How to create a deal in Pipedrive with a Unified API
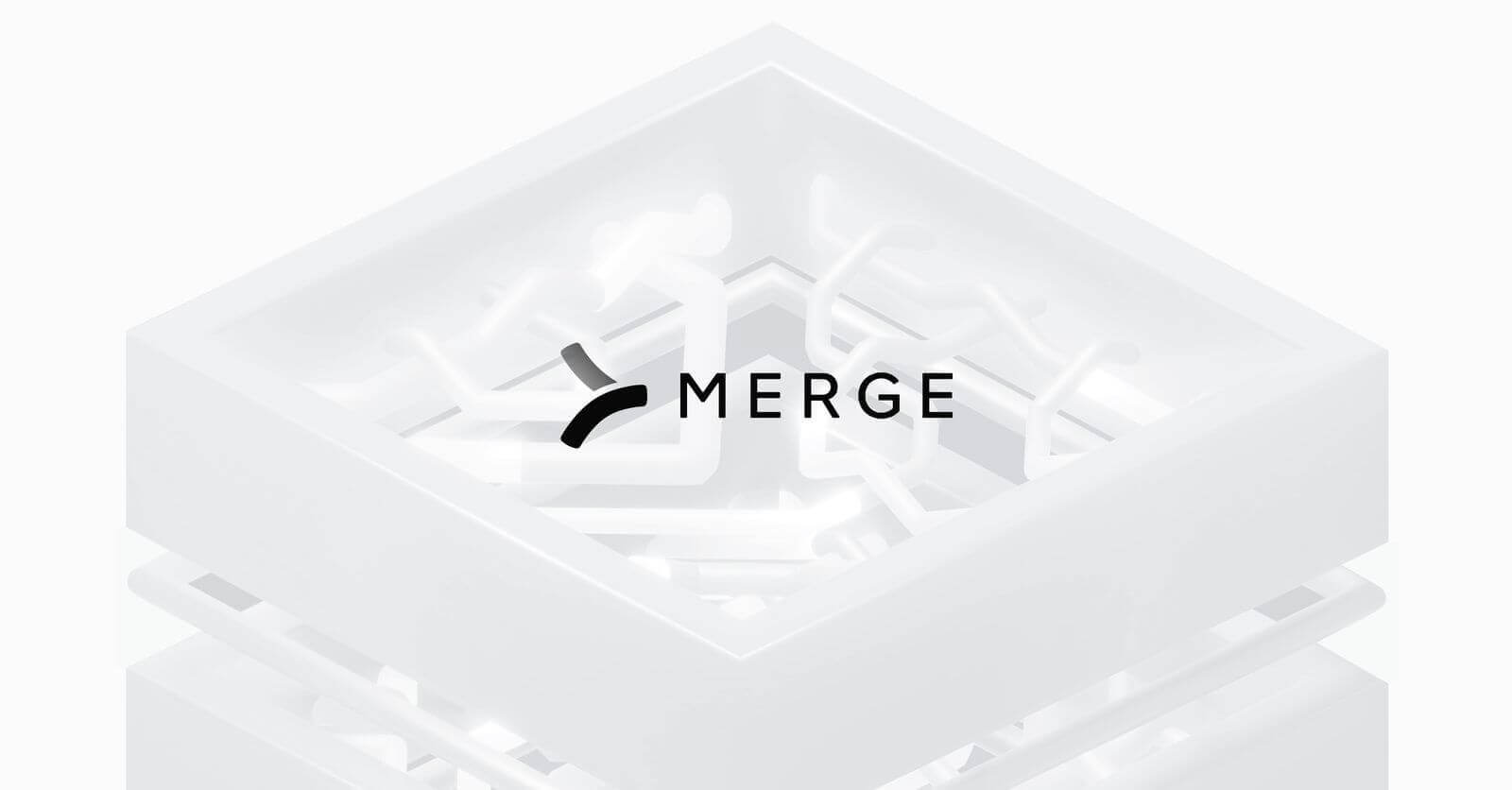
Customer relationship management (CRM) systems help companies manage customer relationships, from leads through opportunities to sales and beyond. They typically include features for storing and tracking customer contact information, managing sales leads, tracking sales opportunities, and providing customer support.
A unified API like that of Merge can help you integrate various CRM systems with each other and into your applications and workflows. For instance, as a software developer for a technology company, you might want the sales team in your organization to be able to create deals in a CRM like Pipedrive as soon as they finish meetings with potential customers. You can use the Merge Unified API to create the deal so that you can automate the process and save time.
In this article, you will learn more about how Merge's unified API can help you automate tasks in CRMs, and you'll see how to use it to create a deal in Pipedrive.
Using Merge to Automate Tasks on Pipedrive
Pipedrive is a popular cloud-based CRM software that provides a user-friendly and intuitive interface that enables sales teams to track leads, manage deals, and close sales efficiently.
If you want to automate any tasks on Pipedrive as part of an integration with other tools, you could use Pipedrive's API. However, it would involve some development effort and maintenance overhead since this will likely not be the only tool and API you work with. Different tools' APIs have varying data models, endpoints, and authentication mechanisms. Relying on individual APIs could make it challenging to manage data consistency and scalability across multiple platforms, resulting in more complex code and potential issues during updates or changes to the integration.
Merge's unified API addresses the challenges of integrating with multiple platforms by providing a single, standardized interface to access data and functionality from various APIs.
Merge allows you to streamline the integration process, reducing development effort and maintenance overhead as you no longer need to manage multiple APIs with varying data models and endpoints. In addition to a more efficient and robust integration process, Merge also simplifies updates and changes to the integration, saving you significant time and effort.
Related: How to GET tasks from Pipedrive
Creating a Deal in Pipedrive with Merge's Unified API
Let's see how you can use Merge's unified API to create a deal in Pipedrive.
You will be using the Python CRM SDK, but the Merge SDK is also available in other languages such as Ruby, Java, and Node TypeScript.
Prerequisites
To follow along, you will need the following:
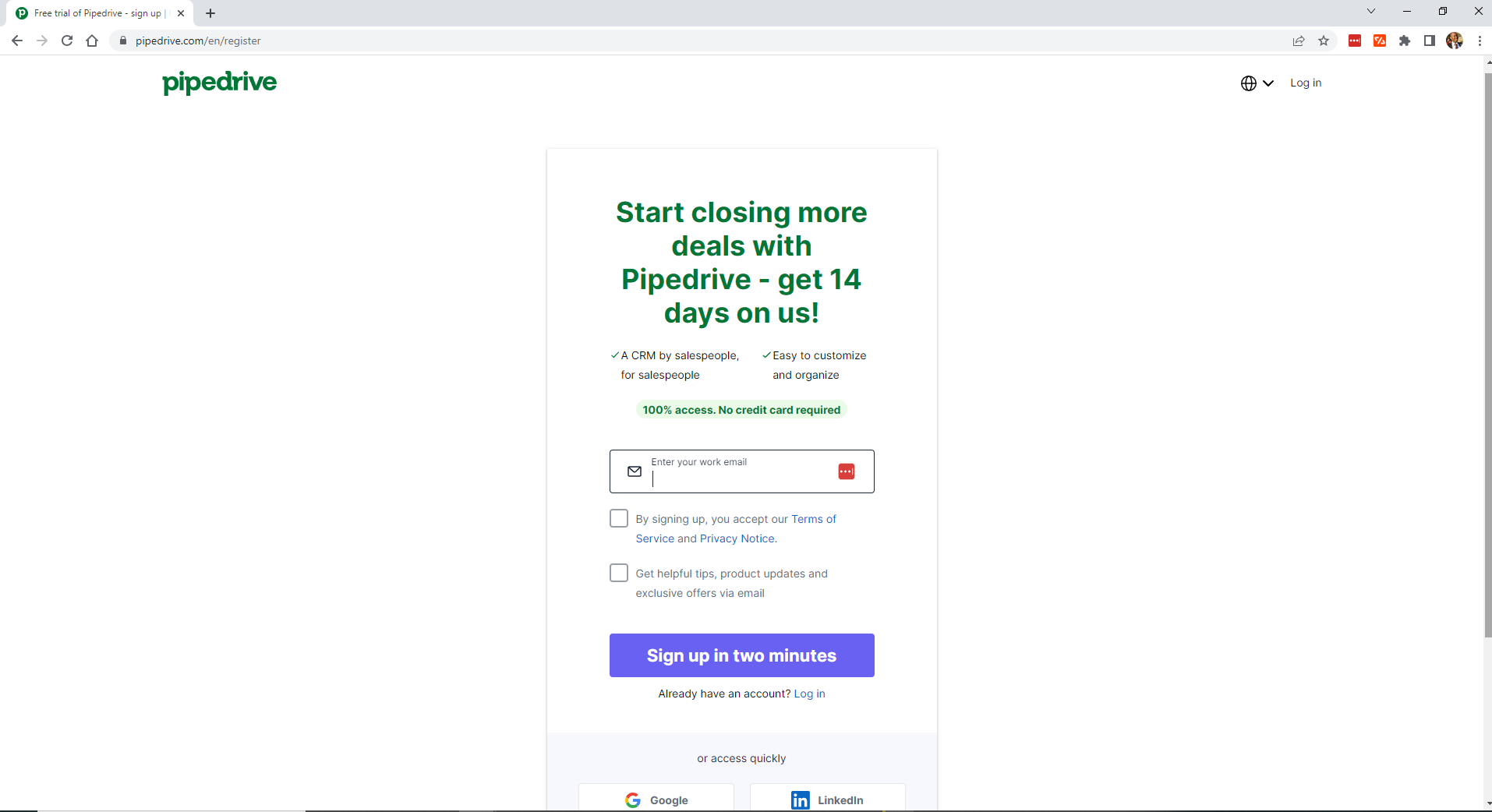
- A Merge account
- A Python installation. Be sure to use version 3.11 or later.
