How to fetch employees from Rippling using Python
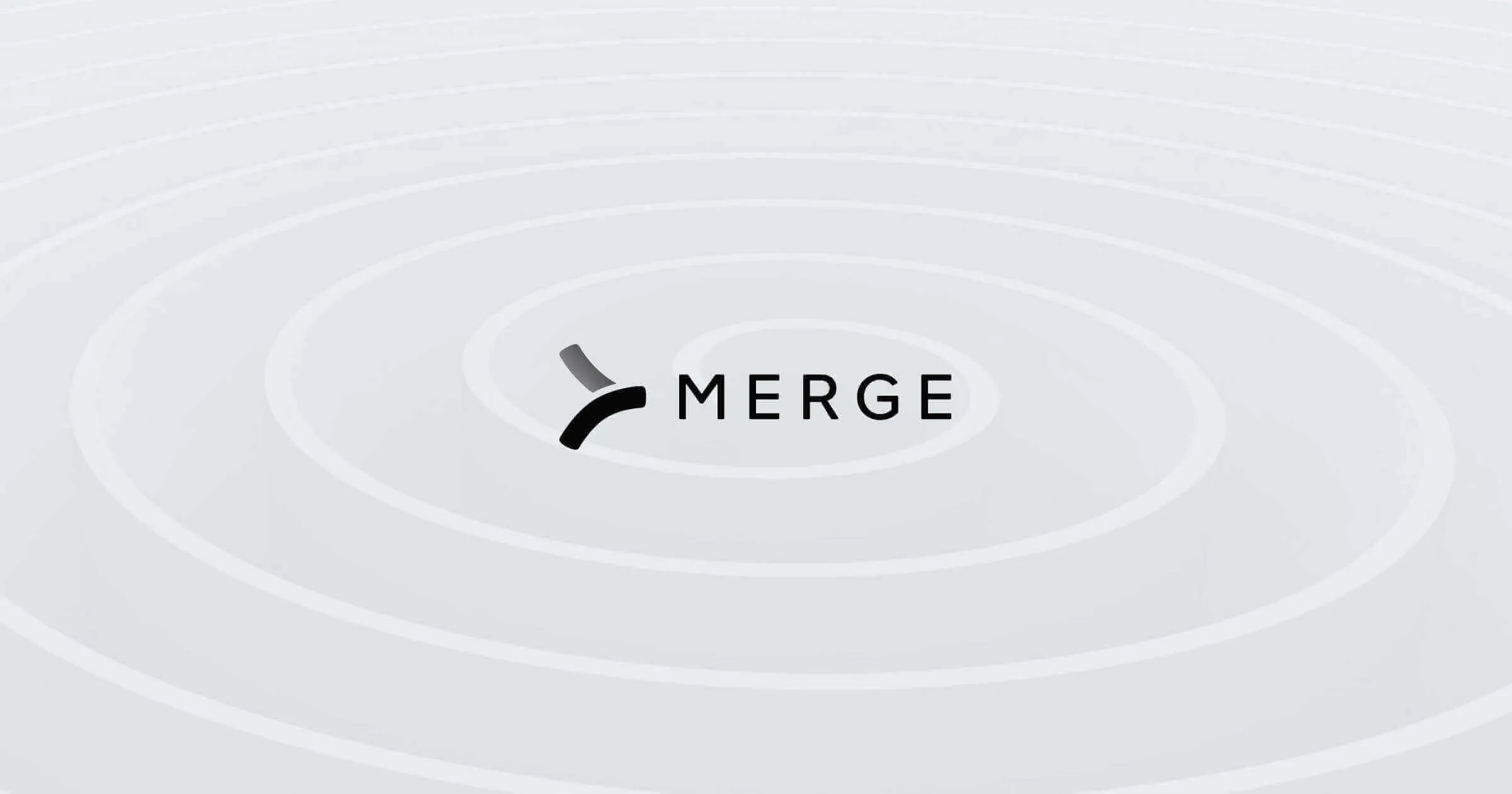
Rippling stands as a potent HRIS that helps businesses manage payroll, benefits, employee onboarding, and more. Given all its use cases, it likely stores rich data on employees.
We'll help you retrieve employee data from Rippling's API (using Python) by breaking down every step you need to follow.
Authentication configuration
To pull employee data from Rippling API, you first need to authenticate your application. Rippling uses the OAuth 2.0 protocol for authentication and authorization.
In the header of each HTTP request, include an authorization token in the following format: <code class="blog_inline-code">Authorization: Bearer {API-KEY}</code>. This <code class="blog_inline-code">{API-KEY}</code> is your unique application key provided by Rippling. Make sure to replace <code class="blog_inline-code">{API-KEY}</code> with your actual API key.
It's important to note that all API requests must be made over HTTPS. Calls made over plain HTTP will fail. Also, you must authenticate for all API requests. Also, remember to keep your API key secure. Do not share your secret API key in publicly accessible areas such GitHub, client-side code, and so forth.
Related: Recruiting integrations worth building
How to get employees
The Python code below fetches employees from Rippling API using <code class="blog_inline-code">requests</code> library. It uses a generator to yield employee data as it is fetched from the API. The function <code class="blog_inline-code">fetch_employees</code> takes an API key and a limit as arguments and fetches employee data in a paginated manner using an offset.
Moreover, the code fetches data from the API endpoint using a GET request. It includes an authorization header in the format <code class="blog_inline-code">Authorization: Bearer {API-KEY}`</code>. The function fetches data in pages of size <code class="blog_inline-code">limit</code> and increments the offset by <code class="blog_inline-code">limit</code> after each request to fetch the next page. It stops fetching when it encounters an empty page.
Here'e an example of a response from the request.
Related: What you need to know to perform API integration in Python
Final thoughts
Your clients likely use a wide range of HRIS platforms—not just Rippling.
To cater to the varied landscape of HRIS tools, you can build to Merge's HRIS Unified API. Once you’ve built to it, you can offer dozens of ticketing integrations to clients, whether that’s Workday, Namely, UKG, etc.
You can learn more about Merge by scheduling a demo with one of our integration experts.