Table of contents
How to get employees from any HRIS with Merge

If you're building internal systems and applications like training programs, payment processing systems, and performance-tracking apps, you'll often need to integrate with multiple human resources information systems (HRISs) to fetch, update, and sync employee data.
Most modern HRISs offer public APIs to allow developers to integrate with them. However, these APIs are not uniform across platforms, which makes integrating with multiple HRISs complex and hard to maintain. A Unified API such as Merge can reduce the complexity of your application and save time and costs by letting you get employee data from multiple HRISs using a single API.
This article compares getting employee data using several public HRIS APIs with a Unified API like Merge. It explains why a Unified API is more time- and cost-effective and allows you to build a simpler, more secure application. It also covers cases where you need to stick to the vendor's public API and shows you what it's like to use Merge to integrate with a popular HRIS like BambooHR.
Related: What, exactly, is HR integration?
Using Public APIs to Get Employee Data
Your first option for getting employee data from multiple HRISs would be to use the vendors' public APIs. The challenge is that these APIs vary in many core aspects like protocols, authentication methods, object schema, data format, and endpoints.
Let's consider how the APIs of two of the most commonly-used, cloud-based, HRIS solutions, Workday and BambooHR, differ.
Workday has a SOAP API architecture that uses XML to exchange messages. To get employee data using the Workday API, you need to:
- authenticate requests by creating an integrated system user (ISU) and giving it the required permissions;
- send requests and receive responses in XML format;
- send a POST request to the Get_Workers_Request endpoint; and
- handle responses according to their employee object schema.
On the other hand, BambooHR has a RESTful API, which is the most common standard for modern APIs. To get employee data using BambooHR API, you need to:
- use HTTP requests;
- authenticate them using API Keys;
- exchange data in JSON format;
- send a GET request to the https://api.bamboohr.com/api/gateway.php/{companyDomain}/v1/employees/{id} endpoint; and
- handle responses according to their specified object schema.
Integrating with only these two HRIS APIs will require extra time and, thus, costs. This process adds to the complexity of your solution.
First, you will need to spend time learning how to interact with each API and go through different sets of documentation created in different styles. The integrations themselves require you to use different authentication methods, perform data normalization and create custom SQL queries to process different data models, and use different endpoints and HTTP requests to pull the same type of data.
Even after building the integration, you will need to maintain it. You will have to keep up with two sets of API updates, revisit the API documentation with each update, update your code as regularly as needed, and ensure that you comply with any changes in the vendors' terms of service.
There are cases where there's no way around the complexity of integrating with multiple APIs. For instance, you might be building integrations with systems that don't serve the same purpose, such as integrating an internal system with HRIS and accounting cloud platforms.
However, if you need to integrate with multiple systems for the same purpose, a Unified API solution can be cost-effective and offer time savings.
Using Merge to Access HRIS Data
Let's see what it would look like to use Merge's Unified API to get employee data from HRISs like Workday or Bamboo HR.
Merge provides a single programming interface to fetch, update, and sync data from multiple APIs. Its Unified API uses a single authentication method, standardized data schemes, and a single set of unified endpoints to send requests to all integrations across all HRIS platforms.
To start, Merge saves you the hassle of learning how to interact with several APIs and going through different sets of documentation. You only need to use one set of well-written and beautifully designed documentation and you create links with the relevant HRIS vendors using a single web-based UI.
Compared to integrating with multiple vendor APIs, a Unified API also shortens development time and reduces the complexity and code size of your integration. You only need to use one authentication method to send all API requests. It lets you use a unified schema for common data objects such as employee, company, and location. ou can also use object relationships and nesting for complex schemas.
You don't even have to worry about data normalization since you will be using a standardized data model for all integrations. Using one authentication method and standard endpoints across all integrations not only reduces complexity but also improves security.
Unified APIs can also improve the performance of your integration. It normalizes and syncs data at a frequency of your choice and then stores data to make it accessible through the API's standard endpoints.