- A virtual environment using venv. Run the command pip install virtualenv to install the virtual environment tool.
Link Pipedrive with Merge
For Merge to interact with Pipedrive, you have to link your Pipedrive account with Merge.
Log in to your Merge account, select Linked Accounts, and click Test Linked Accounts since you don't want to use production for a tutorial.
If you're using Merge for the first time, you'll see that there are no linked accounts.
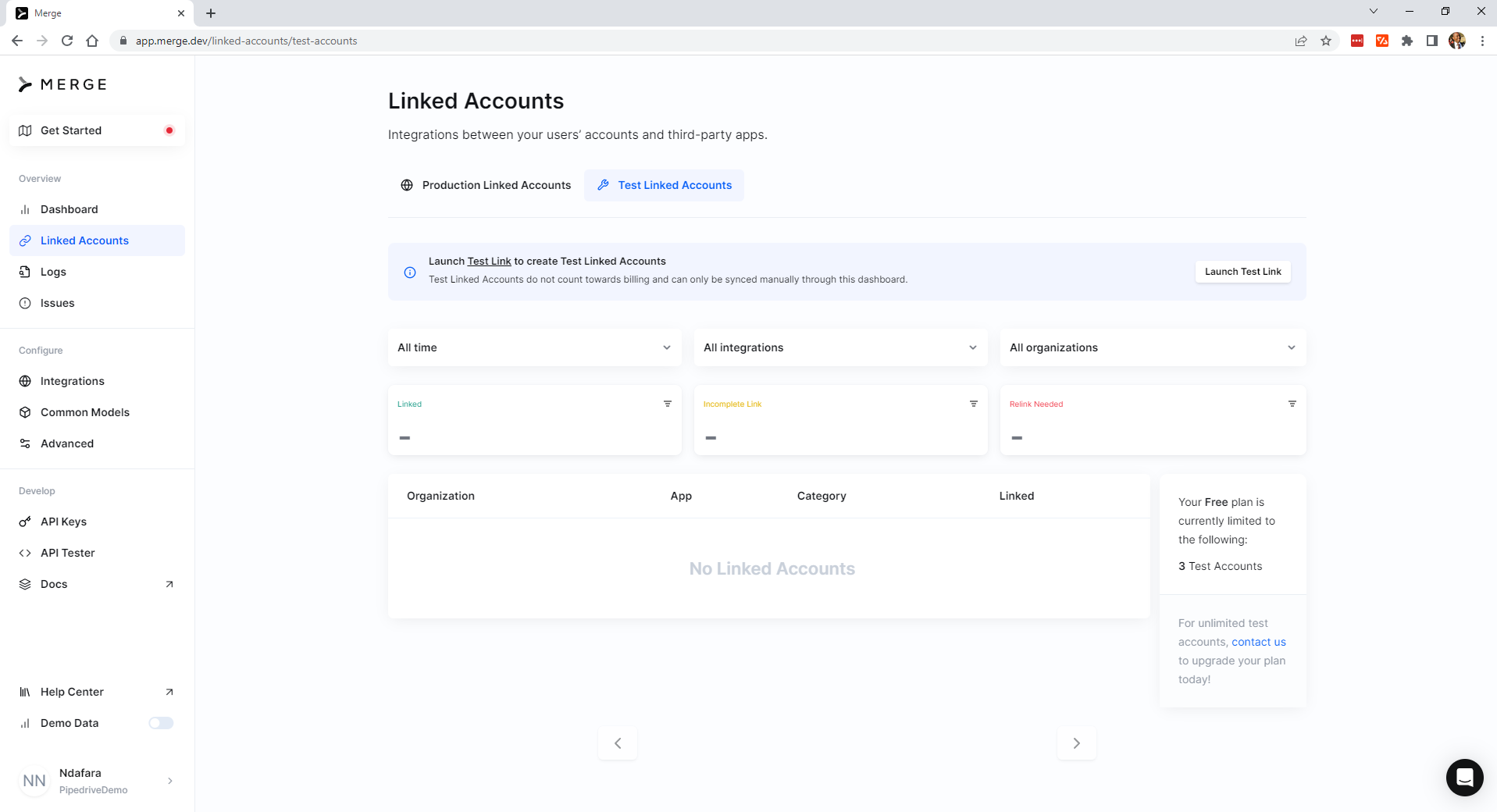
Click on the Launch Test Link button.
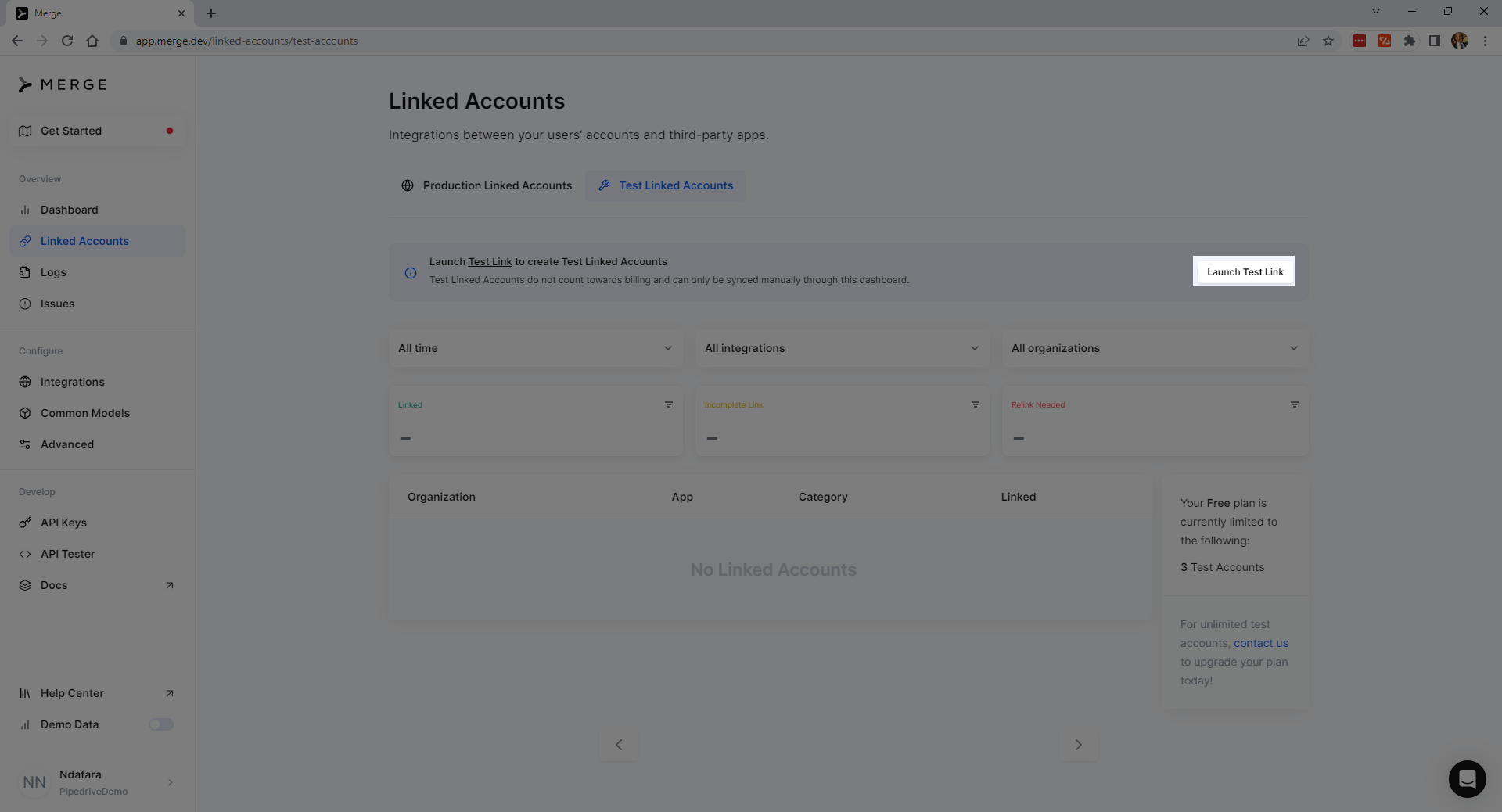
This will open the Select Category dialog. Choose Customer Relationship Management.
