Table of contents
6 real-world examples of SDKs
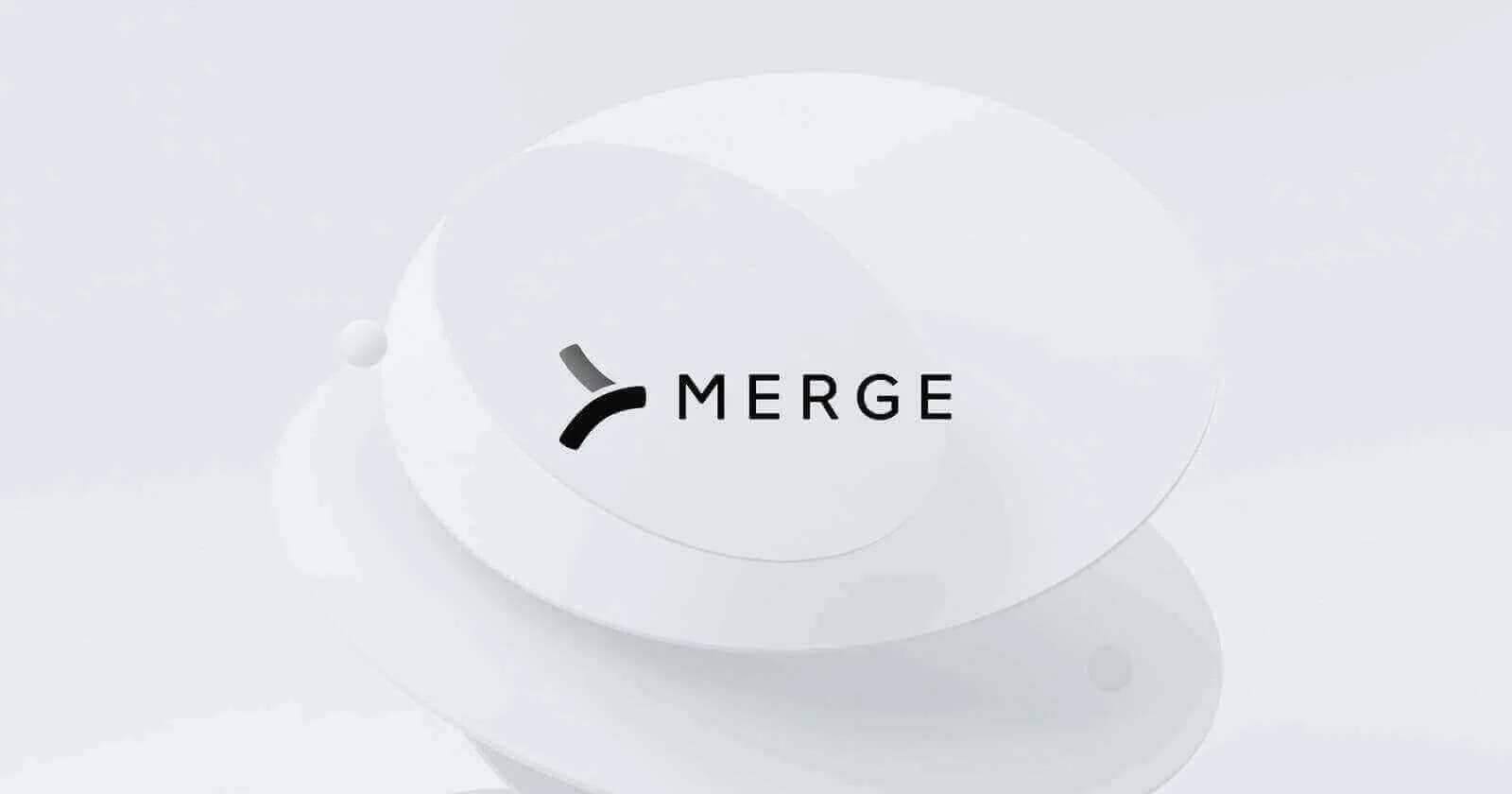
Imagine building a sleek, feature-rich app—and then realizing you need to reinvent the wheel for basic functionalities, like maps, payments, or social logins. Enter software development kits (SDKs): the prebuilt toolkits that allow developers to integrate third-party features into their applications.
