How to fetch conversations from Intercom using Python
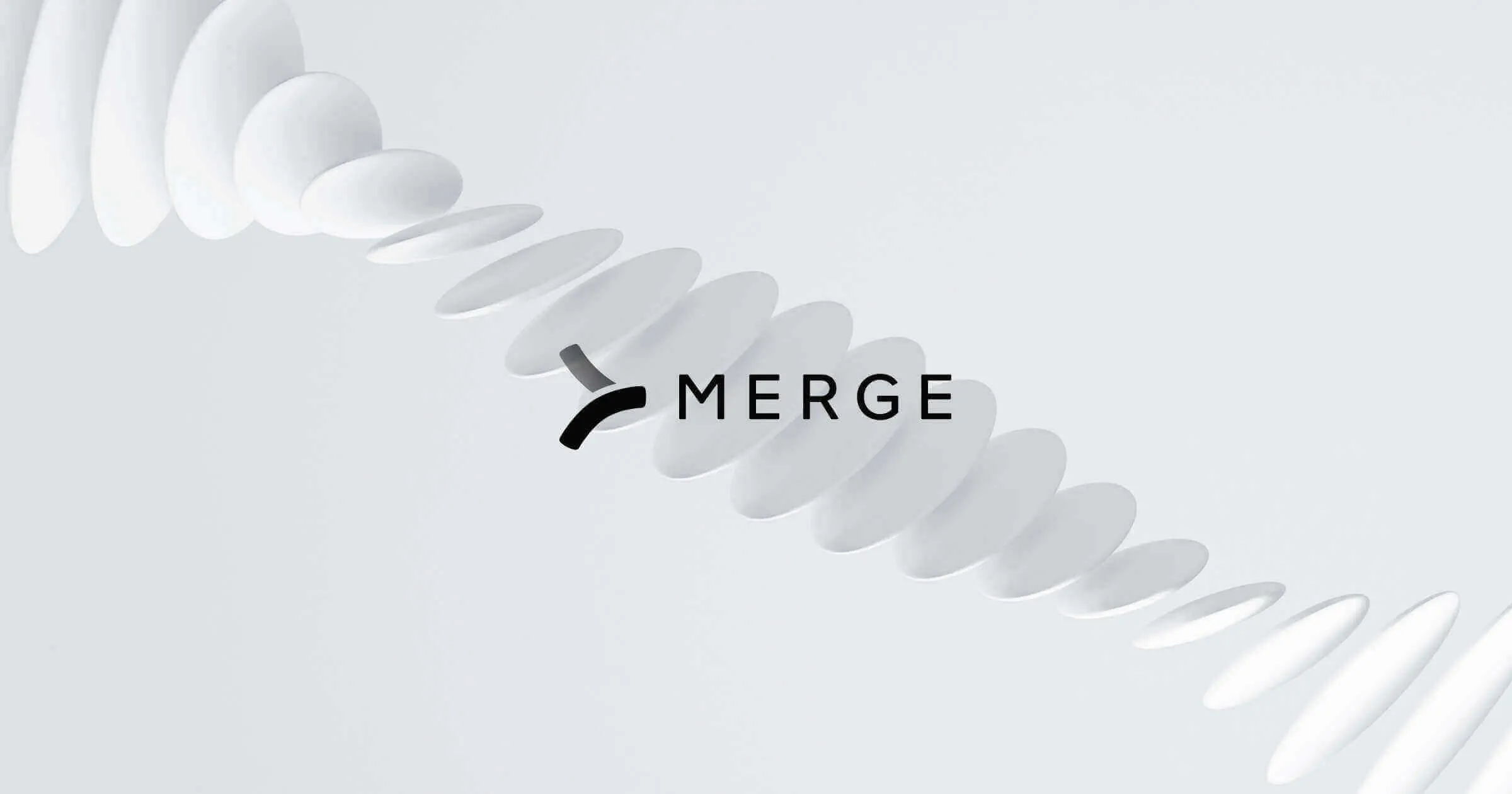
Editor's note: This article is part of a series on building third-party API integrations. Explore Merge if you’re looking to add 180+ integrations across HR, SCIM, payroll, ATS, CRM, accounting, ticketing, marketing automation, and file storage with one unified API.
Intercom is a powerful customer communication platform that equips organizations with an array of tools for effective customer engagement and interaction. It combines functionalities such as live chat, help desk support, and customer engagement, enabling businesses to deliver personalized customer service and foster enhanced relationships. Additionally, Intercom provides an Application Programming Interface (API) for businesses to access, integrate, and manipulate their Intercom data, encompassing conversations, users, and companies.
For developers aiming to extract Conversations from a client's Intercom system, they can do so to analyze customer dialogues, optimize customer service, and gain valuable insights into customer behaviors and preferences. The Intercom API serves as an integration point allowing developers to programmatically retrieve, manage, and manipulate these Conversations. This facilitates a more efficient, data-centric approach to improving customer experience and satisfaction.
Intercom API's authentication configuration
Intercom supports two forms of authentication -- OAuth and Basic Authentication. In this article, we will walk you through the Basic Auth method.
First, you need to find your API key. This token can be found in your Intercom settings under <code class="blog_inline-code">Access Token Management</code>.
Every request you make must include this access token in the <code class="blog_inline-code">Authorization</code> header field. The format will look like this: <code class="blog_inline-code">Authorization: Bearer YOUR_ACCESS_TOKEN</code>.
In addition to this, all requests to Intercom must include the header field <code class="blog_inline-code">Accept: application/json</code>. This specifies that the client wants the response data in JSON format. Make sure to include these header fields in all of your API requests to ensure successful communication with the Intercom API.
GET conversations from Intercom's API
Now let's get coding.
In this script below, we are making a GET request to Intercom's Conversations API endpoint. We are using the <code class="blog_inline-code">requests</code> library to send the HTTP request and the <code class="blog_inline-code">json</code> library to parse the JSON response.
The function <code class="blog_inline-code">get_conversations</code> sends a GET request to the provided URL and returns the parsed JSON response. The function <code class="blog_inline-code">extract_conversations</code> extracts the conversations from the response. We first call <code class="blog_inline-code">get_conversations</code> with the initial URL and extracts the conversations from the response.
Now, we start paginating through Intercom's response. The function <code class="blog_inline-code">get_next_page</code> checks if there is a next page URL in the response and returns it if there is one -- otherwise it returns <code class="blog_inline-code">None</code>. Then we enter a loop where it keeps calling <code class="blog_inline-code">get_conversations</code> with the next page URL and extracting the conversations until there are no more pages. Finally, we print all the conversation!
Congrats! Now you should see the list of Conversations from Intercom as the output.
Conclusion
Wasn't that fun? Now you can keep building!
Unfortunately, Intercom is probably only one of many integrations in the help desk / ticketing space that your team must integrate with.
This is where a Unified API, such as Merge, comes into play. At Merge, we’ve built an API that lets you easily integrate once to offer 30+ Ticketing, Project Management and Helpdesk integrations. Our Unified API fully normalizes authentication, pagination, rate limits and response bodies.
Learn more about Merge by scheduling a demo!