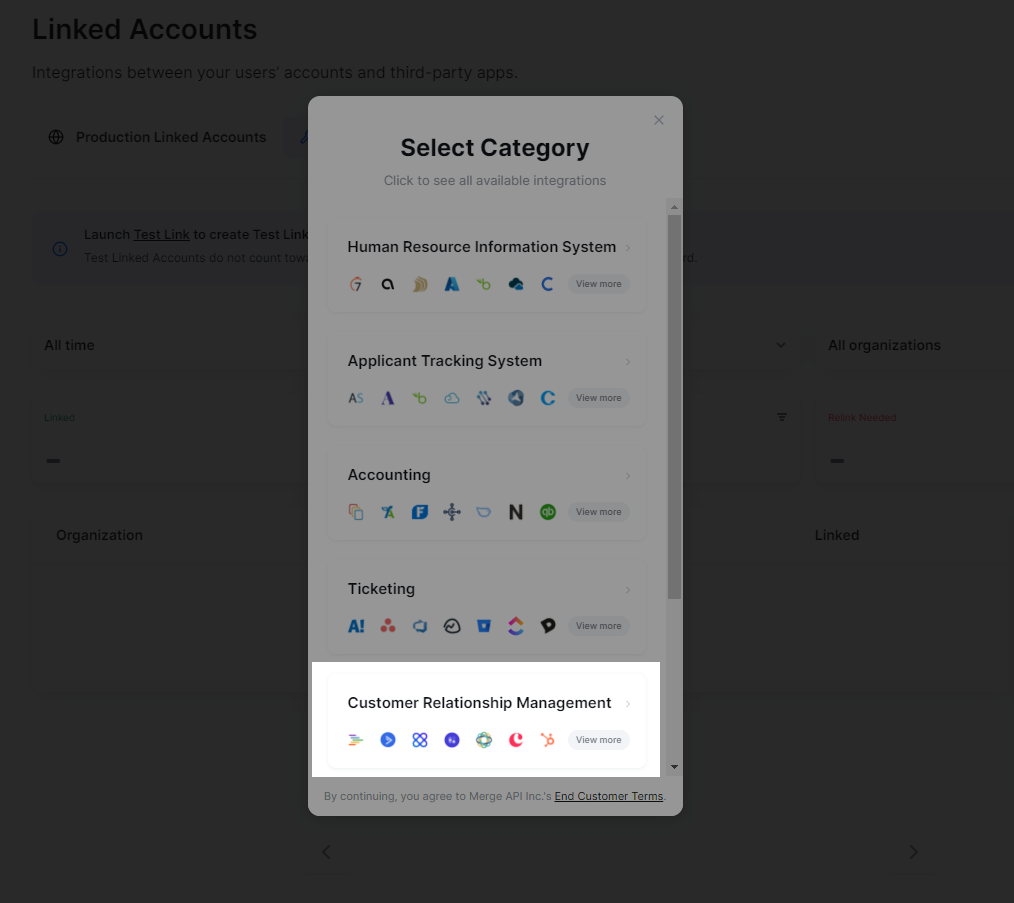
In the Select Integration dialog, select Pipedrive.
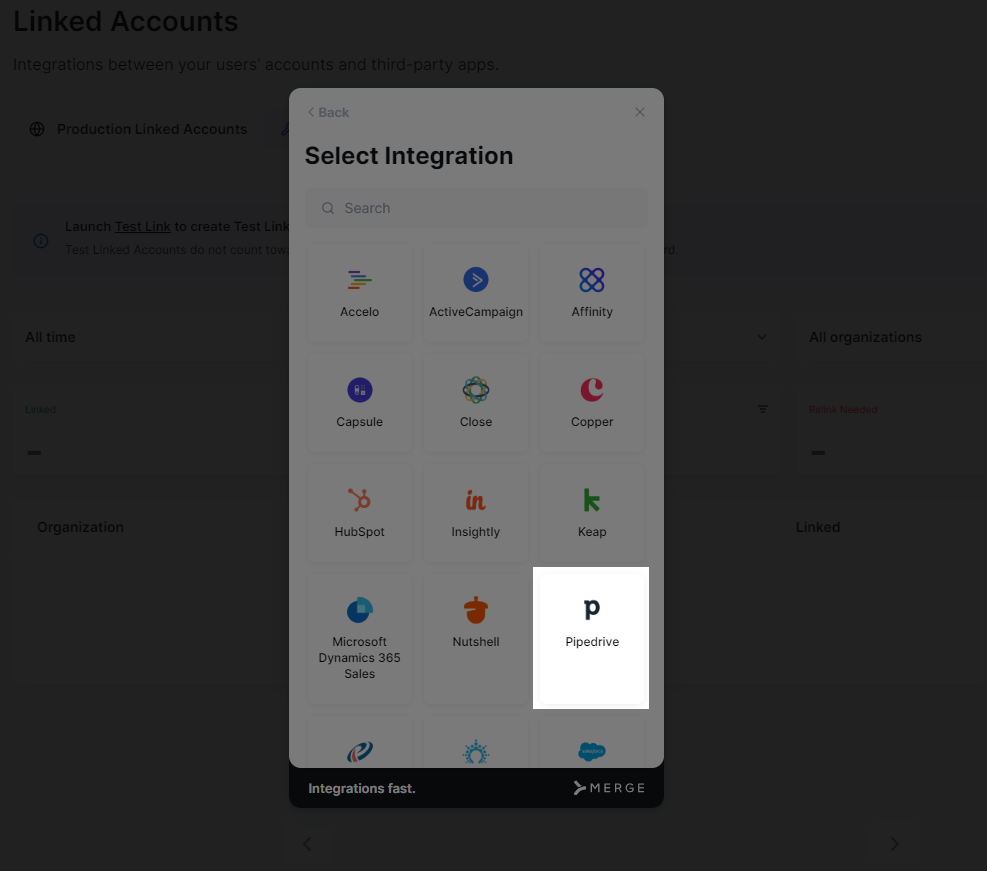
Merge will then start setting up the integration.
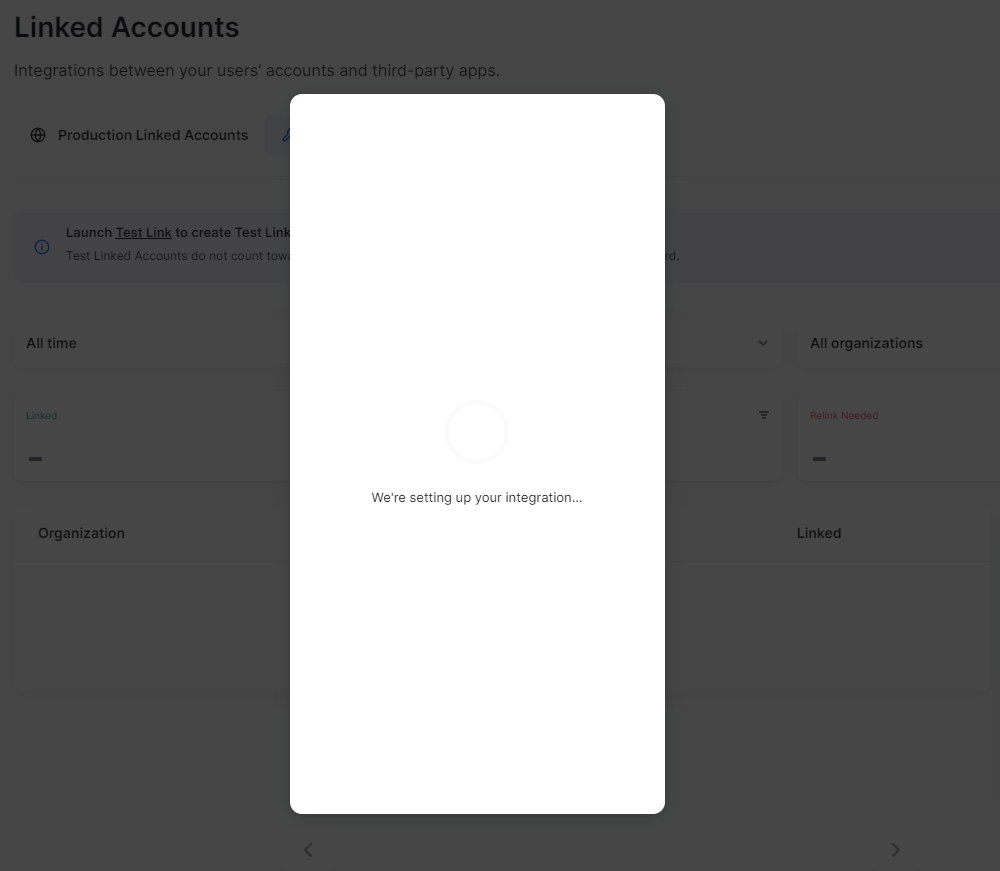
You'll have to provide consent for Merge to read the data in your Pipedrive account. Click Continue.
