Table of contents
How to GET folders from the Google Drive API in python
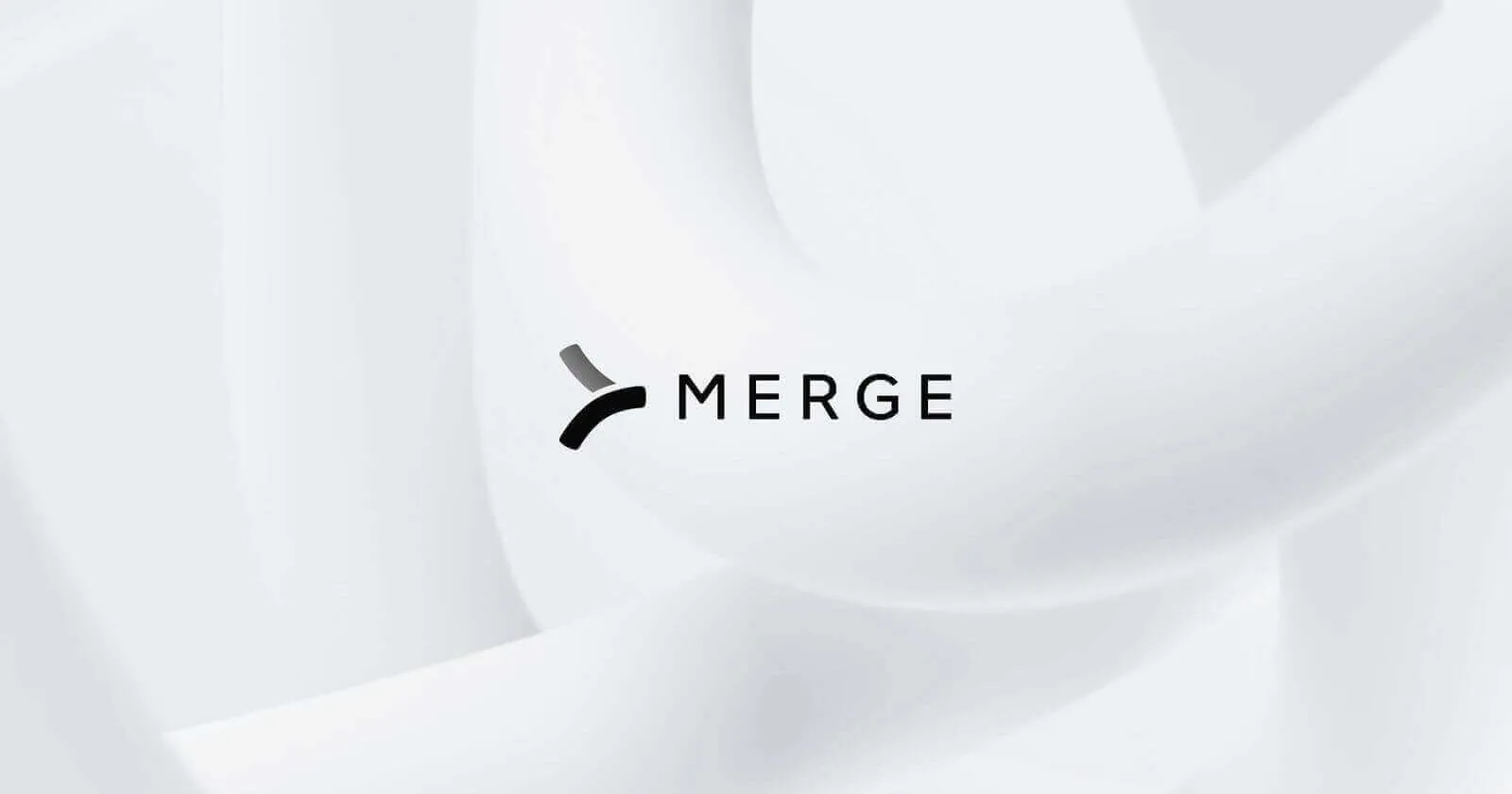
While a cloud storage solution like Google Drive offers many benefits on its own, such as multidevice access, backup, and easy sharing, it's value gets extended when its files can be accessed by your other internal applications or your product.
To help you do just that, we'll walk through every step you should take to get all the folders in the Google Drive API using Python.
