How to GET repositories using the GitHub API in Python
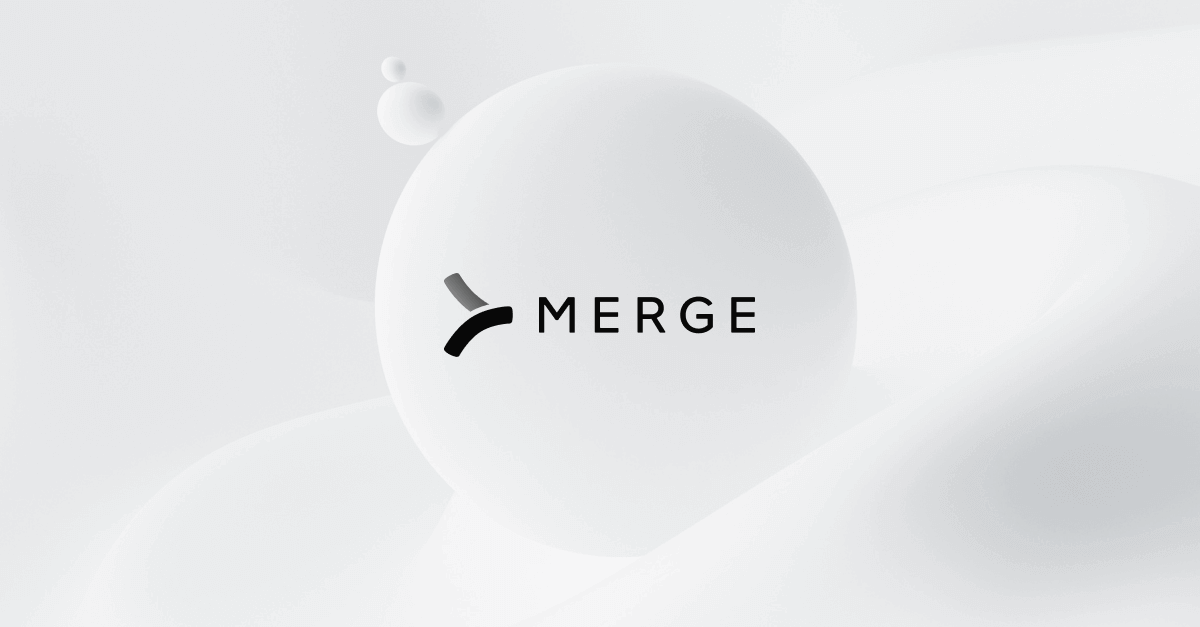
GitHub allows you to find all kinds of resources and code on their platform, which includes the source code for entire websites, applications, servers, and games.
The source code on GitHub is most commonly stored in “repositories”. As a result, it can be useful to fetch and read information on specific GitHub repositories.
In this article, we’ll walk through how you can do just that. This includes how you can generate the credentials needed for making authenticated HTTP requests to GitHub’s Repositories API endpoint, and writing the code for retrieving the information using Python.
Prerequisites
To follow along this guide you’ll need:
- A valid GitHub account
- Python version 3.10 or later
- The python requests library installed in your environment (like your laptop)
Authenticating to the GitHub Repositories API
There are 3 options you can use to authenticate to the GitHub Repositories API endpoint:
1. Fine-grained personal access tokens
Fine-grained personal access tokens function similarly to bearer tokens, but they provide certain security advantages. More specifically, a fine-grained personal access token allows you to easily configure the resources it can access as well as revoke access if and when needed.
Note: We’ll be using this token for our walkthrough. You can learn more about generating a fine-grained personal access token in GitHub’s docs.
2. Github user access token
In GitHub, a user access token functions much like an OAuth token. Unlike a normal OAuth token however, GitHub’s user access tokens also have fine-grained permissions.
Learn more about generating a user access token.
3. App installation access tokens
If you want to authorize HTTP requests on behalf of a GitHub application, you can use an app installation access token.
This is useful for installing applications in your repositories that can, for example, help keep the versions of any installed packages up to date automatically.
Learn more about authenticating as a GitHub App installation.
Generating a fine-grained personal access token
There are many public repositories on GitHub that we can fetch high-level information on. However, if you want access to detailed information on a repository or information about a private repository, you’ll need to make sure you have some form of authentication that has permission to read that data.
You can do the latter by generating a fine-grained personal access token. Here’s how:
- Verify your GitHub account’s email address (if it hasn't been verified yet).