Apart from allowing you to reuse prebuilt logic, SDKs also offer access to cutting-edge technology, boost engagement, and even open doors to new revenue streams.
Curious how this translates to the real world? This article explores six SDKs across industries that allow you to do the following:
- Enhance the customer experience: Integrate payment gateways, social logins, and chatbots
- Boost operational efficiency: Connect to logistics platforms, analytics tools, and marketing automation services
- Unlock new revenue streams: Offer in-app purchases, targeted advertising, and subscription models
But before we dive into our SDK examples, let's align on the definition of an SDK.
What is an SDK?
It's a collection of tools and resources that application developers can use to build applications for a specific platform. SDKs typically include prewritten code, libraries, and documentation that make it easier for developers to create applications that interact with the platform.
SDKs help companies save time and money by using prewritten code and libraries. They give access to features and functionality that are not available through other means. They also allow you to create applications that are compatible with a specific platform.
SDKs rely on a crucial ingredient: APIs (application programming interfaces). You can think of APIs as messengers that relay information between your app and the service. They define the specific commands and responses your app can use to access data, features, and functionality.
Related: How to use SDKs effectively
SDK vs API
Just to avoid any confusion, let's clarify the differences between SDKs and APIs.
- Scope: SDKs are broader, encompassing prewritten code, libraries, and documentation alongside APIs. APIs are more focused, strictly defining the communication protocol
- Purpose: SDKs empower developers to build entire applications, while APIs facilitate specific interactions between apps and platforms
- Depth: SDKs delve deeper, providing platform-specific tools and resources. APIs stick to the surface, defining communication rules
- Flexibility: SDKs are language-specific, which means that a provider may not necessarily offer an SDK for your preferred programming language or framework. In contrast, APIs are language-agnostic and can be implemented in most cases. Since SDKs are language-specific, you can use language-related features, such as code autocomplete and syntax highlighting, but only as long as an SDK is available (and maintained) in the language of your choice