{{this-blog-only-cta}}
Prerequisites and project setup
To get started, you can install the following on your machine:
You also need a Google Cloud project where your app will live. Create a new one or reuse an old one. Enter your preferred name and organization settings and click Create:

Finally, you will need a Google account to use as the test account for this tutorial. This account will be used with Google Drive to store and retrieve folder names.
You need to create three Google Drive folders in this Google account to test your app. Sign in to Drive with the account and add the folders:
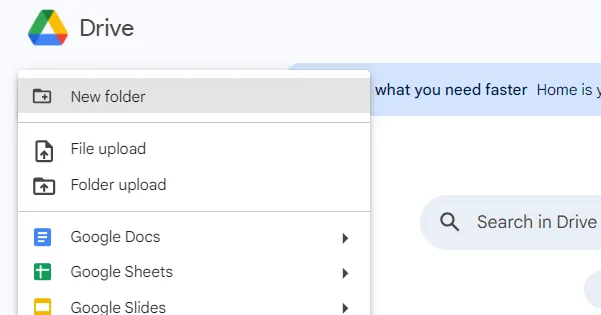
You will need to use this same account later in the tutorial when you'll be adding a test account to the Google Cloud project.
Configure your app to access the Google Drive API
You need to configure your app to access the Drive API following the setup instructions.
To enable the Google Drive API in your project, navigate to the Google Cloud Console, confirm the project, and then click on Enable:

Next, configure the OAuth consent screen, which is the screen users will see when they request permission to access their folders. Navigate to the OAuth consent screen console. Select the user type—use the External user type for this tutorial:
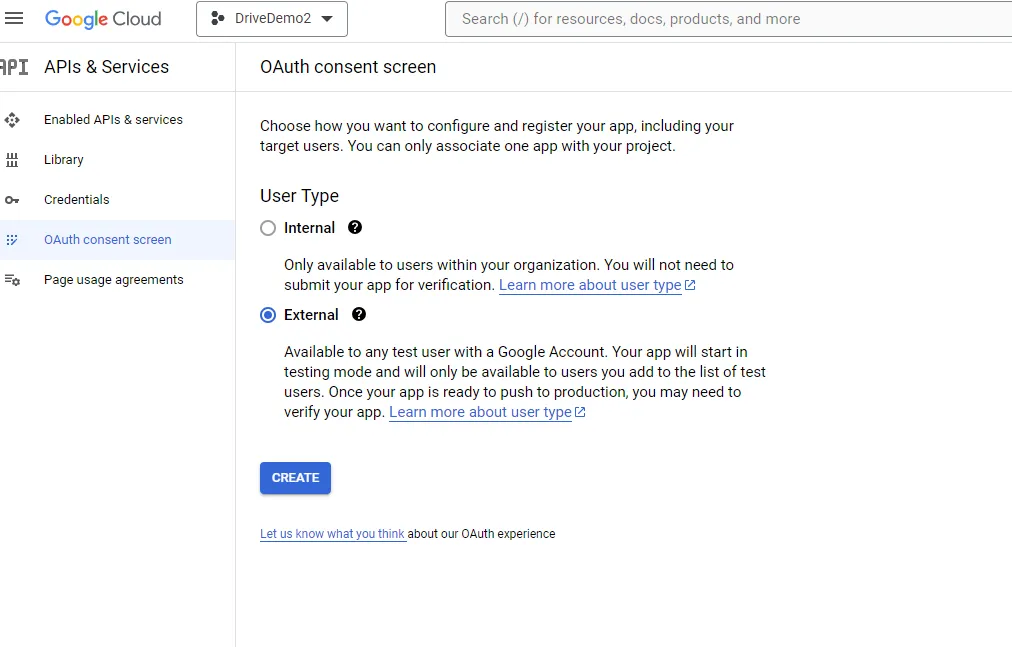
You are taken to the App Information page next. Enter your app information, which is the information that will be presented to the end user in the consent screen. Click Save and Continue:

You can skip Scopes for this tutorial, so click Save and Continue.
Next, you'll add test users; these are the accounts that can use the app before it is published. For this tutorial, add the Google account in which you created the Drive folders described in the Prerequisites section:

Finally, you need to create credentials that authenticate your Python application. These credentials help Google understand that the request to retrieve Drive information is coming from an authentic application.