- In the upper-right corner of any page, click your profile photo, then click Settings.
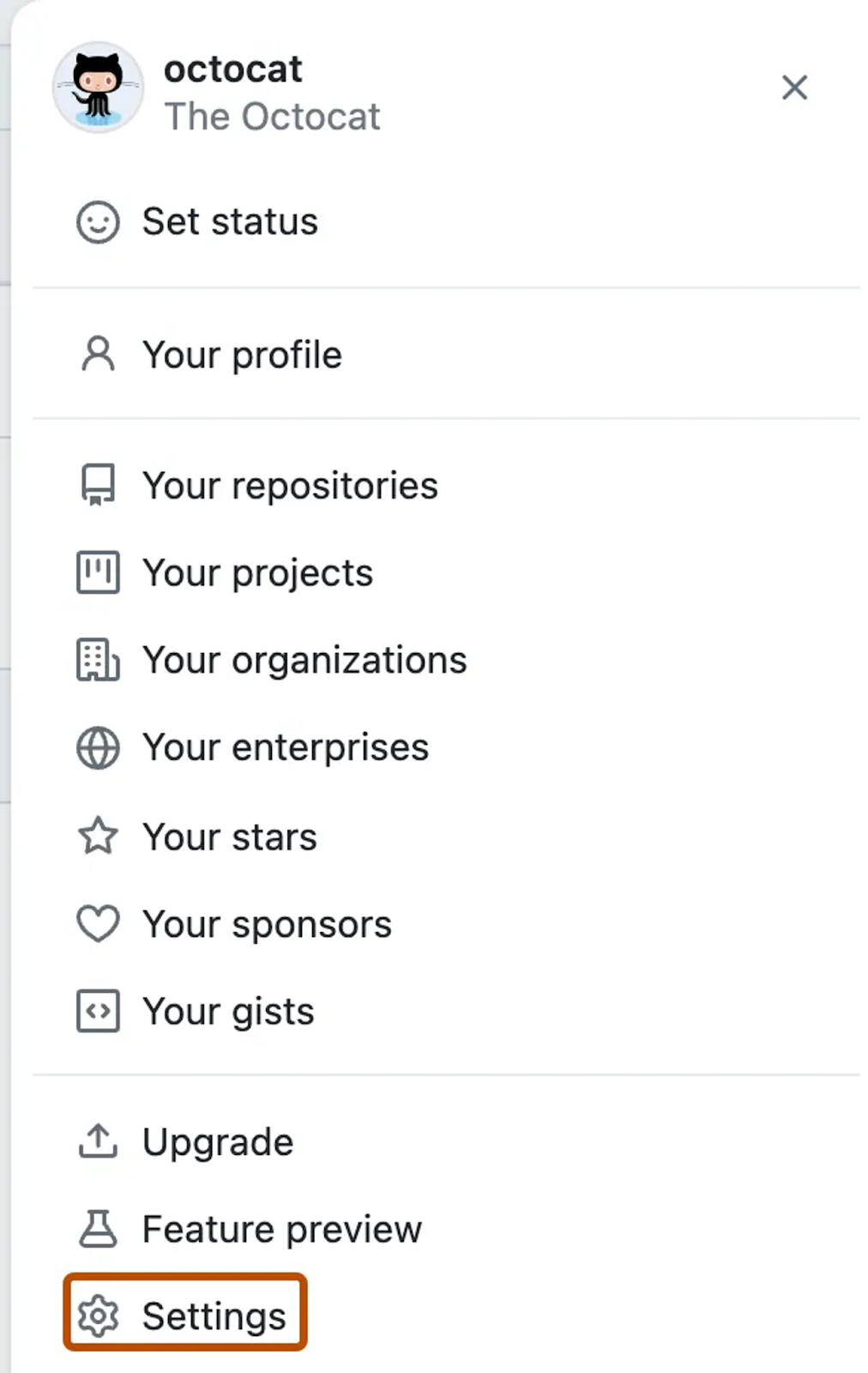
3. In the left sidebar, click Developer settings.
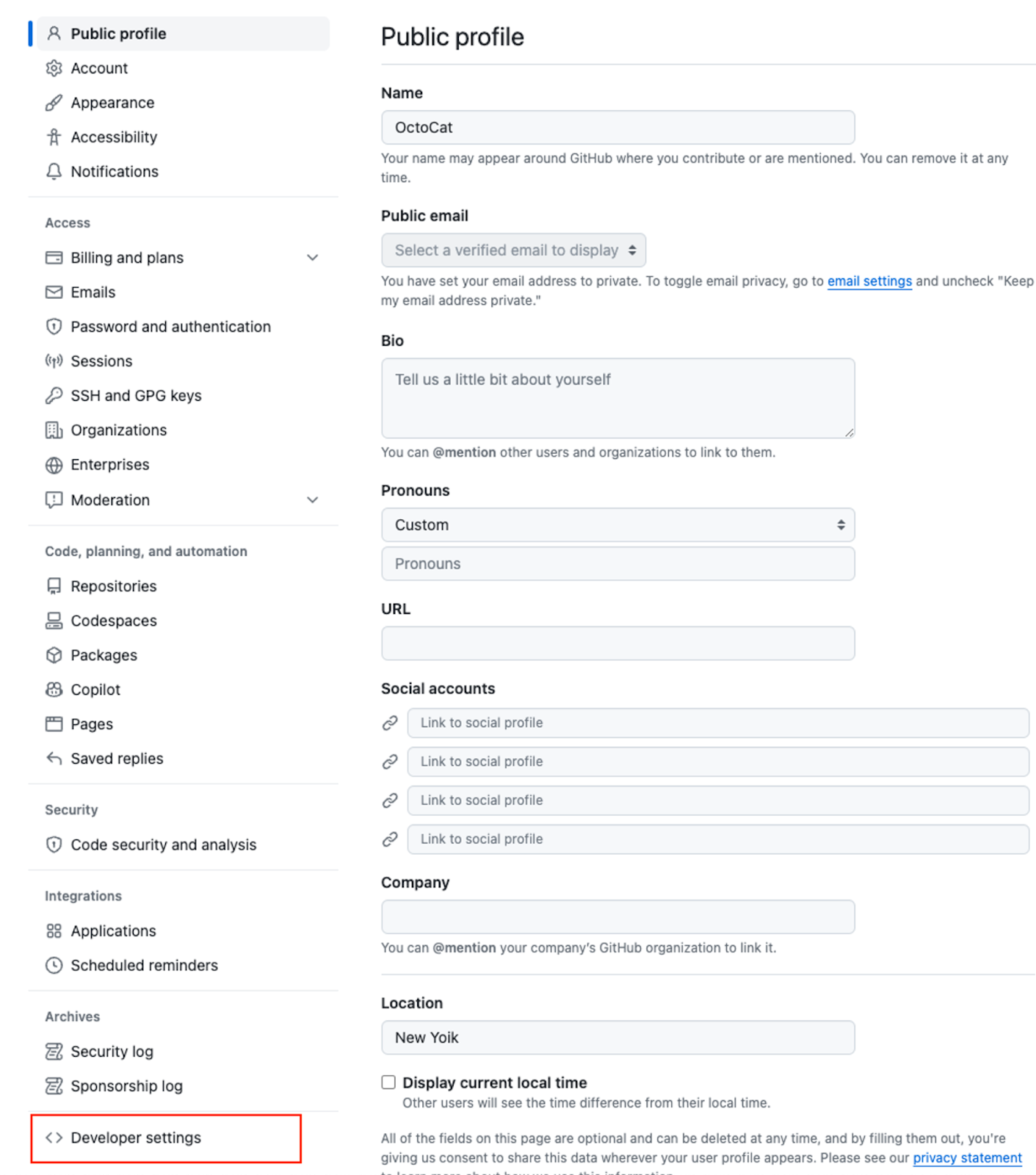
4. In the left sidebar, under Personal access tokens, click Fine-grained tokens.

5. Click Generate new token.
6. Under Token name, enter a name for the token.
7. Under Expiration, select an expiration for the token.
8. (Optional) Under Description, add a note to describe the purpose of the token.