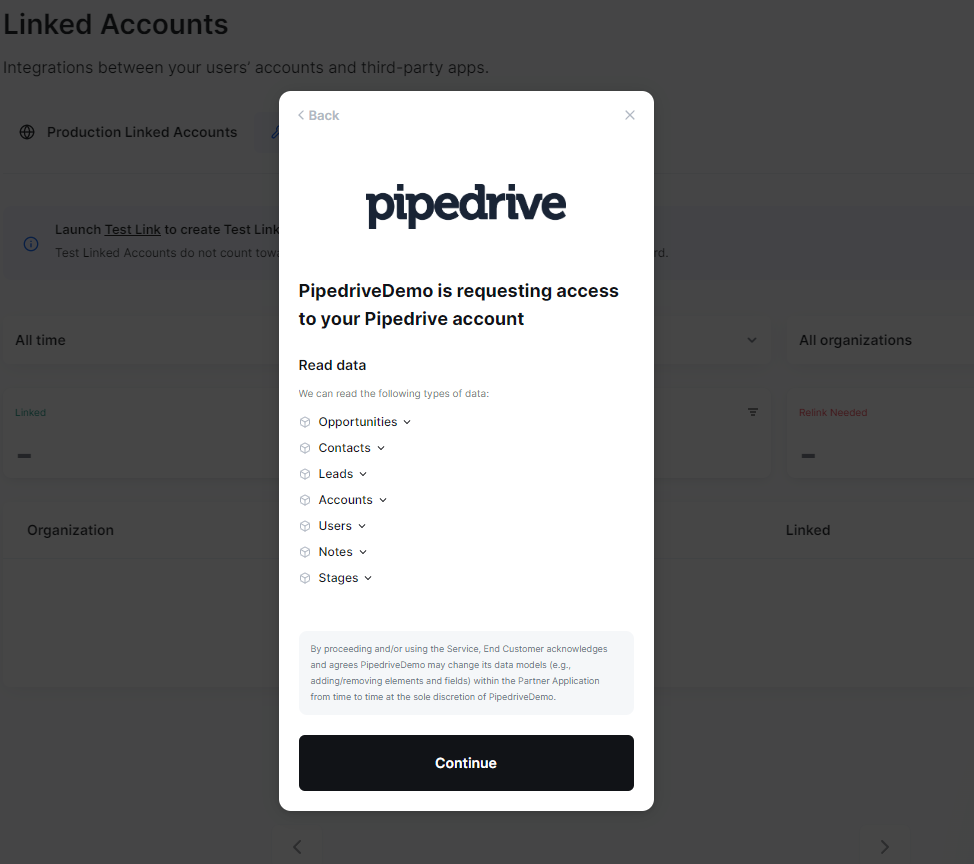
Next, you'll have to specify how you want to authenticate with Pipedrive. Keep the default choice of I want to use my API Key or Access Token to authenticate and click Next.
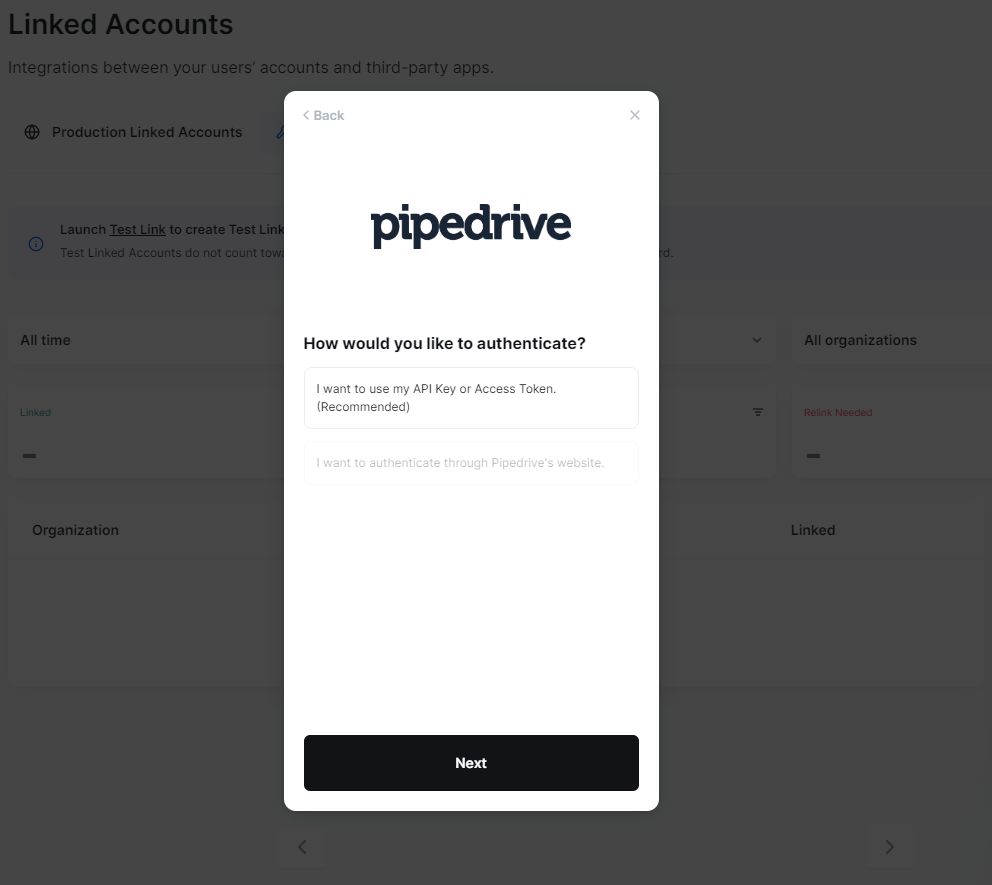
Merge gives you detailed instructions on how to get the API Key from your Pipedrive account.
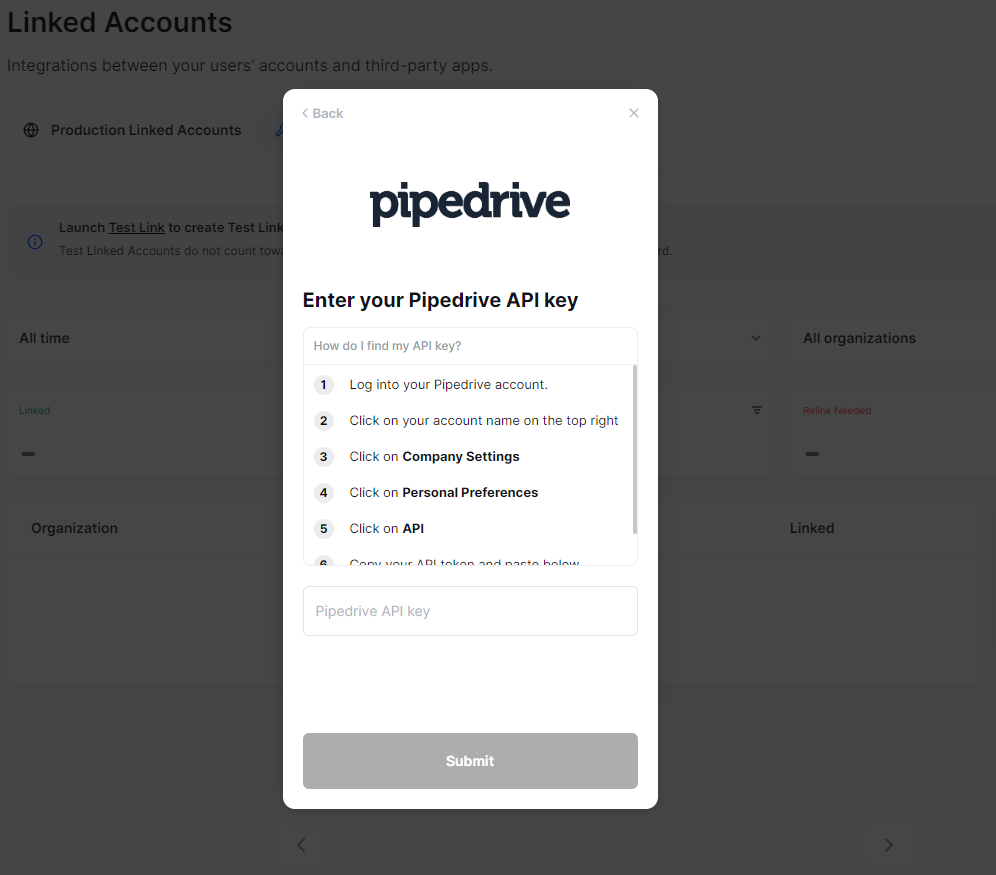
Log in to your Pipedrive account and click on your account name in the top right corner and then on Personal Preferences.