Navigate to the credentials console by clicking on Credentials on the left of your screen. You'll be creating credentials of the OAuth Client ID type to be able to access user data:
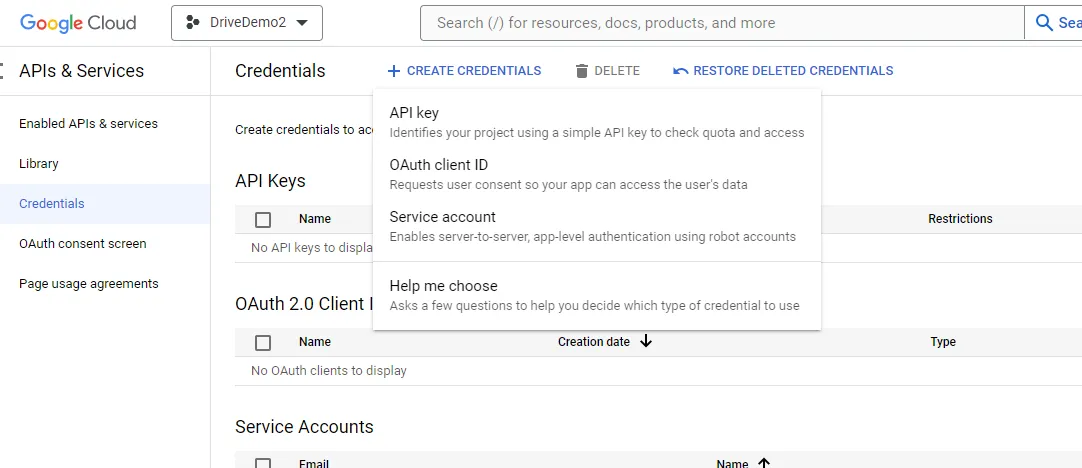
In the next screen, choose Desktop app as the application type since you will be running the app from your desktop in this tutorial:
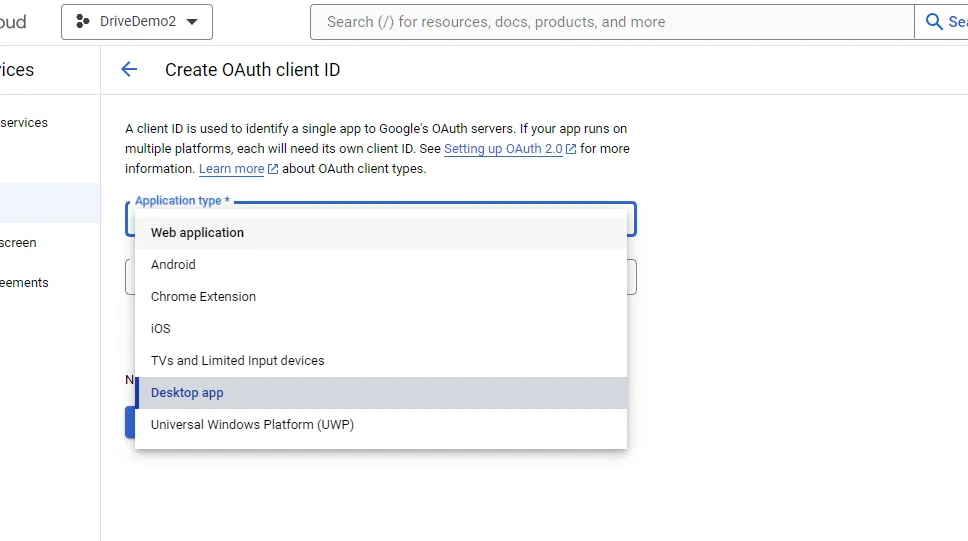
In the next screen, you have the option to download a JSON file with the app's credentials. Download it to the folder in which you will be developing your app:

Get Folders
In this section, you'll write a function that calls the Google Drive API using the Google SDK library.