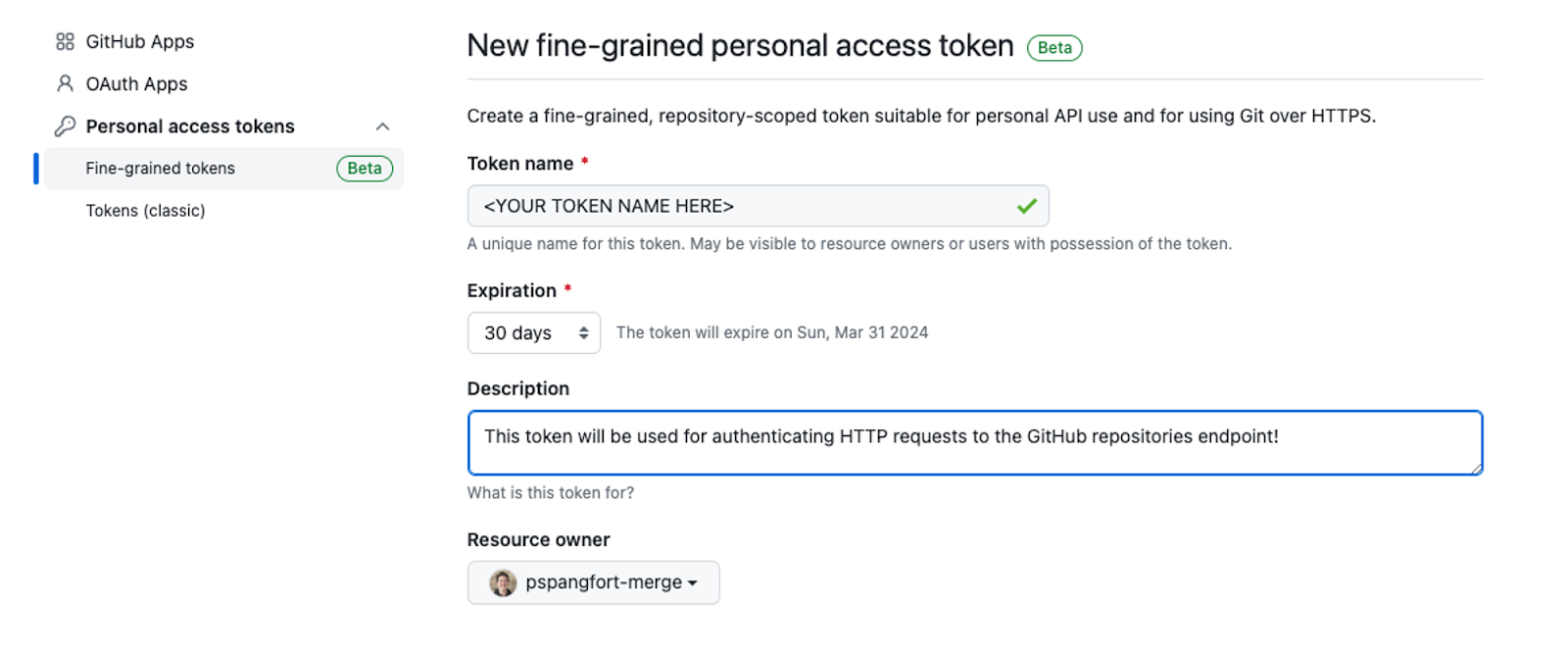
9. Under Resource owner, select a resource owner. The token will only be able to access resources owned by the selected resource owner. Organizations that you are a member of will not appear unless the organization opted in to fine-grained personal access tokens. You can learn more about setting a personal access token policy for your organization here.
10. Under Repository access, select which repositories you want the token to access. You should choose the minimal repository access that meets your needs. Tokens always include read-only access to all public repositories on GitHub.
11. If you selected Only select repositories in the previous step, under the Select repositories dropdown, select the repositories you want the token to access.
