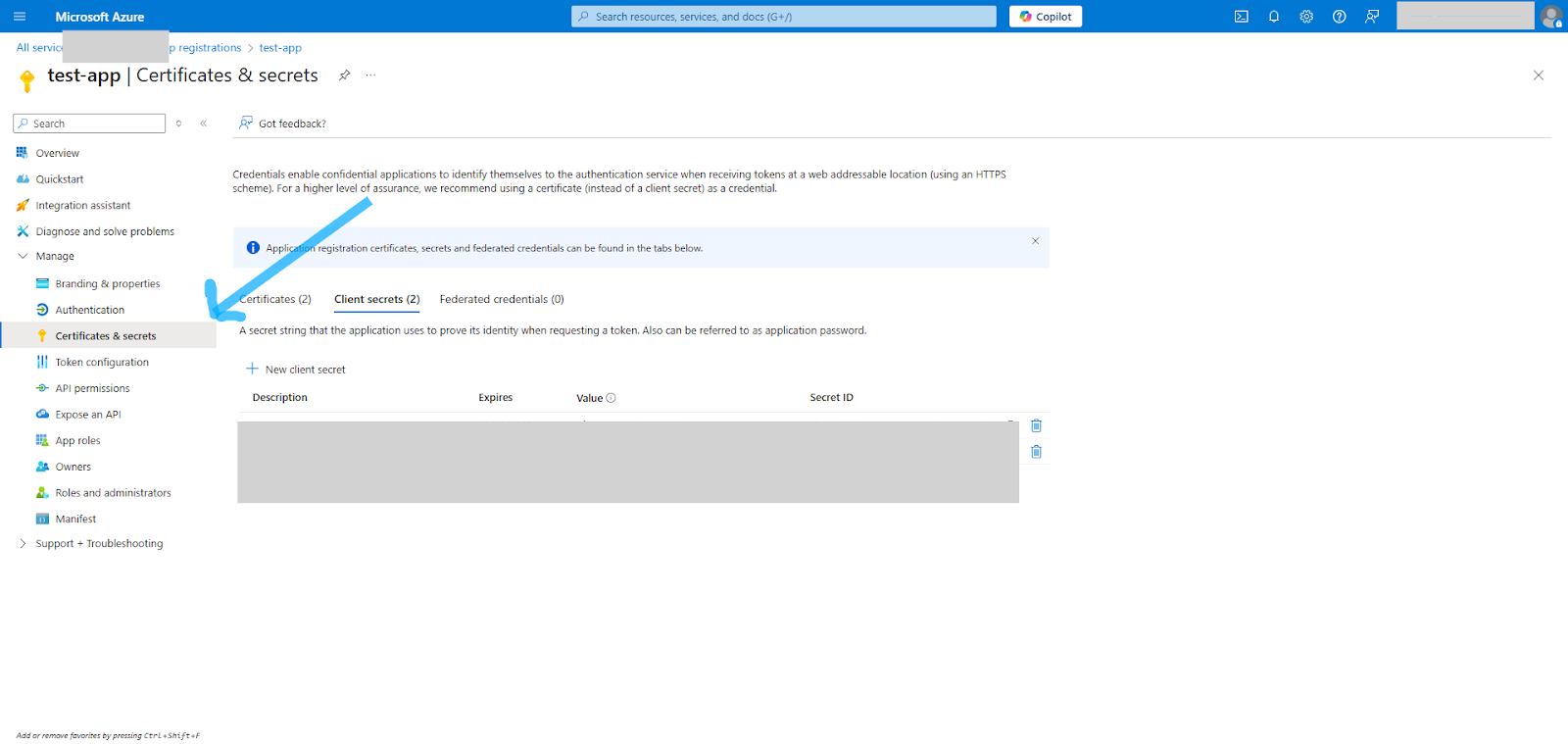
Click New client secret, specify an expiry, and copy the generated secret immediately.