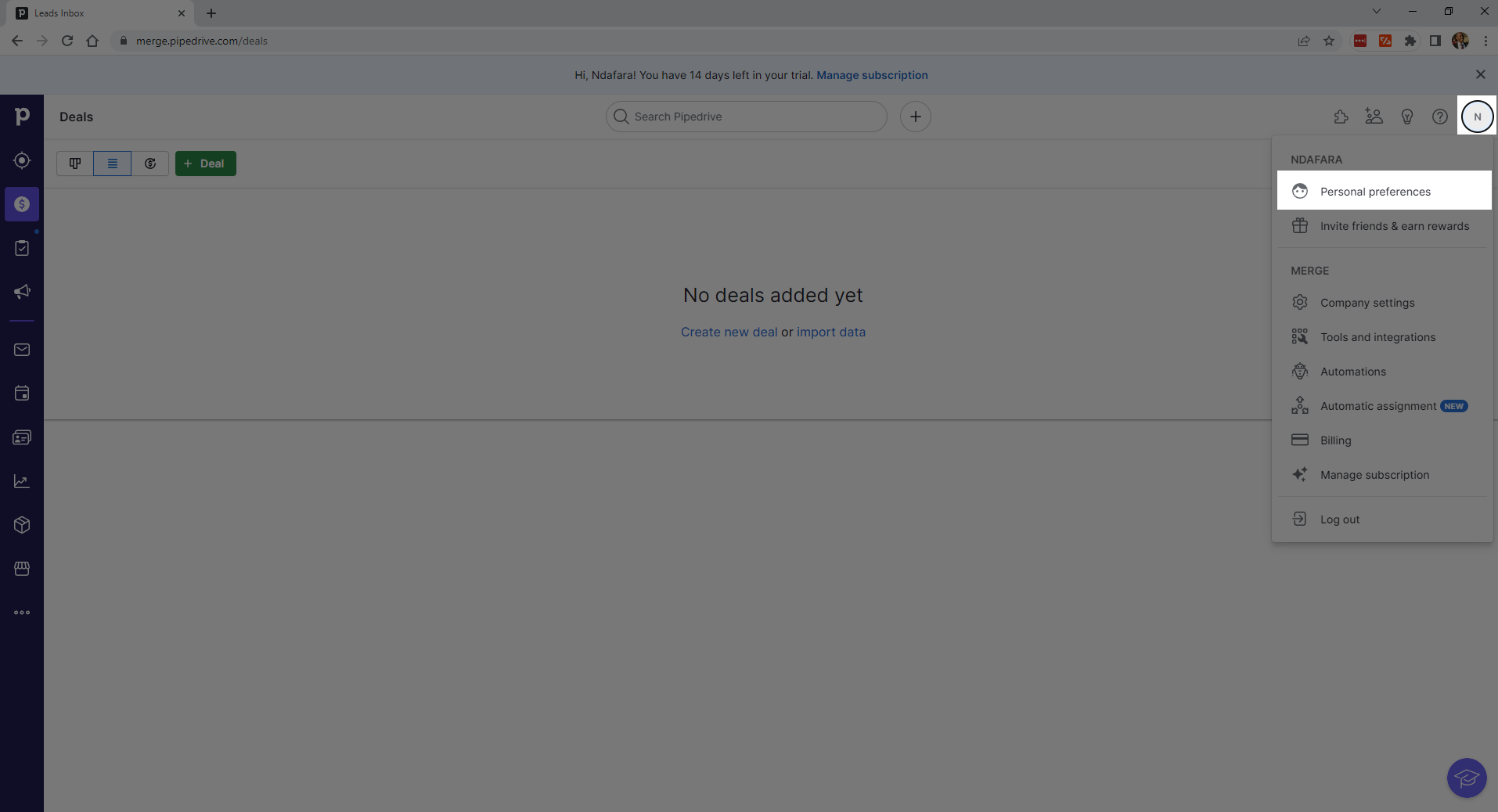
In the Personal Preferences page, select the API tab and copy the API key provided.
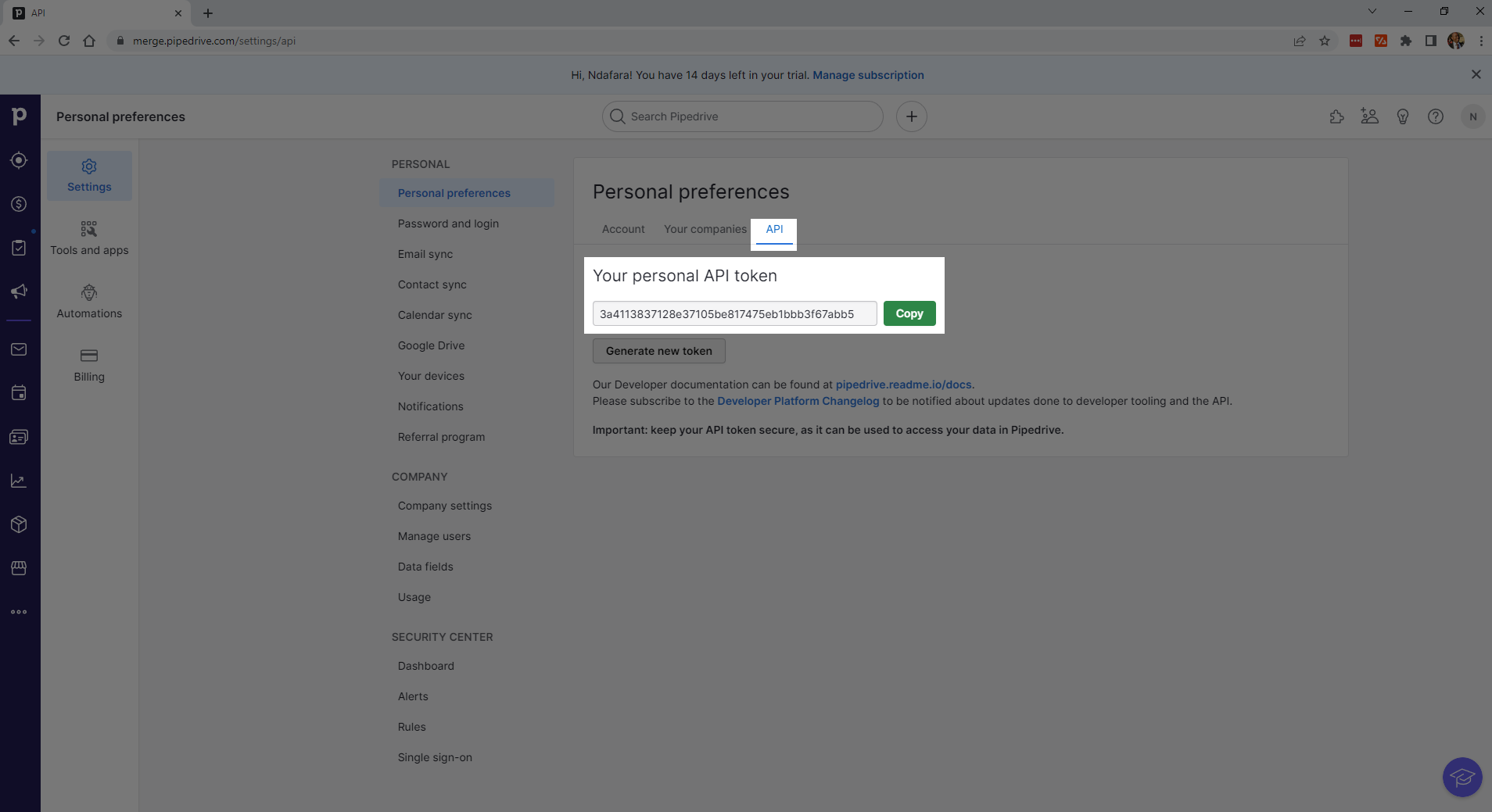
Back in Merge, paste the API key you copied and click on Submit.
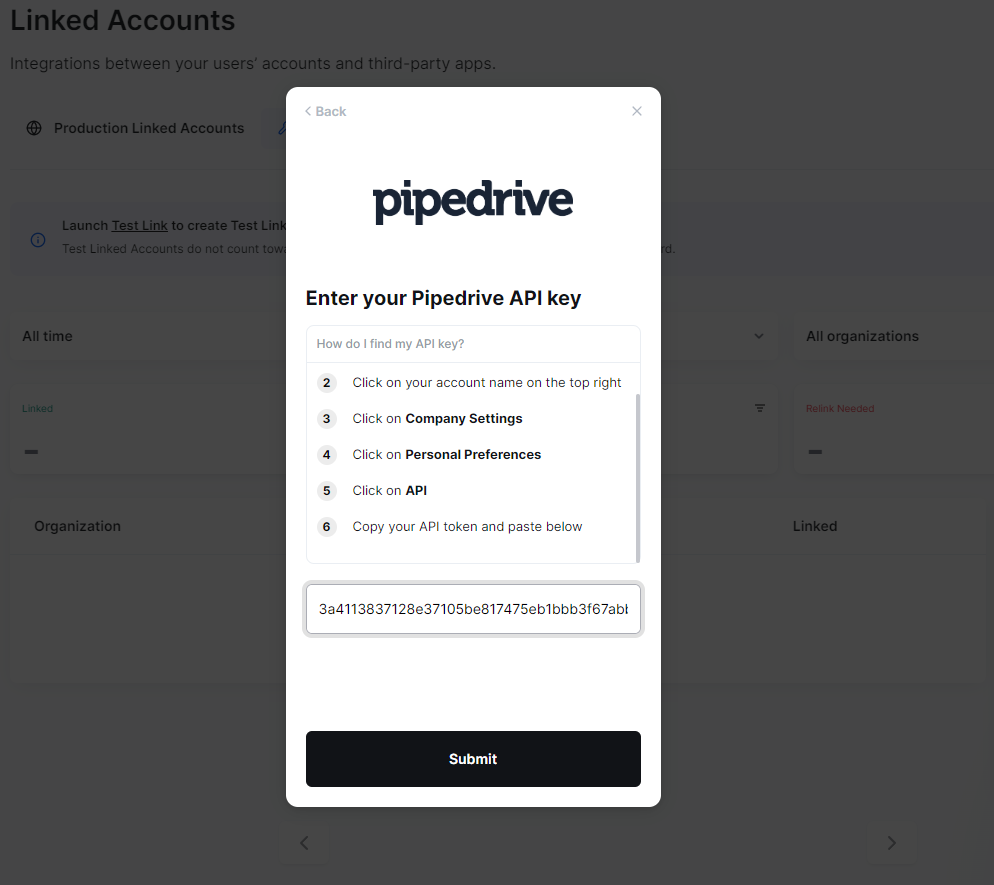
Provide your Pipedrive subdomain and click on Submit.
