How to use python to get tickets from Zendesk
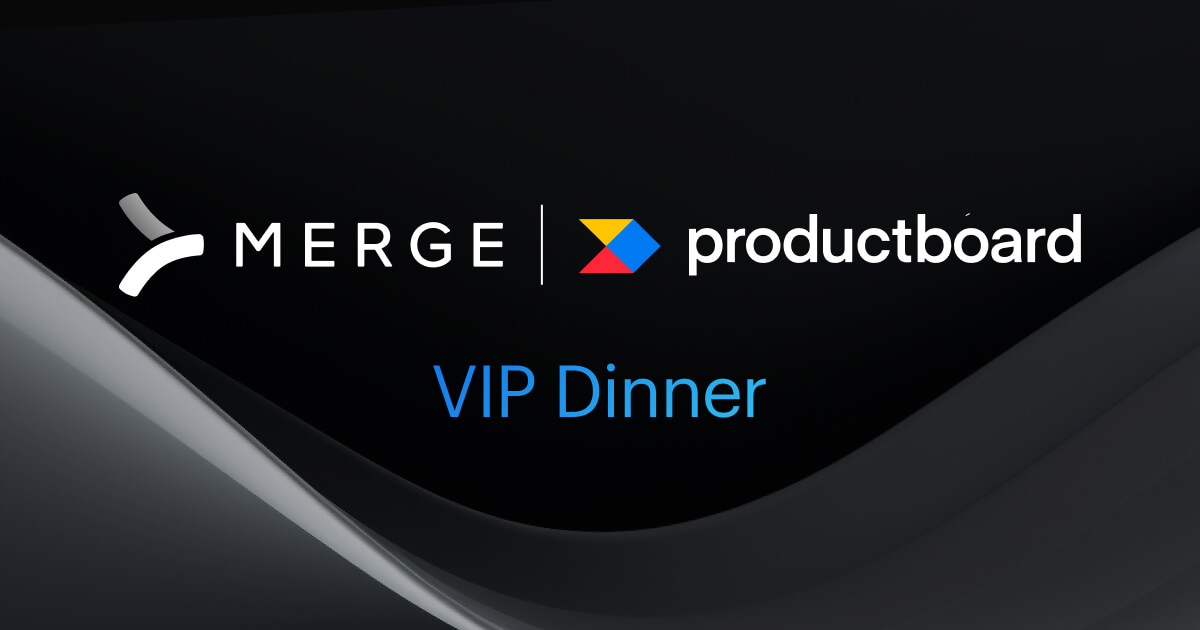
This tutorial is part of a series on building product integrations.
Zendesk is more than just a ticketing system – it's a unified platform that many businesses use to categorize, manage, respond to, and solve customer inquiries and complaints. The platform simplifies the customer support process by transforming all incoming requests from different channels into tickets, which are easier for support teams to track and handle.
Zendesk's API functionalities makes it easy for developers to integrate with other systems and programmatically pull ticket data for analysis or to meet other business use cases like building custom dashboards, conducting in-depth analytics, automating workflows, etc.
In this tutorial, we’ll cover:
- How to establish authentication for the Zendesk API
- How to pull normalized data from Zendesk by making get requests to retrieve tickets . This normalized method will enable you to manage your data more effectively.
- How to use a single API endpoint to connect with hundreds of third-party ticketing systems. This will streamline your operations and enable better integration across various platforms.
Related: How to GET GitHub repositories
Authentication
To authenticate with the Zendesk API you need a specific header format for the HTTP requests. The header needs to include an authorization field, using the Basic access authentication method. The string following 'Basic' should be a Base64 encoded string that represents your email address (used for the Zendesk account), followed by '/token:', and ending with your API key. The entire string should be encoded in Base64.
Note: the '/token:' part is a literal string - it doesn't change.
Make sure that your email and API key are correct, and that you properly encode the whole string. This authentication method provides a secure way to interact with the Zendesk API.
Here’s a simple Python function to generate the required authentication header:
Replace 'email' and 'api_key' with your actual email address and Zendesk API key respectively. The function will return the complete authorization header value.
How to get tickets from Zendesk
This Python script uses the <code class='blog_inline-code'>requests</code> library to make HTTP requests to the Zendesk API. The script begins by defining the email, API key, and domain of your Zendesk account.
The email and API key are used to create a <code class='blog_inline-code'>Basic Auth</code> header for the requests to the API. The domain is used to construct the URL for the requests.
The script then enters a loop. In each iteration of the loop, the script makes a GET request to the Zendesk API to get a page of tickets. Tickets are extracted from the response and printed out.
Then, the script checks if there are more pages of tickets to get. If there are, the script updates the URL for the next request with the URL of the next page of tickets. If not, the script breaks out of the loop.
For reference, here’s an example of an individual item returned by this API Endpoint:
Related: What you need to know about reading API documentation
Use a unified ticketing API to integrate at scale
In this article, we walked through the process of getting tickets from Zendesk using Python. But what if your clients use a variety of ticketing systems?
You can integrate your product with all of your clients' ticketing solutions to sync tasks—among other types of data—by leveraging Merge, the leading Unified API solution.
Simply build to Merge's Ticketing and Project Management Unified API to offer 30+ ticketing integrations. You'll also be able to access Merge's robust Integrations Management features to identify and troubleshoot issues quickly and successfully.
You can learn more about Merge by scheduling a demo with one of our integration experts.