To assign permissions, go to the Manage accordion again and select API permissions:

Click Add a permission, navigate to the SharePoint option, and select Application Permissions. Add the SharePoint permission scope (<code class="blog_inline-code">Sites.ReadWrite.All</code> or <code class="blog_inline-code">Sites.Manage.All</code>) and grant admin consent.
Make sure you store the client ID and secret securely (using environment variables or a secure secrets manager) and never commit them directly to source control. In the next step, you'll use the client ID and secret to authenticate with SharePoint using <code class="blog_inline-code">ClientContext</code> from the <code class="blog_inline-code">Office365-REST-Python-Client</code> package.
Authenticate with ClientContext
<code class="blog_inline-code">ClientContext</code> is your authenticated session with SharePoint. It manages authentication tokens, session lifecycles, and API call batching. It handles these low-level complexities behind the scenes so your code stays clean and focused, and you can perform actions securely. Once you set it up, you use this context to interact with your SharePoint data.
Here's how to authenticate using app-based authentication:
This code sets up the SharePoint session using <code class="blog_inline-code">ClientContext</code> and your app credentials. The <code class="blog_inline-code">.execute_query()</code> runs the request, confirming the connection by printing the site title.
Make sure to replace <tenant> with your Microsoft tenant name and <your-site> with your SharePoint site name. For example, if you create a SharePoint site named reporting and the URL for that site is https://my-company-123.sharepoint.com/sites/reporting, my-company-123 is your tenant name and reporting is your SharePoint site name.
Note: If you're using SharePoint Online and encounter a 401 error, your environment may require certificate-based authentication. For guidance on generating a certificate and configuring it within Microsoft Entra ID, refer to these instructions. Make sure to replace contoso.onmicrosoft.com with your tenant name instead (eg <tenant-name>.onmicrosoft.com).
After you're done generating and uploading the certificate, you can run this code:
It's similar to the preceding code, but this one uses a certificate file for authentication (common in high-security setups) instead of a <code class="blog_inline-code">CLIENT_SECRET</code>.
Core data transfer operations with the SharePoint API
Once authenticated, here's how you perform essential file operations:
Downloading files
To download a file from SharePoint, simply specify the file path on the server:
This script downloads a SharePoint file by its server-relative path and saves it locally. Always ensure paths are correct and permissions allow read access.
It's important to note that SharePoint uses paths relative to your site URL (e.g., "/sites/<your-site>/Shared Documents/Reports/sales_data.csv"). This simplifies your code and reduces URL management overhead.
Uploading files
Uploading files is equally straightforward:
This code snippet reads a local file and uploads it to the specified SharePoint folder, making it available to your team instantly.
Reading files into memory (for ETL)
For data-heavy workflows, you often need direct file access without local storage:
This code loads SharePoint files directly into memory using <code class="blog_inline-code">File.open_binary()</code> and converts the bytes into a pandas DataFrame with <code class="blog_inline-code">pd.read_csv(io.BytesIO(file_content))</code>.
This approach eliminates local file storage, reduces processing time, and ensures you're working with the latest data. This is ideal for extract, transform, and load (ETL) pipelines and analytics workflows.
Writing data directly to SharePoint (from pandas DataFrame)
You may also need to write the data back to SharePoint after processing it, especially in reporting or analytics workflows. Instead of saving your pandas DataFrame to a file and uploading it separately, you can upload it directly from memory using a BytesIO stream:
This code converts your DataFrame directly to a CSV-format byte stream in memory and then uploads it to SharePoint without saving to disk first. This approach is faster, reduces I/O overhead, and enables fully automated end-to-end workflows.
With these core actions mastered, you're ready to build powerful workflows, automations, and integrations between SharePoint and your Python-based systems.
What you can do with the SharePoint API access
Using Python to connect with the SharePoint API lets you complete many practical use cases, including automating repetitive tasks, enhancing data accessibility, and building custom solutions. Here are a few practical examples:
Automated weekly CSV imports
Stakeholders often drop new CSVs, such as sales numbers, survey results, and inventory counts, into a shared folder. You can have a Python job run on a schedule, detect only the latest files (using a simple Modified >= last_run filter), and load the data straight into your database or ETL pipeline. No more Monday-morning download-and-upload rituals.