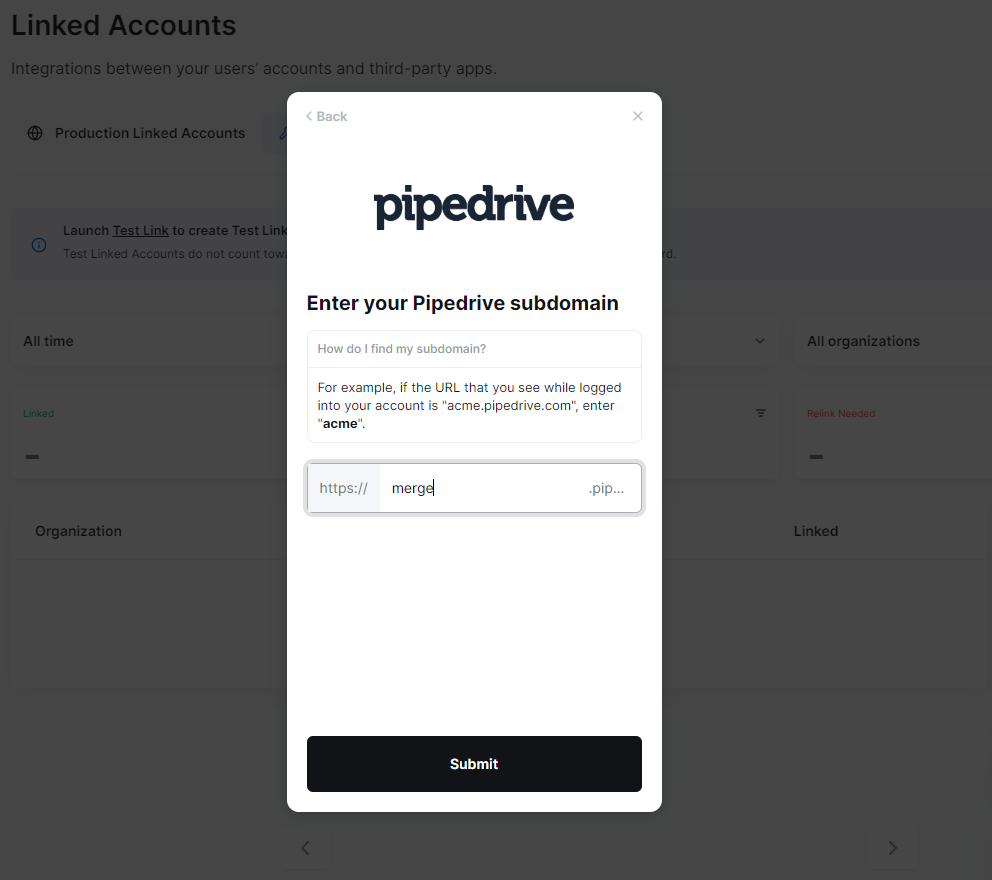
Merge will continue to set up the integration.
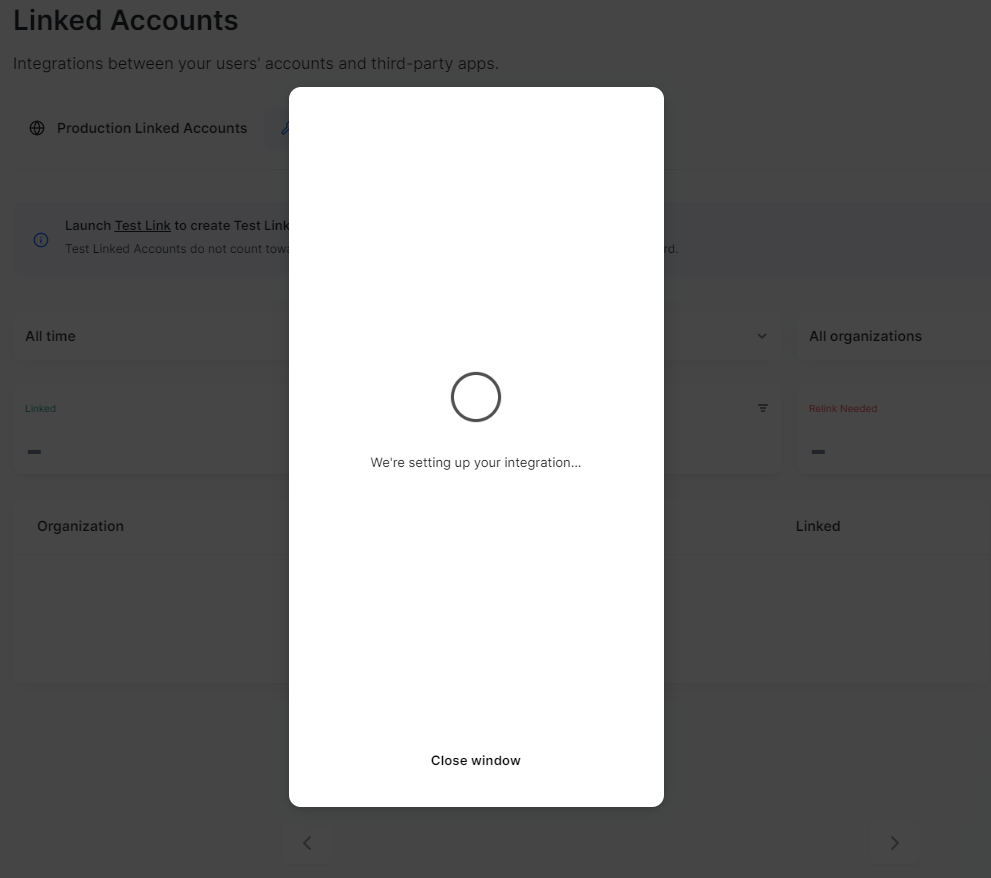
You will get a confirmation message upon a successful setup.
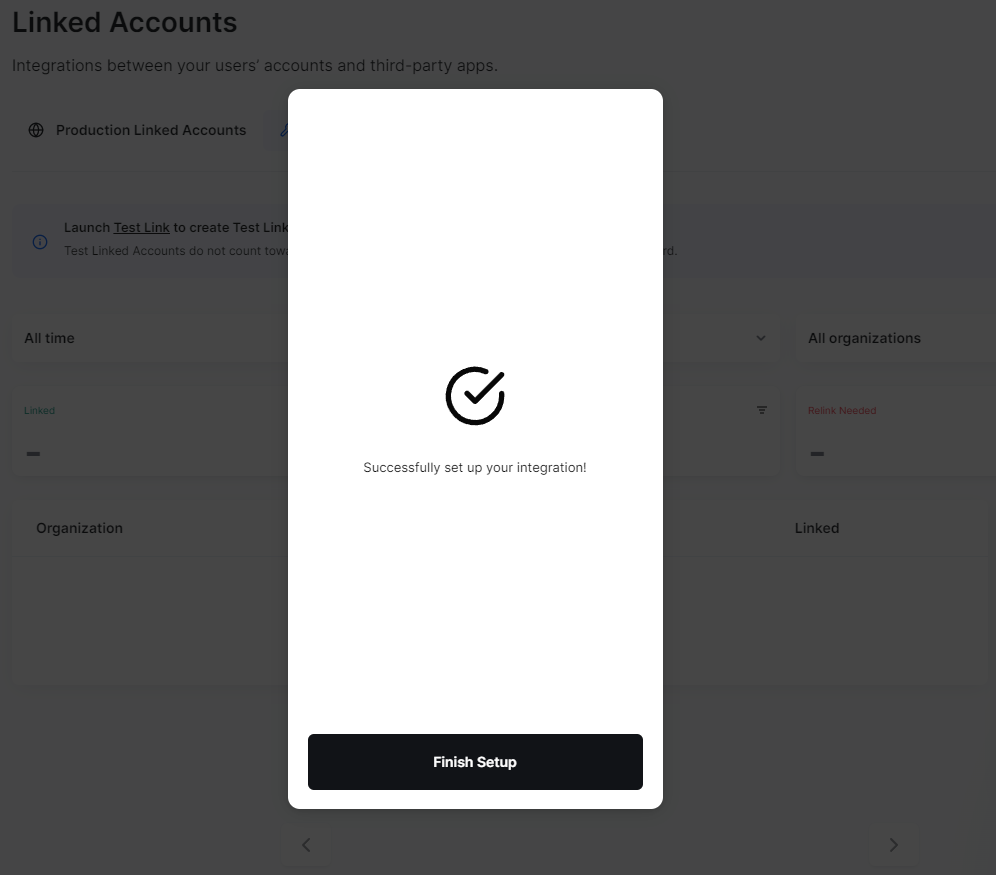
When you click Finish Setup, you should see Pipedrive on your linked accounts.