Maintaining your integrations is also less time-consuming and costly. If a vendor updates their API, Merge updates its integration with them. Your integration with Merge remains unaffected. This means you don't have to frequently dive into the documentation of multiple APIs, update your integration to comply with each API update, explore new features, or remove deprecated and revoked functions.
Lastly, unlike most HRIS vendors, Merge provides SDKs for many programming languages. Merge's HRIS SDKs are available in many popular programming languages, including Python, Ruby, Go, Java, Elixir, and C#.
Related: What is an employee API?
Setting Up Merge with BambooHR
What is it like to use Merge to integrate with an HRIS such as BambooHR?The steps for setting up the integration and fetching employee data are the same no matter which HRIS you integrate with:
- Create a link between Merge and the HRIS.
- Get authentication tokens.
- Fetch employee data.
For illustrative purposes, we'll use BambooHR.
You'll need to have a Merge account and gain access to a BambooHR Sandbox to follow along. You can sign up for free accounts with Merge and BambooHR. The BambooHR account comes with demo data that you can use for testing.
The code sample that follows is written in Node. To run it, you need to have Node installed along with npm.
Create a Link Between Merge and the HRIS
Navigate to your Merge dashboard in your browser. If your account is newly created, you will see a Get Started page to help you create your first integration. Scroll down and click Open Merge Link. If you don't see the Get Started page in your dashboard, you can create a new link from the Test Linked Accounts page.
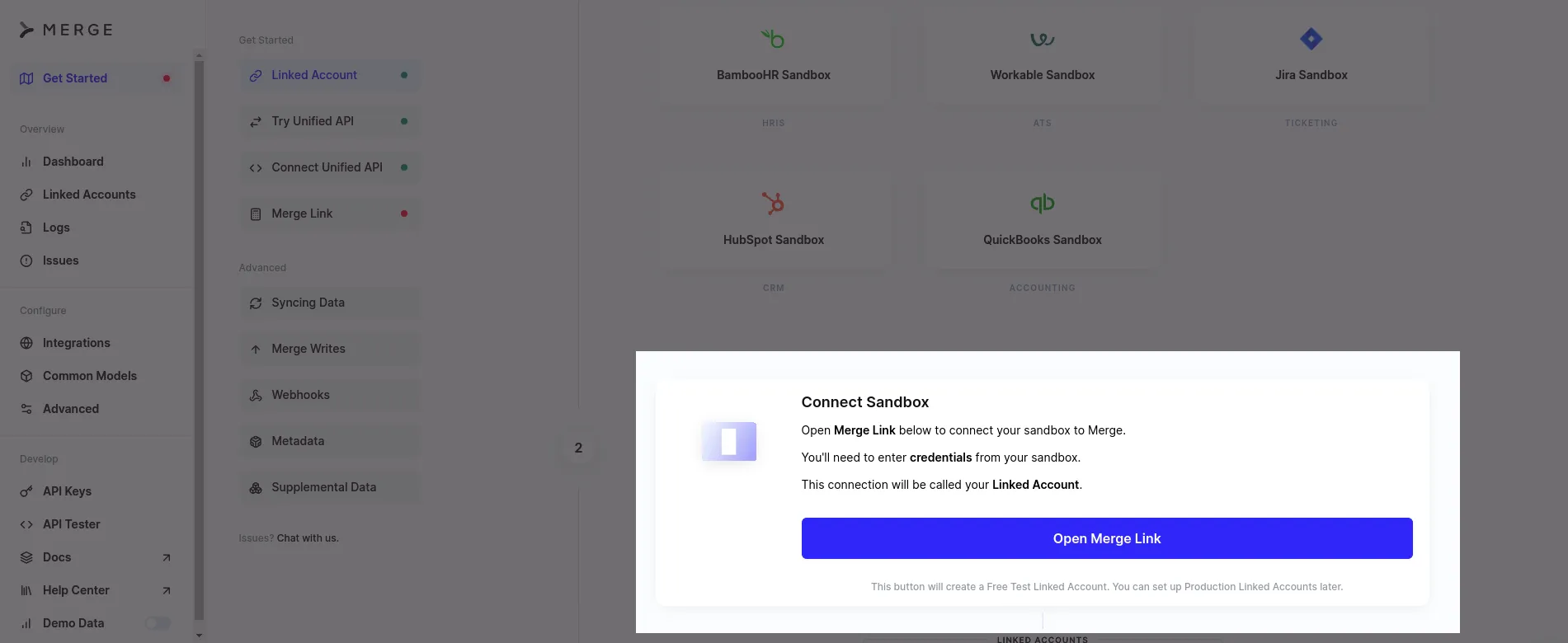
In the pop-up that shows all the integration options available, select Human Resources Information System and then BambooHR:

Merge will start creating the link and syncing your data in the background. The link will take a few minutes or more depending on the size of your data. Once it is done, you will get a message that confirms the link is created:
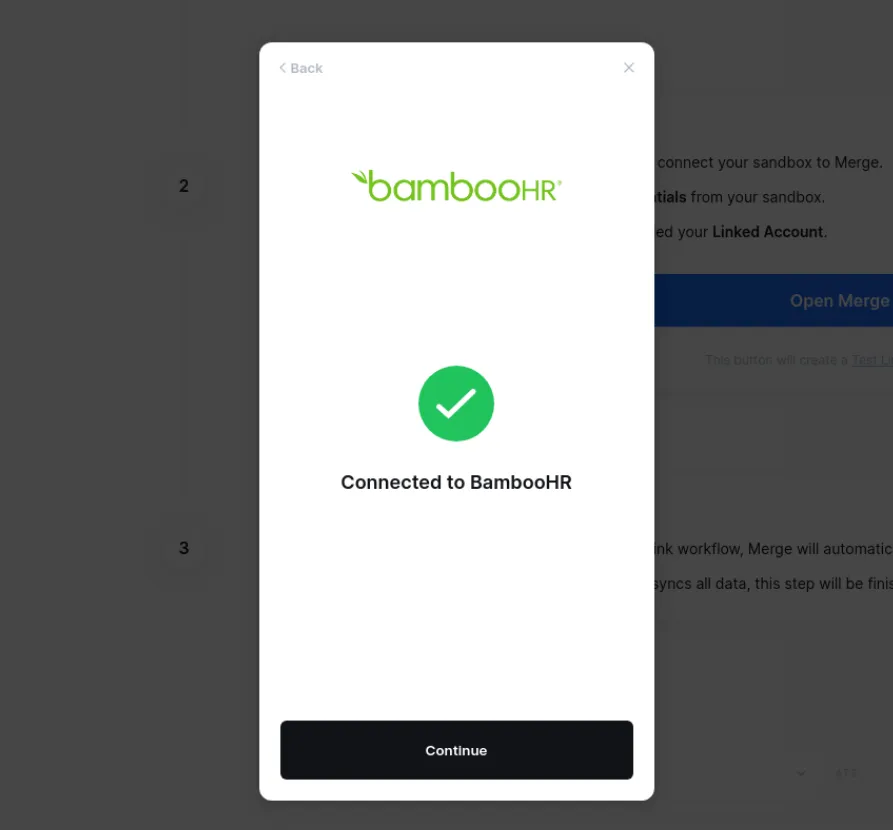
Get Authentication Tokens
To authenticate every Merge API request, you need two keys: an access key and an account token.
To get the access key, open the API Keys page from the left-side menu and copy the default key listed under the Test Access Keys.