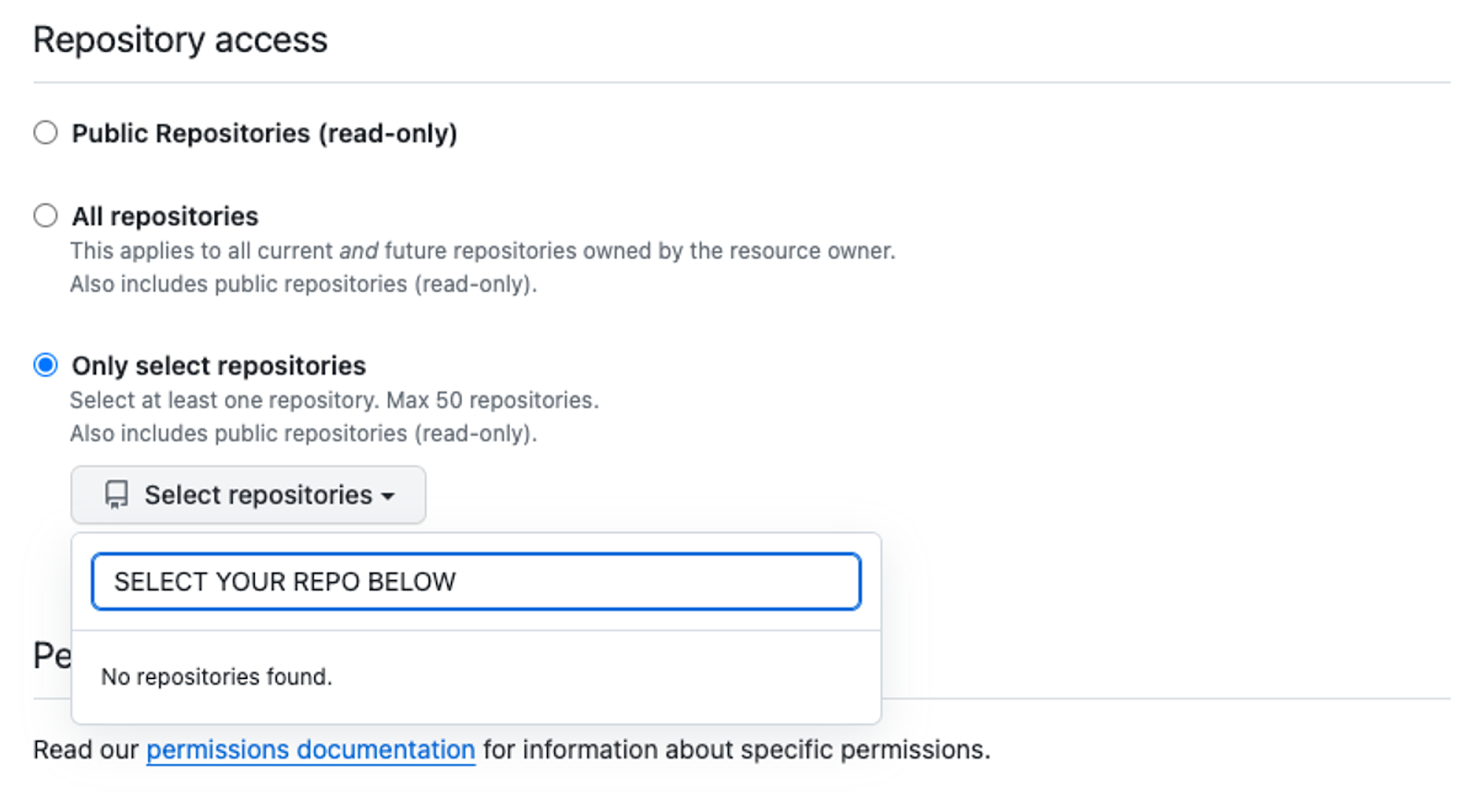
12. Under Permissions, select which permissions to grant the token. Depending on which resource owner and which repository access you specified, there are repository, organization, and account permissions. You should choose the minimal permissions necessary for your needs. In this example, I’ll be generating a token that has read-only access to the contents of my selected repository.
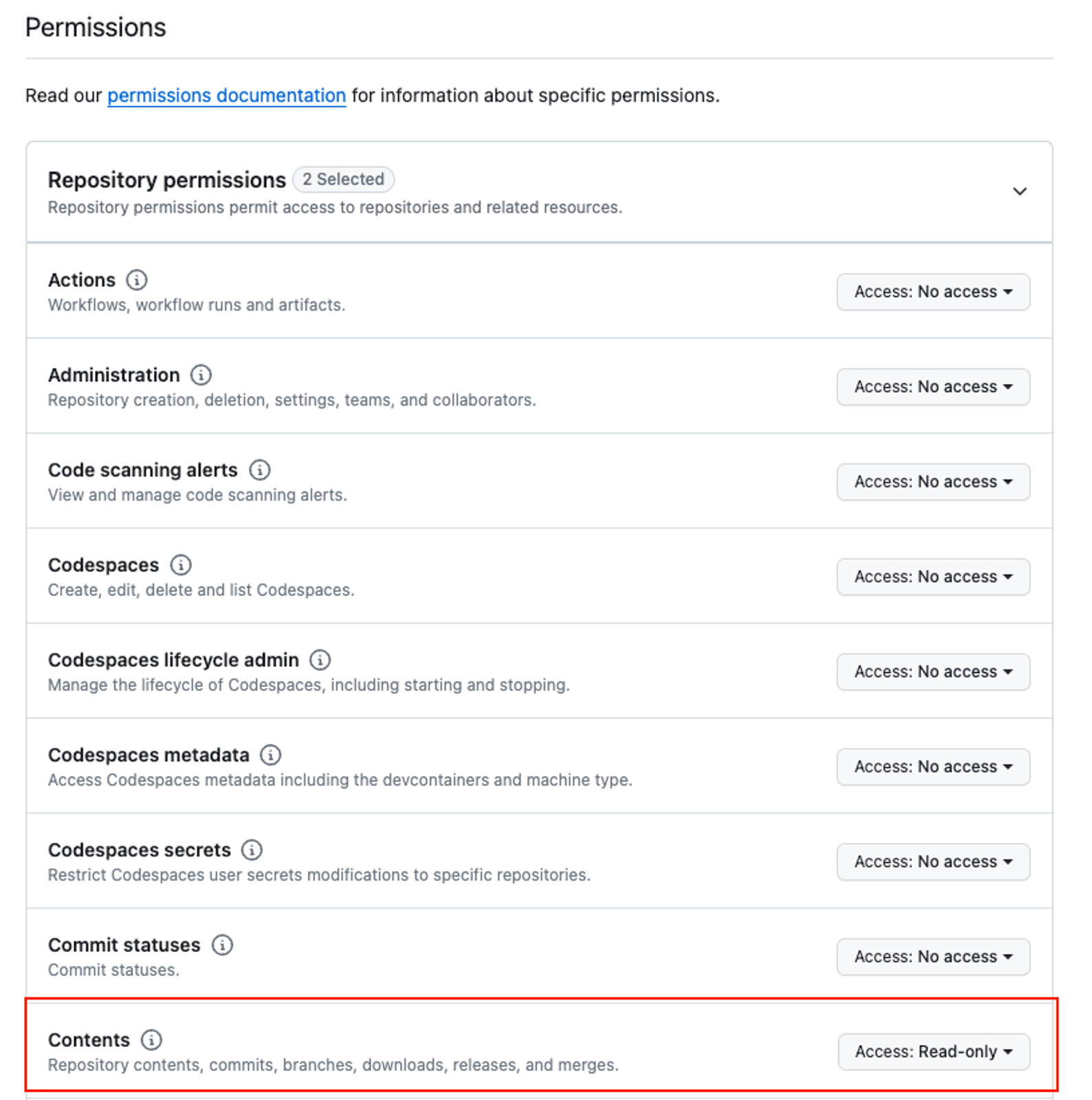
13. Click Generate token.
Note that you must save the token after you generate it, as there will be no way to access it after it has been created and shown to you.
We are now ready to take our access token and make some authenticated requests!
NOTE: Not all GitHub endpoints can be accessed using fine-grained personal access tokens. Please refer to GitHub’s documentation about which Endpoints are available for fine-grained personal access tokens.
Fetching repositories from the GitHub API in Python
Let’s go through two example requests we can make to GitHub’s repositories endpoint, one without authentication, and one using our new personal access token.
Making an unauthenticated request
Many organizations on GitHub have a public list of repositories that we can fetch information about without authenticating our request.
In this example, let’s see what repositories the Netflix organization stores on GitHub.
First we’ll import the necessary requests package and construct the URL that we want to make a request to (see GitHub’s list organization repositories documentation for more information).
After that, we can use our requests library to make a GET request to the URL we constructed to fetch information about Netflix’s repositories.
Making an authenticated request
This time, let’s make a request to the individual repository that we set our personal access token to have access to.
Similarly to before, we’ll first construct the URL that we want to make the request to, and this time we’ll also set up the headers for our request, which will contain our personal access token in the “Authorization” key.
After this, we can pass in the headers to our request and make an authenticated request to the GitHub API.
We’ve now successfully made an authenticated request to the GitHub API and retrieved (hopefully) useful information.
Final thoughts
The process of building to the GitHub API may seem manageable, but your clients likely want you to provide product integrations with countless other tools.
To meet customer demand for integrations at scale and without straining your engineering resources, you can use Merge, the leading unified API solution.
Merge offers a single API that lets you provide hundreds of integrations to your product, whether that’s CRM, ticketing, accounting, and so on.
Merge also provides Integration Observability tooling to help your customer-facing employees manage your integrations and maintenance support on behalf of your engineers—all but ensuring that you can offer reliable and performant integrations.
You can learn more about Merge by scheduling a demo with one of our integration experts.