Enterprise reporting pipelines
Finance, operations, or project teams store Excel workbooks and PDFs in SharePoint. When you pull these files via the API, you can schedule automated dashboard refreshes in tools like Power BI or Tableau and then push the rendered reports back to SharePoint for easy access. This provides stakeholders with the latest available insights and eliminates the need for manual data extraction and uploading.
ML and AI workflows
SharePoint can be a valuable source of data for ML and AI applications. You can use SharePoint as a curated data lake: store labeled images, CSVs, or text files for model training and then let a script feed them into your ML pipeline. After inference, drop prediction outputs (e.g., predictions.csv) right back into SharePoint so business users can review results without leaving their familiar workspace.
Content migration and backup
When you need to retire an old site or comply with audit requirements, the API allows you to programmatically migrate or archive thousands of documents. Loop through folder.files, copy them to a cloud storage, or generate ZIP archives for long-term retention. This technique is far safer and faster than manual drag-and-drop.
Enterprise AI search
Say you offer an enterprise AI search product, like Guru or Glean.
To help your customers' employees receive answers to all kinds of questions, you can integrate with their Sharepoint instances and sync their file contents with your product on a recurring cadence.
That way, when employees ask all kinds of questions (e.g., "What's our PTO policy?"), your AI assistant can leverage the integrated file contents to answer the question as well as provide the file(s) that was used to generate the response.
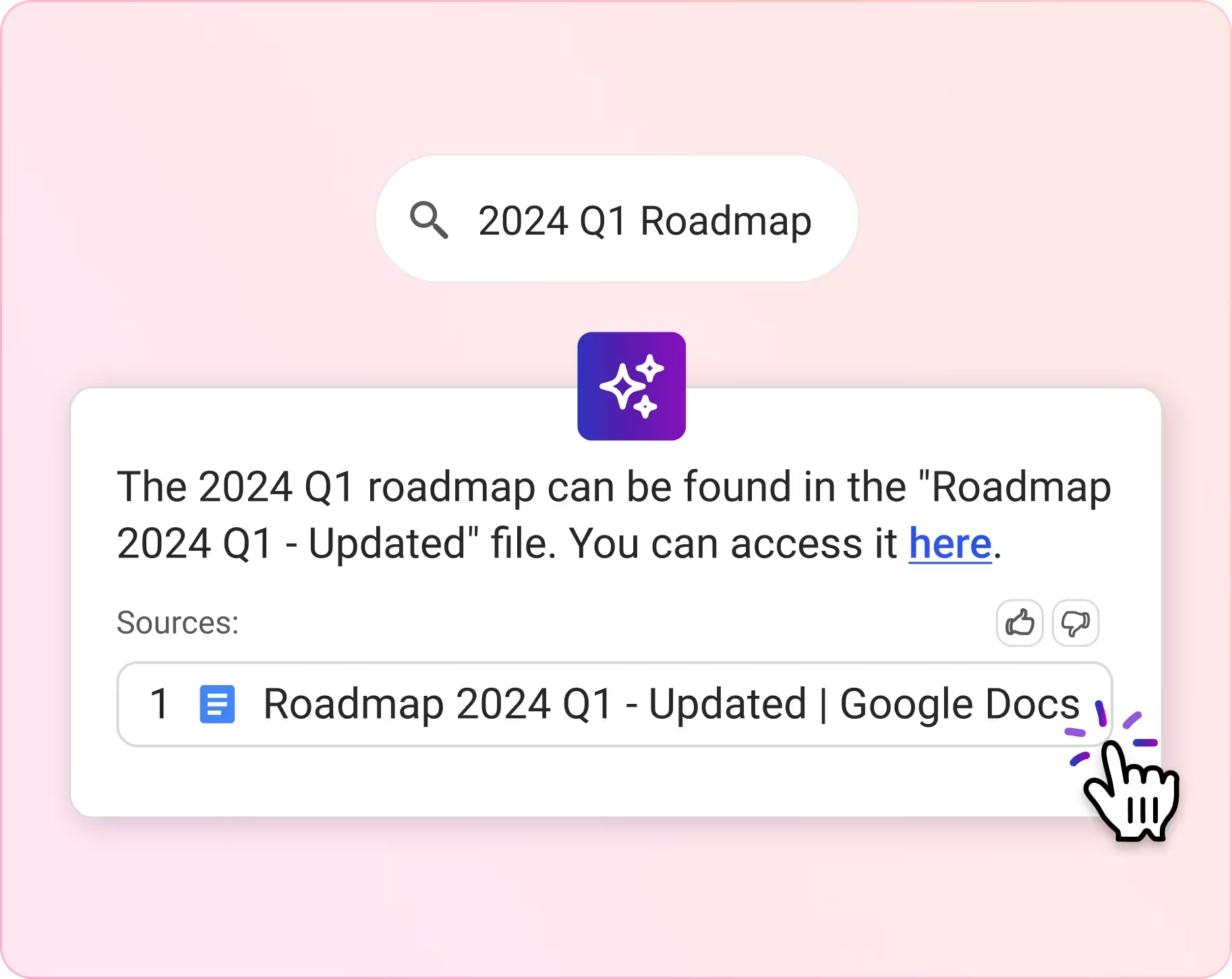
https://www.merge.dev/blog/ai-agent-integrations?blog-related=image
Advanced considerations for a production-ready integration
Basic data transfer operations provide a solid foundation for SharePoint integration, but building robust and production-ready solutions typically requires a few additional considerations.
Incremental data loading
Always fetch only the data that has changed. Use SharePoint's OData filters <code class="blog_inline-code">(Modified ge datetime'{last_run}')</code> or Microsoft Graph delta queries so nightly jobs process kilobytes, not gigabytes.
Caching and temporary storage