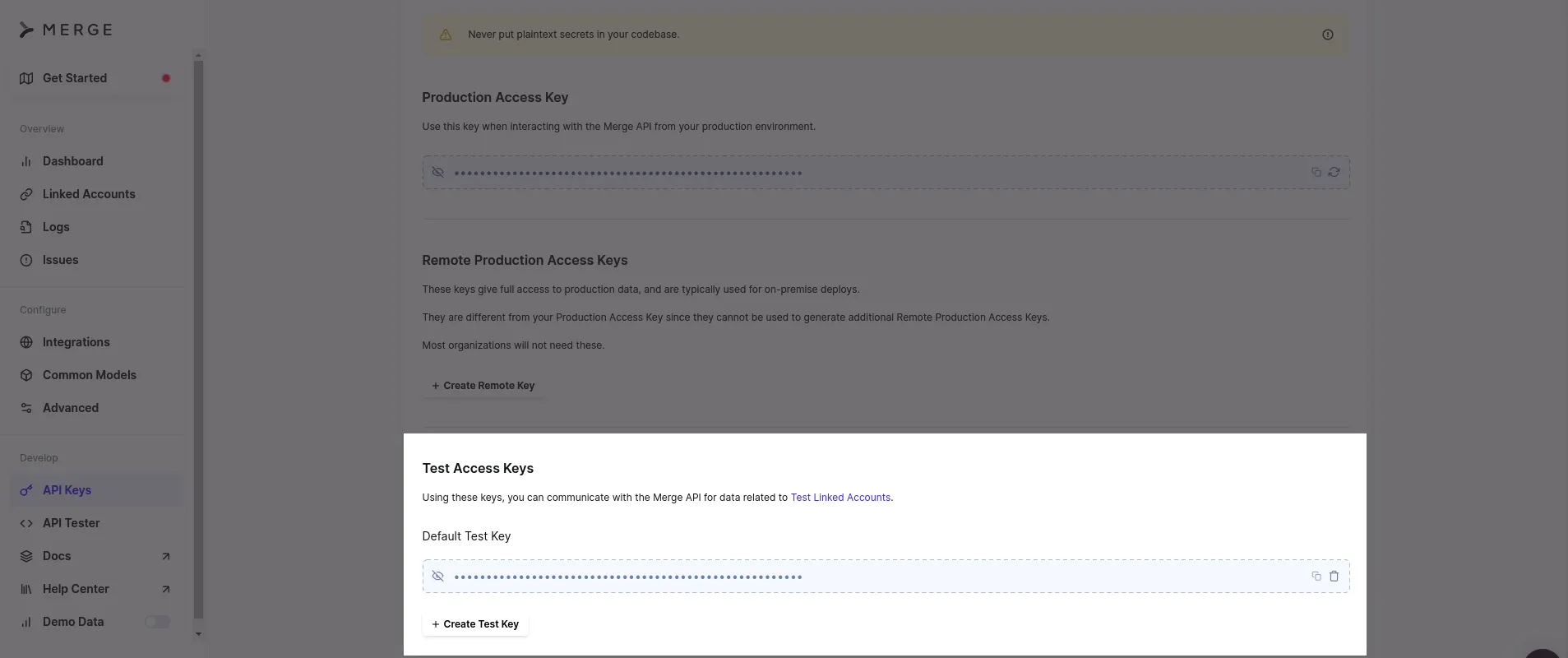
The account token is specific to every Merge link. To get the account token for your BambooHR integration, open the Linked Accounts page from the left-side menu. This page shows both the production and testing linked accounts. Click Test Linked Accounts on the top menu and then click on the BambooHR link that you created in the previous step:

From the BambooHR link page, copy the account token:
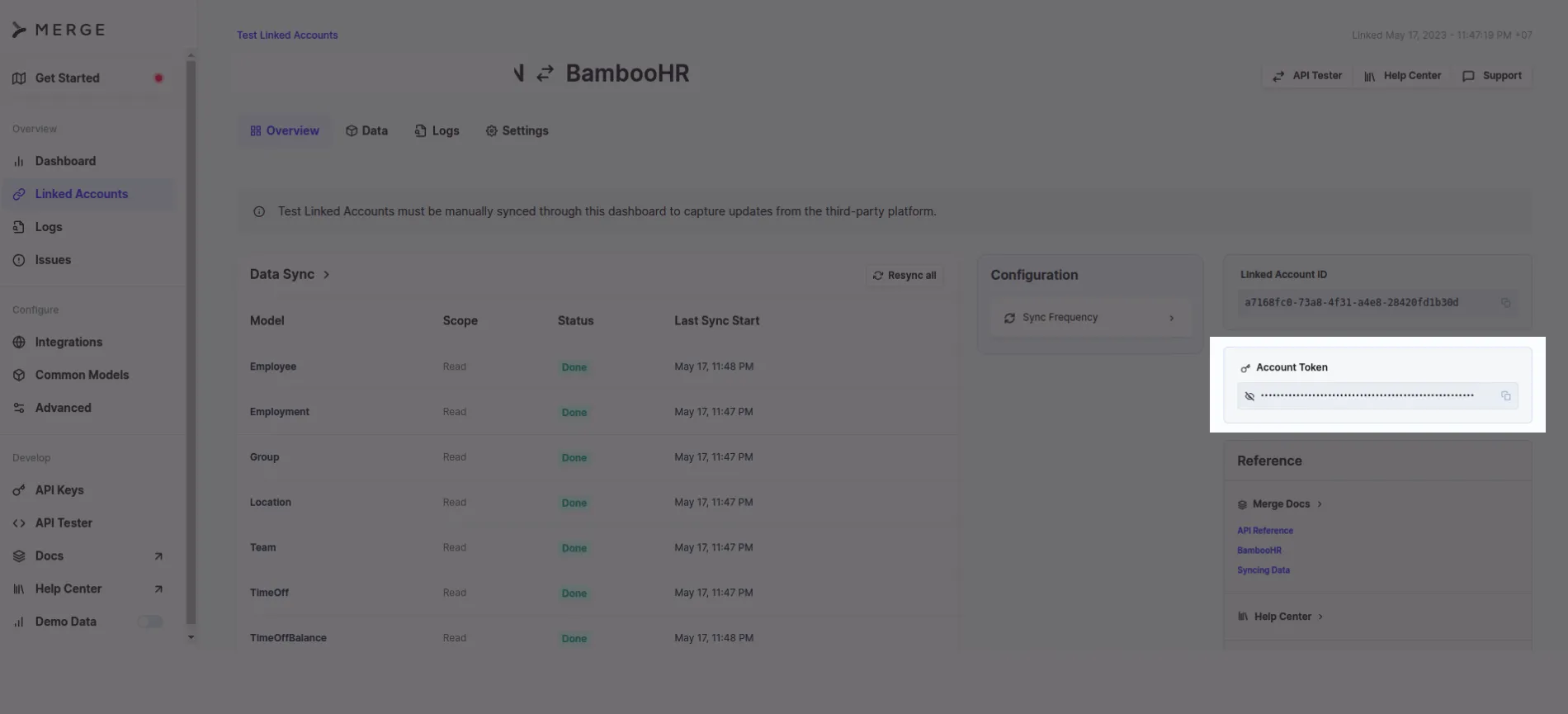
Fetch Employee Data
To send API requests to Merge endpoints, you will use the axios package.
Navigate to your project folder in your terminal, and run the following command:
In the same folder, create a file named getEmployees.js, open it, and paste in this code:
In this code, the axios library is imported at the first line, followed by declaring the <code class="blog_inline-code">API_ACCESS_KEY</code> and <code class="blog_inline-code">BAMBOOHR_TOKEN</code> variables. Then, the config variable is declared to hold the authorization headers. The axios.get function sends a GET request to the https://api.merge.dev/api/hris/v1/employees endpoint, which is the endpoint that can be used to get employee data from all HRIS integrations.
Before running the code, replace API ACCESS KEY HERE with your access key and BAMBOOHR TOKEN HERE with your BambooHR access token to authenticate requests.