Get Merge API Key and Account Token
To make calls to Merge using the SDK, you will need to get a test access key and account token.
To get the key, select API Keys in the left-hand pane of your Merge screen. Because you are using the testing environment, copy and keep the test key under Test Access Keys. You will use this later in your code.

To get the account token, click Linked Accounts on the left-hand pane of your Merge screen. Click on the integration for which you want to get the account token—in this case, Pipedrive. You can find the account token towards the right of the screen. Copy and keep it too for your code.
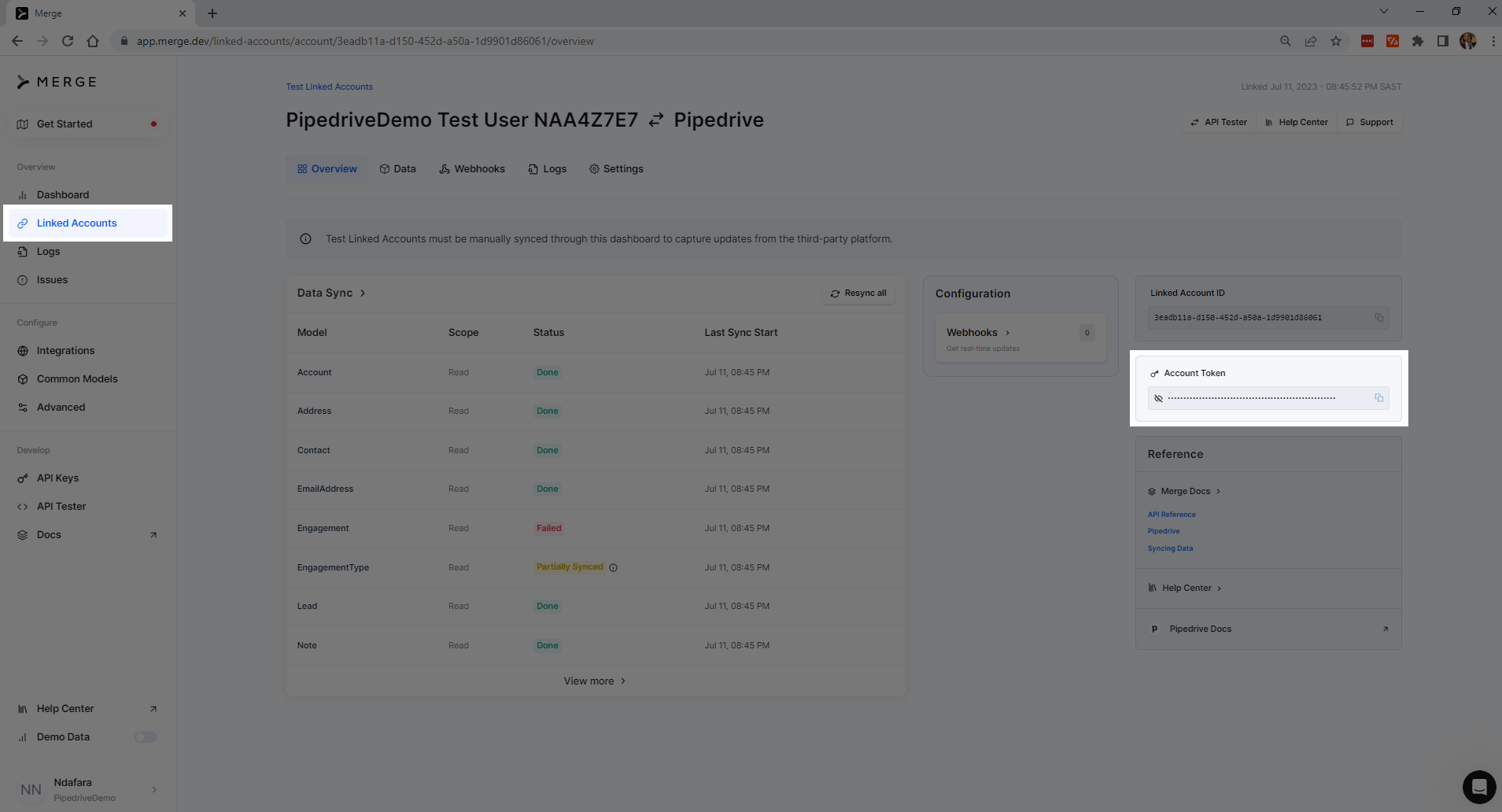
Enable Read-Write Permissions on the Common Model
You will be using the common model provided by Merge, and the permissions here are set to read by default. Creating a deal is a write, though, so you have to enable the write permissions on the opportunity model.
On your Merge dashboard, click Common Models on the left and then CRM. Click on the Opportunity model, and then enable Read+Write.
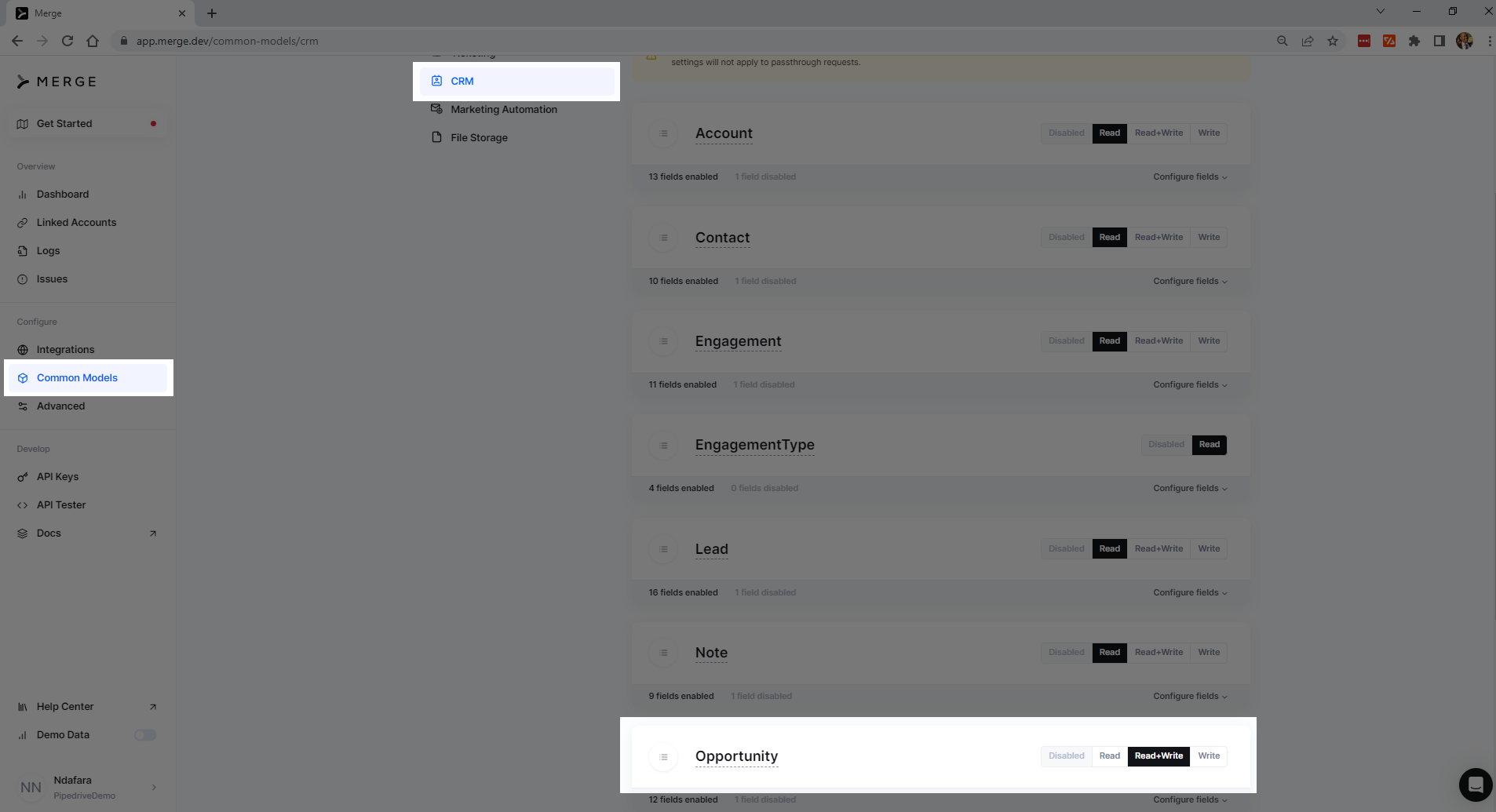
Create an Opportunity in Pipedrive Using the Merge CRM SDK
You're now ready to create a deal—or an opportunity as it's called in Pipedrive—using the Merge CRM SDK in Python.