- Error handling: SDKs handle HTTP requests and responses for you cleanly, freeing you from the burden of handling failed network requests, interpreting error codes, and setting up retries
SDKs may contain one or more platform-specific APIs to help you achieve the task they are intended for. However, since they usually encompass prewritten libraries and documentation, they are usually easier to get started with when integrating a service into your application.
{{this-blog-only-cta}}
6 real-world examples of SDKs
Let's take a look at some real-world SDKs to better understand how they work and what to look for when choosing one for yourself.
HRIS: Freshteam
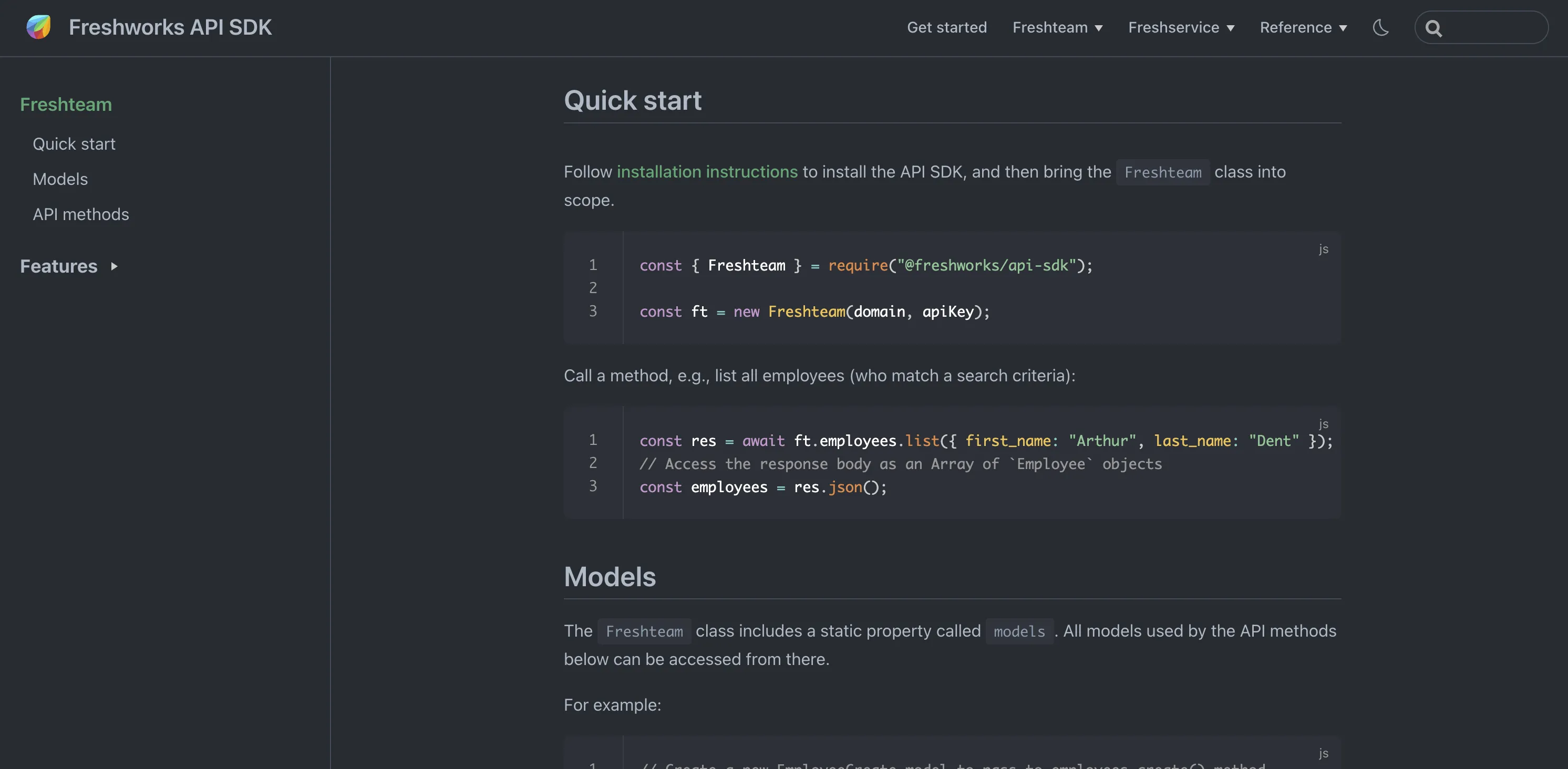
Freshteam, a leading cloud-based HR platform owned by Freshworks, offers an SDK to allow developers to integrate features such as automating tasks and building custom workflows into their applications to improve the overall HR experience in the organization.
The Freshteam SDK provides prewritten code and libraries in various programming languages, such as Python, Java, and Node.js, for interacting with its APIs. This code lets you manage employee data, create and track job applications, process time-offs, and do much more without having to handle HTTP requests and responses manually.
Here's how you can integrate the Freshteam SDK in Node.js:
Freshteam provides comprehensive documentation with code examples, tutorials, and reference guides for each supported language. You can take a look at the Freshworks API SDK documentation to learn more about how you can use it.
Accounting: FreshBooks

FreshBooks, a popular cloud accounting platform, provides an SDK that developers can use to automate tedious accounting tasks and integrate financial data into their applications to save time and ensure accuracy.
The FreshBooks SDKs provide prewritten code and libraries for languages such as Python, Node.js, Java, and PHP, for interacting with the FreshBooks APIs. You can use it to automate tasks like creating and sending invoices, capturing and categorizing expenses directly from your app, and accessing client data.
Here's an example that uses the Python SDK to access the details of a client from your FreshBooks instance:
Related: 5 webhook use cases
Ticketing: Asana

Asana, a popular project management platform, offers an SDK to help you integrate task management functionalities into your application. Integrating with your ticketing system allows you to streamline workflows, helps you automate tasks, and enables collaboration within your applications.