To run the code, go to the terminal window and run the following command:
After the request execution, the response will look like this:
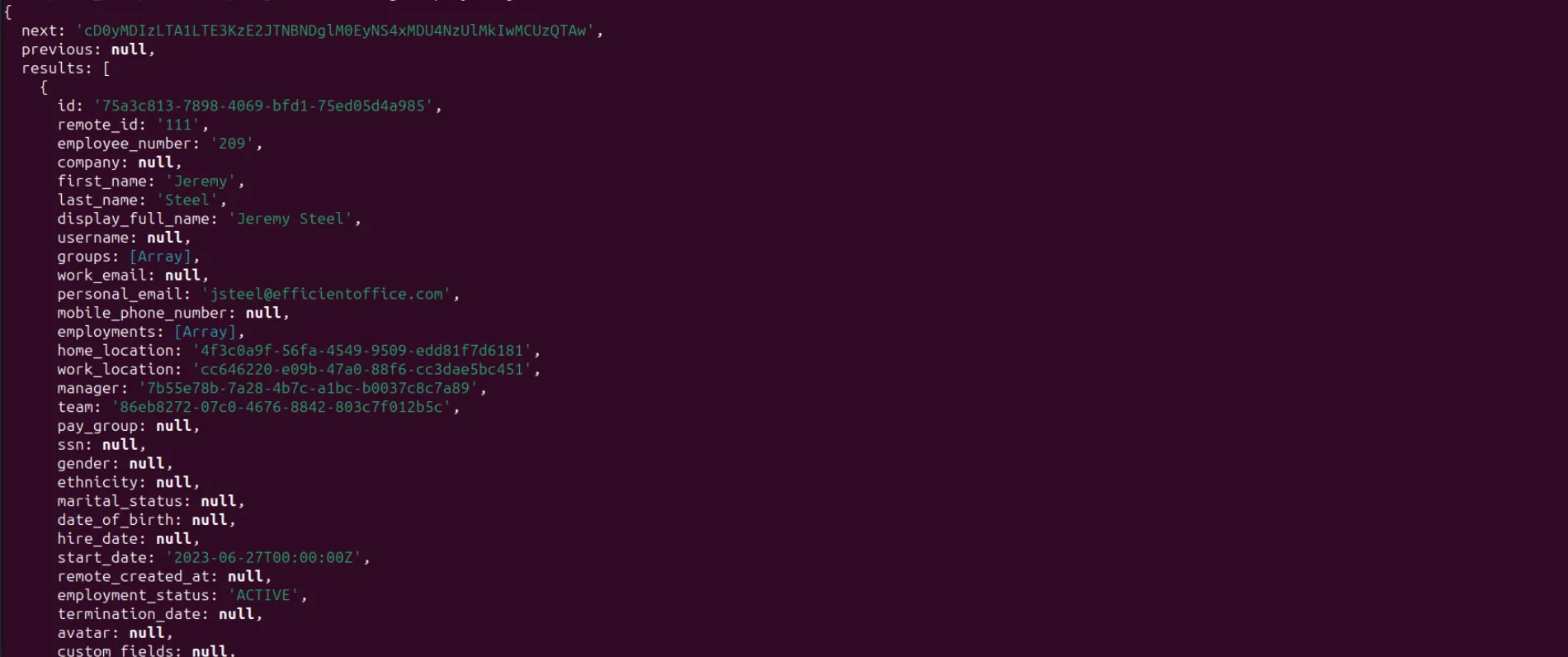
The response data object includes pagination parameters and an array of the employees' data.
This employee object is unified across all the HRIS integrations supported by Merge, which means you can use these same steps to integrate your application with all the other HRIS vendors that Merge integrates with.
Conclusion
Integrating with multiple HRIS APIs to get employee data is complex, time-consuming, and costly.
Though unavoidable in some cases—like when you're integrating with systems that don't serve the same purpose in your app or where the HRIS you need to integrate with is not supported by any Unified API—, using a unified API like Merge is more time- and cost-effective and results in a simpler, more secure solution.
Merge provides a single programming interface to get data from more than fifty HRIS APIs, including Workday, BambooHR, ADP, Gusto, UKG Pro, and Hibob. You also get access to hundreds of integrations with accounting, ticketing, marketing automation, and file-storage platforms.
As you saw, getting employee data from a popular HRIS such as BambooHR requires only three straightforward steps. Merge also offers webhooks that you can use to be notified of changes in data in HRIS platforms and trigger automated workflows.
Sign up for a free Merge account to see how Merge helps reduce the time you spend on creating and maintaining integrations.