Hot files that get read repeatedly should be cached—either in a temp directory or with an in-memory decorator like <code class="blog_inline-code">functools.lru_cache</code>. Fewer API calls mean faster scripts and a lower risk of SharePoint throttling.
Robust error handling and logging
To avoid network glitches and 429 Too Many Requests errors, wrap critical calls in a retry utility like Tenacity, backoff, or your own exponential-backoff helper. Log meaningful details like status codes or response text so failures surface quickly and debugging is painless.
Security and compliance best practices
When working with sensitive business data, securing your integration is just as important as making it work. Always load secrets from environment variables or a vault, rotate them regularly, and scope app permissions to only the sites or libraries you need.
Performance tuning for Scale
For multi-GB files, switch to chunked transfers. For thousands of small files, bundle requests into SharePoint batch calls or run them in parallel with <code class="blog_inline-code">concurrent.futures</code>, always mindful of throttling limits. Test under real-world loads, tune, and retest.
Integrate Sharepoint—and any other file storage solution—with your product via Merge
As you can tell from this article, integrating with Sharepoint—and maintaining the integration—is complex and time intensive.
When you couple this with all of the other file storage solutions you need to integrate with your product, like Google Drive, OneDrive, Box, and Dropbox, the workload only increases exponentially.
To help you add all of these integrations quickly and with minimal resource investment, you can leverage Merge, the leading unified API solution.
Simply build to Merge's File Storage Unified API to access all of the file storage integrations you need.

Merge also offers advanced features to sync any custom data in your customers' file storage systems; observability tooling to empower your customer-facing teams to manage these integrations; enterprise-grade security features to only sync the data that's absolutely necessary (e.g., Common Model Scopes) and keep the data secure, and much more.
Learn how Merge can help you integrate with Sharepoint and the rest of your customers' file storage systems by scheduling a demo with one of Merge's integration experts.