How to get comments from Jira using JavaScript
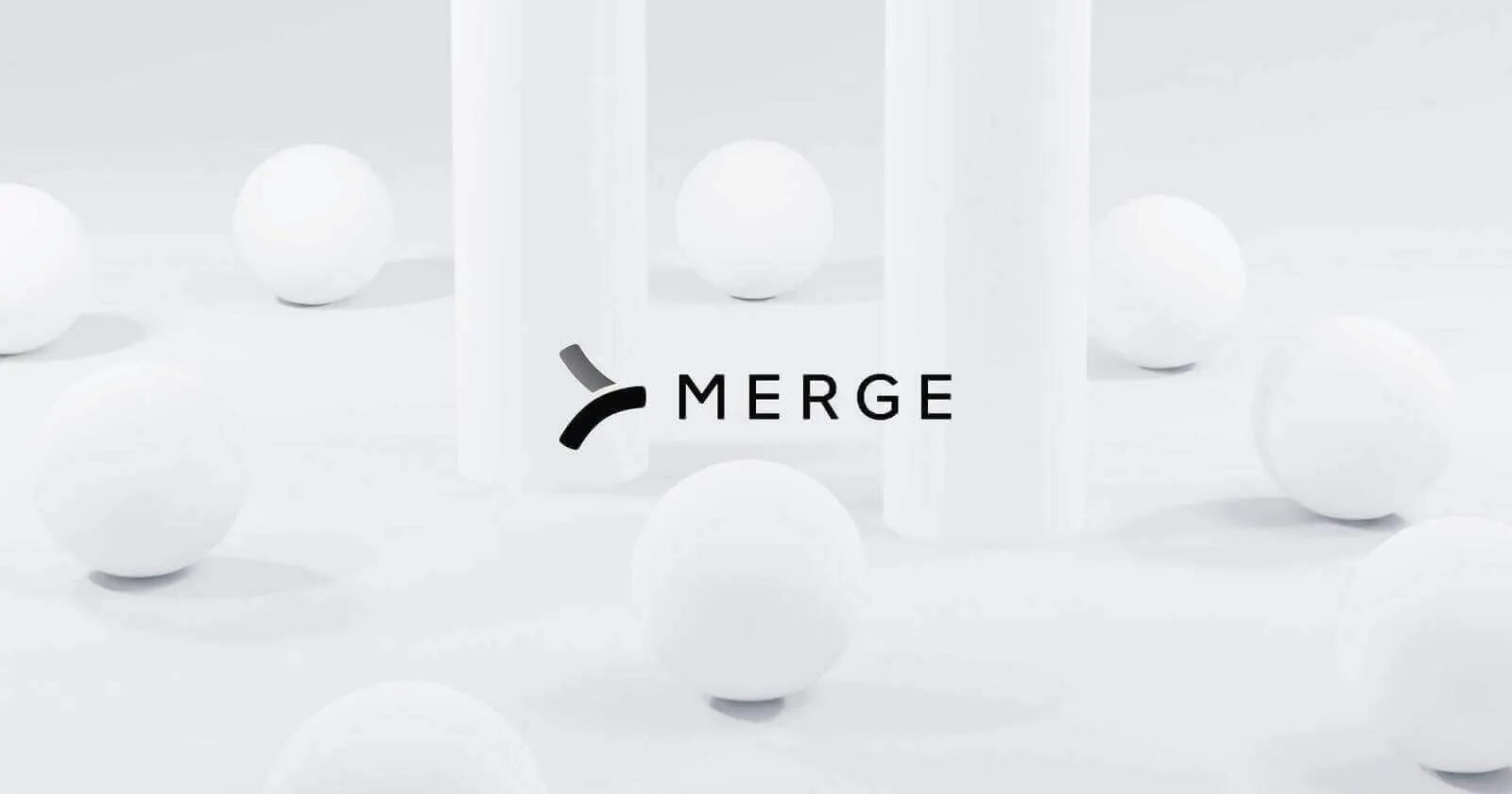
Jira, a potent ticket management tool, provides a powerful API that gives developers the means to extract key data. And while there are a variety of different types of data you can fetch, comments in Jira might be at the top of your list.
Fetching comments for your product or the other systems you’re using internally allows you to elevate collaboration, streamline task management, and ultimately save employees’ time.
We’ll help you get comments from Jira via JavaScript by covering how you can set up authentication for the Jira API, and then make get requests to pull comments.
Related: What you need to do to retrieve attachments from Jira using JavaScript
Authenticating with Jira
To interact with the Jira API, you'll need to authenticate your requests.
You can do this by including an `Authorization` header with your HTTP requests.
The value of this header should be `Basic` followed by a base64-encoded string, which is a combination of your email address and API key.
The format of this header should look like this: <code class='blog_inline-code'>Authorization: Basic {Email Address}:{API-KEY}</code>.
To obtain your API key, you can visit the Atlassian account settings. The email address should be the one associated with your Atlassian account.
For example, if your email is `johndoe@example.com` and your API key is `1234567890abcdef`, the base64-encoded string would be the result of base64-encoding `johndoe@example.com:1234567890abcdef`. This encoded string is what you should use in your `Authorization` header.
It’s worth noting that this is a basic form of HTTP authentication and may not be suitable for all use cases. For higher security, consider using OAuth.
Related: How to fetch comments from the Jira API using Python
Pulling comments from Jira
The code below leverages the <code class='blog_inline-code'>axios</code> library to send GET requests to the Jira API. The first API endpoint is hit to get the list of issues, using pagination to get all issues. For each issue, the ticket_id is extracted and used to hit the second API endpoint to get the comments for that issue. The comments are then logged to the console.
It’s also worth noting that the pagination logic for the comments is kept simple for this demonstration, but it can be expanded to handle more complex scenarios.
Before running this code, replace <code class='blog_inline-code'>Email Address</code> and <code class='blog_inline-code'>API-KEY</code> with your actual email address and API key, and replace <code class='blog_inline-code'>{DOMAIN}</code> with your actual domain.
An example of an individual item returned by this API endpoint is:
Related: A guide to getting projects from Jira via JavaScript
Tips for testing your Jira integration
Let's say you and your team haven't spotted any glitches with the Jira integration. That's great, but keep in mind, it might still encounter snags once it's live in production. These snags can be anything from network latency issues to unexpected user input to conflicts with other software.
Here's what you can do to get ahead of these potential problems:
- Understand the API specifications: This is key because knowing the nitty-gritty of the API helps you predict where problems might crop up. With this in mind, dive into the documentation and get familiar with how it's supposed to work.
- Create a test plan: This is crucial as it guides your testing process. Think of it as your road map. For instance, if your plan includes testing how the integration handles large data sets or reacts to invalid user inputs, you're less likely to be caught off guard.
- Test under different conditions: This step is vital because it ensures your integration holds up under various scenarios. Try mixing it up with different environments or vendors—like testing on both Windows and Linux systems, for example.
- Check response status codes: This is a must-do. It's how you'll know if things are running smoothly or if something's gone haywire. Regularly monitoring these codes will give you real-time insights into the health of your integration.
- Collaboration and communication: Last but not least, keep in constant touch with your team; sharing insights and flagging issues early on can make a world of difference in managing the integration effectively.
Final thoughts
It's highly probable that your clients utilize a diverse range of ticketing platforms—not just Jira.
To cater to this varied landscape of ticketing tools, you can build to Merge's Ticketing Unified API. Once you’ve built to it, you can offer dozens of ticketing integrations to clients, whether that’s Zendesk, Jira, Asana, GitHub, etc.
You can learn more about Merge by scheduling a demo with one of our integration experts.