Start by creating a new directory and name it CreateOpportunityInPipedrive.
In the directory, run the following command to create a virtual environment called creatingOpportunityInPipedrive: <code class="blog_inline-code">python -m venv creatingOpportunityInPipedrive</code>
After creating the virtual environment, you need to activate it. If you are on Windows, run the following command: <code class="blog_inline-code">creatingOpportunityInPipedrive\Scripts\activate</code>
If you are on Linux or Mac, run the following command: <code class="blog_inline-code">source creatingOpportunityInPipedrive/bin/activate</code>
Once the virtual environment is activated, install the Merge CRM SDK by running the following command: <code class="blog_inline-code">pip install --upgrade MergePythonClient</code>
The Merge Python library provides access to the Merge API from Python. This tutorial uses version 0.2.5+ of the Merge Python Client.
You will get the following output if the installation was successful:
After installing the Merge Python Client, create a file called opportunities.py and paste the following code in it:
This code creates an opportunity with name, description, and amount fields. You can also include other fields such as last_activity_date, close_date, and stage.
Don't forget to replace YOUR_API_TOKEN and YOUR_ACCOUNT_TOKEN with your actual values.
As you can see below, there are currently no opportunities in Pipedrive.
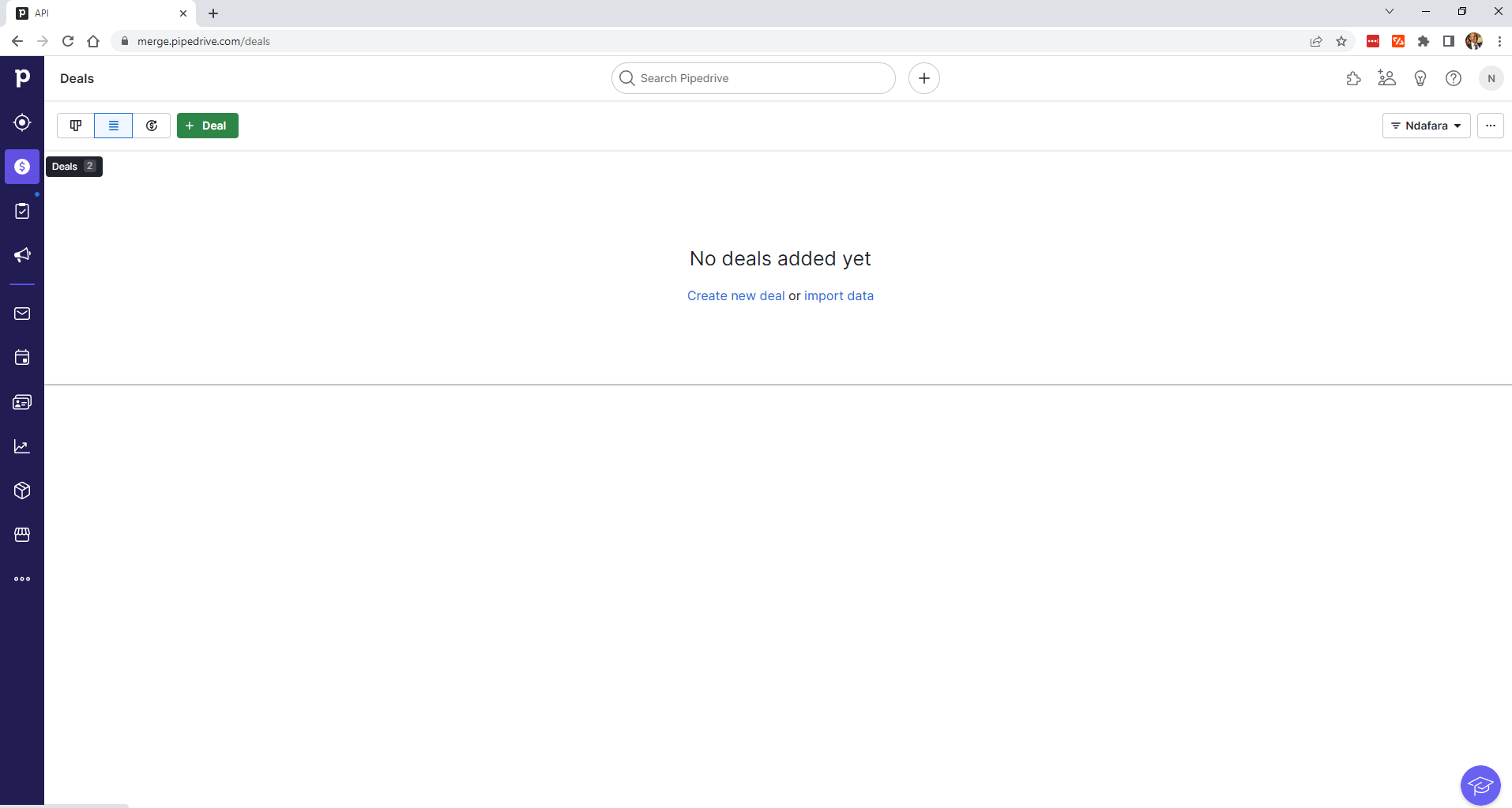
Run the following command to execute the script: <code class="blog_inline-code">python opportunities.py</code>
If everything executes well, a deal will be created in Pipedrive, and you will get the following output, which shows that there are no errors or warnings:
If you now go back to Pipedrive, you will see that there is now one deal with the title Unified API Solution and a value of 50,000 in the currency of your Pipedrive account.
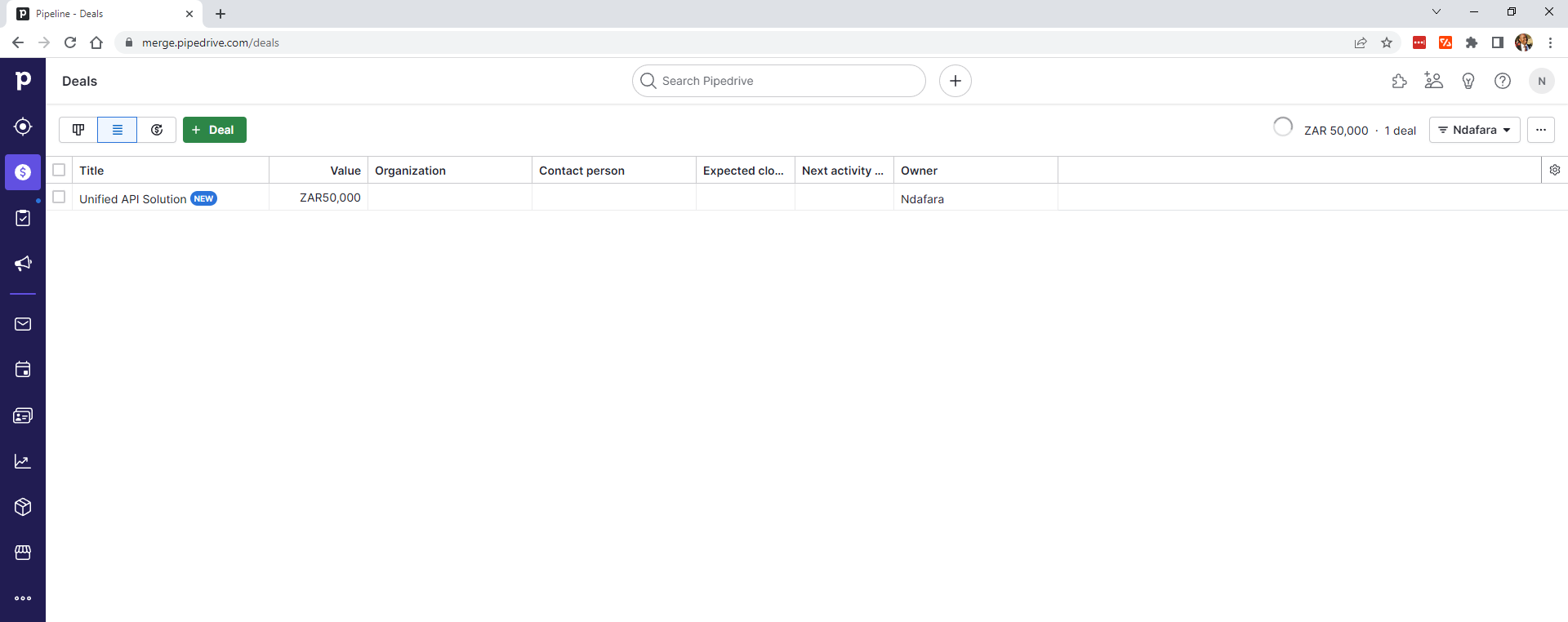
Conclusion
In this article, you saw how you can use Merge's unified API to automate creating a deal in Pipedrive.
This isn't the only use case for Merge's unified API. It can also help you automate other CRM tasks and integrate your CRM systems with other applications in your organization. Its simplicity and flexibility help improve your efficiency to save time and costs.
Sign up for free to explore Merge's full potential.