The Asana SDKs provide prewritten code and libraries in various languages, such as JavaScript (Node), Ruby, and Java, to interact with its APIs. You can use it to create and manage tasks, assign tasks to members, and trigger actions based on events to automate workflows, like sending notifications and responding to customer emails.
Here's a quick example of how to use the Asana SDK in a Node.js app:
The Asana SDKs lack a dedicated documentation page on their developer website. However, the GitHub repos contain detailed README files with a substantial number of example code snippets to help you get started quickly.
CRM: Zoho CRM

Zoho CRM, a popular CRM platform, offers an SDK that can help streamline lead management, automate workflows, and integrate CRM data into your applications. This toolkit unlocks a range of possibilities for boosting sales, improving customer relationships, and gaining valuable insights.
The Zoho CRM SDK provides prewritten code and libraries for various languages, such as Java, Python, and Node.js, to interact with the Zoho CRM APIs. You can use the SDKs to do the following:
- Manage leads and contacts: create, update, and track leads and contacts
- Automate sales processes: trigger actions and send notifications based on specific events
- Integrate with other applications: connect your CRM data with other tools for seamless workflows
- Gain insights with reports and dashboards: analyze customer data and track key performance indicators (KPIs)
Here's how you can get started with the Node.js SDK:
As you can see in the preceding example, the Zoho CRM SDK offers several wrappers and helper modules to help you write reliable integrations. However, the documentation may be a little tricky to navigate, especially the pretty large code blocks shared as part of the example code snippets in the docs.
Related: APIs vs integrations
Marketing automation: SendGrid

SendGrid, a leading cloud-based email delivery platform, offers a detailed SDK to help developers send and manage emails programmatically. This toolkit simplifies email sending, streamlines automation, and ensures exceptional deliverability to help you reach your audience effectively.
The SendGrid SDKs provide prewritten code and libraries in various languages, such as Python, Node.js, and Ruby, to interact with the SendGrid APIs. The SDKs allow you to do the following:
- Send transactional emails: trigger automated emails based on events in your app, such as order confirmations or password resets
- Track email performance: monitor open rates, click-throughs, and other key metrics for data-driven insights
- Segment your audience: send targeted emails to specific groups based on preferences and behavior
Here's a quick code snippet to help you get started with the SendGrid Python SDK:
Compared to some of the other SDKs discussed, SendGrid offers more comprehensive documentation and getting-started guides for its SDK. Since it's backed by Twilio, the developer experience offered by SendGrid is better than that of most companies offering SDKs.
File Storage: Box
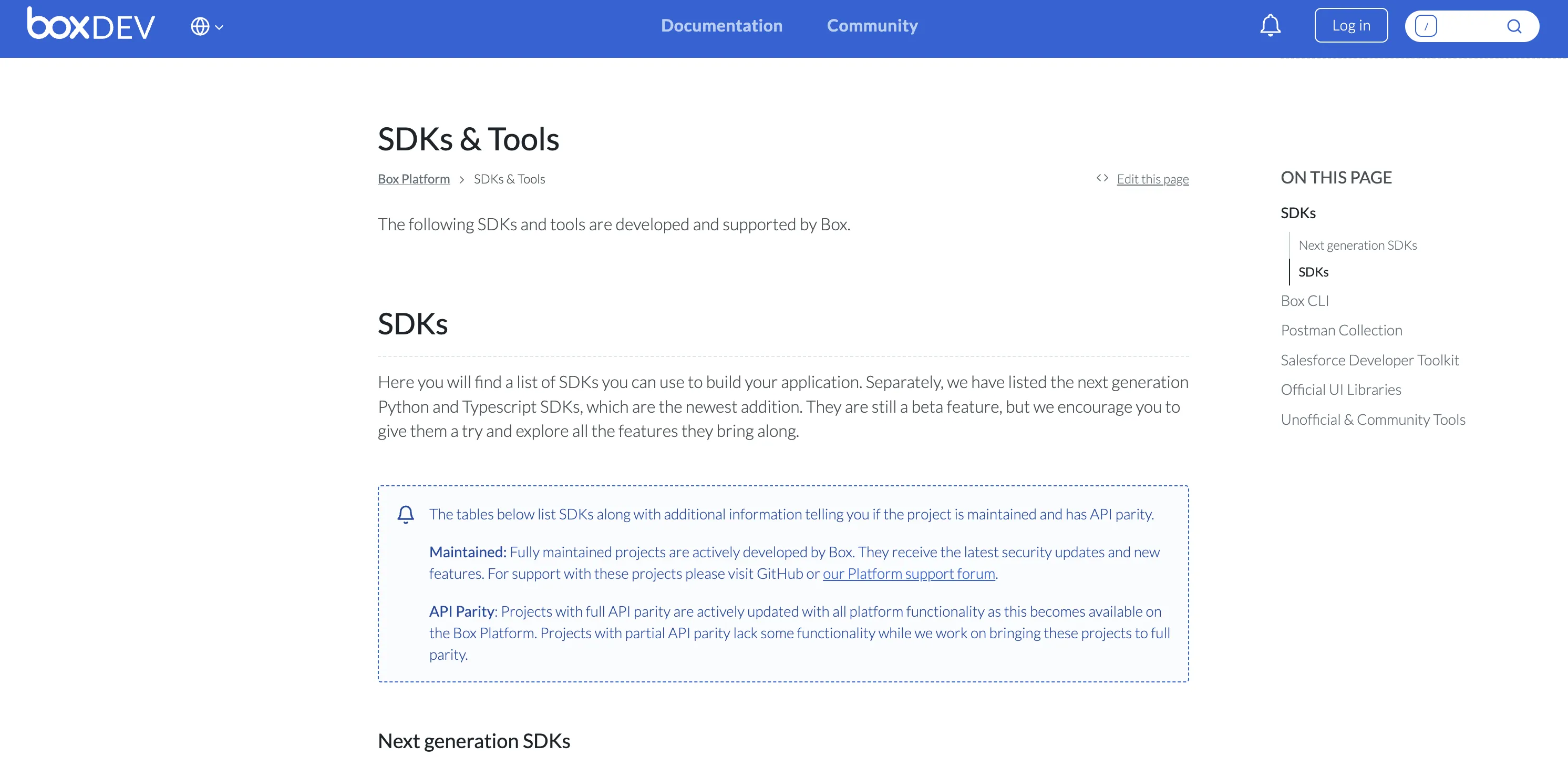
Box, a cloud-based content management platform, offers an SDK that lets developers integrate secure file-sharing and collaboration features into their applications. This developer-friendly toolkit unlocks a range of possibilities for managing files, streamlining workflows, and boosting team collaboration.
Box provides prewritten code and libraries for various languages, such as Python, Java, and Node.js, to interact with its APIs. The SDKs allow you to do the following:
- Upload and download files: manage various file types securely within your application
- Collaborate on documents: enable real-time editing and sharing of files within teams
- Automate workflows: trigger actions based on specific events, such as file uploads or approvals
Getting started with the Box SDK is easy. Here's an example in Node.js:
While Box offers SDKs across a wide range of platforms, including TypeScript and mobile, the documentation is bare-bones. It's provided in the GitHub repositories of the SDKs as a collection of Markdown files, and navigating through the files and finding the relevant methods and examples can sometimes get tricky.
Merge: one SDK for managing all your integrations

Merge—a single API that lets you add hundreds of integrations to your product—allows you to build to hundreds of APIs through one SDK, saving your engineers countless time and effort.
The Merge SDK also provides prewritten code libraries for various languages such as Python, Node.js, Go, Java, and Ruby, all but ensuring your team can use it in their preferred language.
You can learn more about Merge's SDK and its platform more broadly by scheduling a demo with one of our integration experts.