Create a Python file named drivedemo.py in a folder named DriveDemo and add the following content to it:
Before you can run this script, you need to install the Google API Client and Flask. To do that, run the following command:
Now run the following command to test the script: <code class="blog_inline-code">python drivedemo.py</code>
The first time you run drivedemo.py, Google's OAuth consent screen will come up, requesting permissions to the account's drive. Remember that you need to be logged in to the Google account you added as a test user account when you click on Continue to allow access:
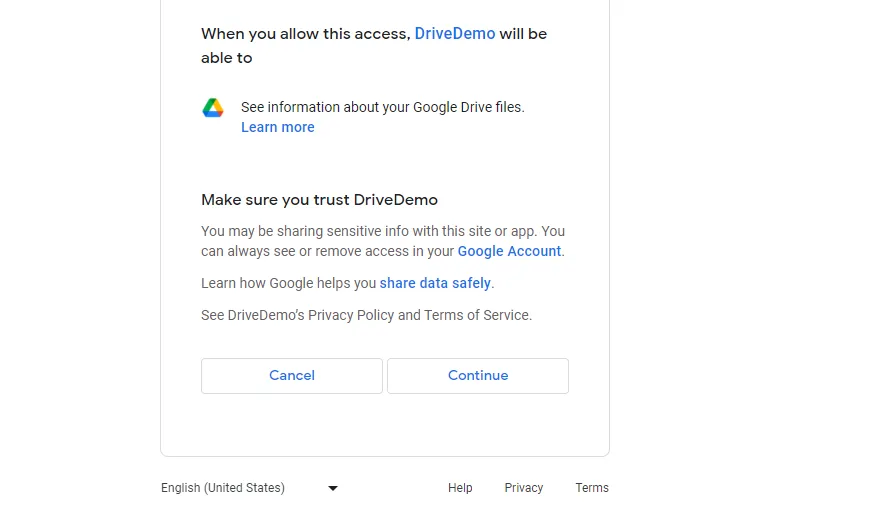
The previous code snippet saves credentials to a local file called token.json to be reused for future runs. This logic is implemented in the getCredentials() method.
It authenticates with the <code class="blog_ inline code">credentials.json</code>
previously downloaded and the user with <code class="blog_ inline code">token.json</code>.
Central to the app is the following snippet, which runs in a while loop:
It uses the files.list method of the REST API to pass in the query string `mimeType = 'application/vnd.google-apps.folder` to filter down to the folders. It extracts the `id` and `name` file fields and saves the `page_token` for the next page of results.
Repack your app into Flask
In this section, you will repackage your app into a web application so it can be accessed from the browser.
In the same folder, DriveDemo, create another file named app.py with the following content:
Your app is now running locally. You can access it by navigating to http://17.0.0.1:5000 on your browser, where you'll see the app's home page.

Navigate to http://127.0.0.1:5000/folders to see and access the folders:
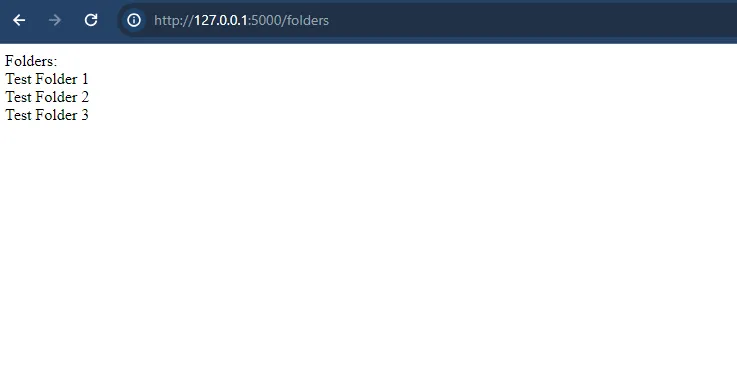
Congrats! You now have an app that integrates with Google Drive.
You can find the code for this tutorial in this GitHub repository.
Further steps could involve publicly exposing your app on web servers such as Apache and Nginx.
Conclusion
As you can tell, retrieving files from Google Drive via API requests can be technically complex and time consuming to set up (and maintain!). These issues only get exacerbated when you consider the other file storage tools your clients want to integrate with your product, such as Dropbox or Box.
To help you integrate with any of your clients' file storage solutions so that your product can access their folders (among other resources), you can simply build to Merge's File Storage Unified API.
You can learn more about Merge and its Unified API by scheduling a demo with one of our integration experts.