How to fetch employees from Factorial using Python
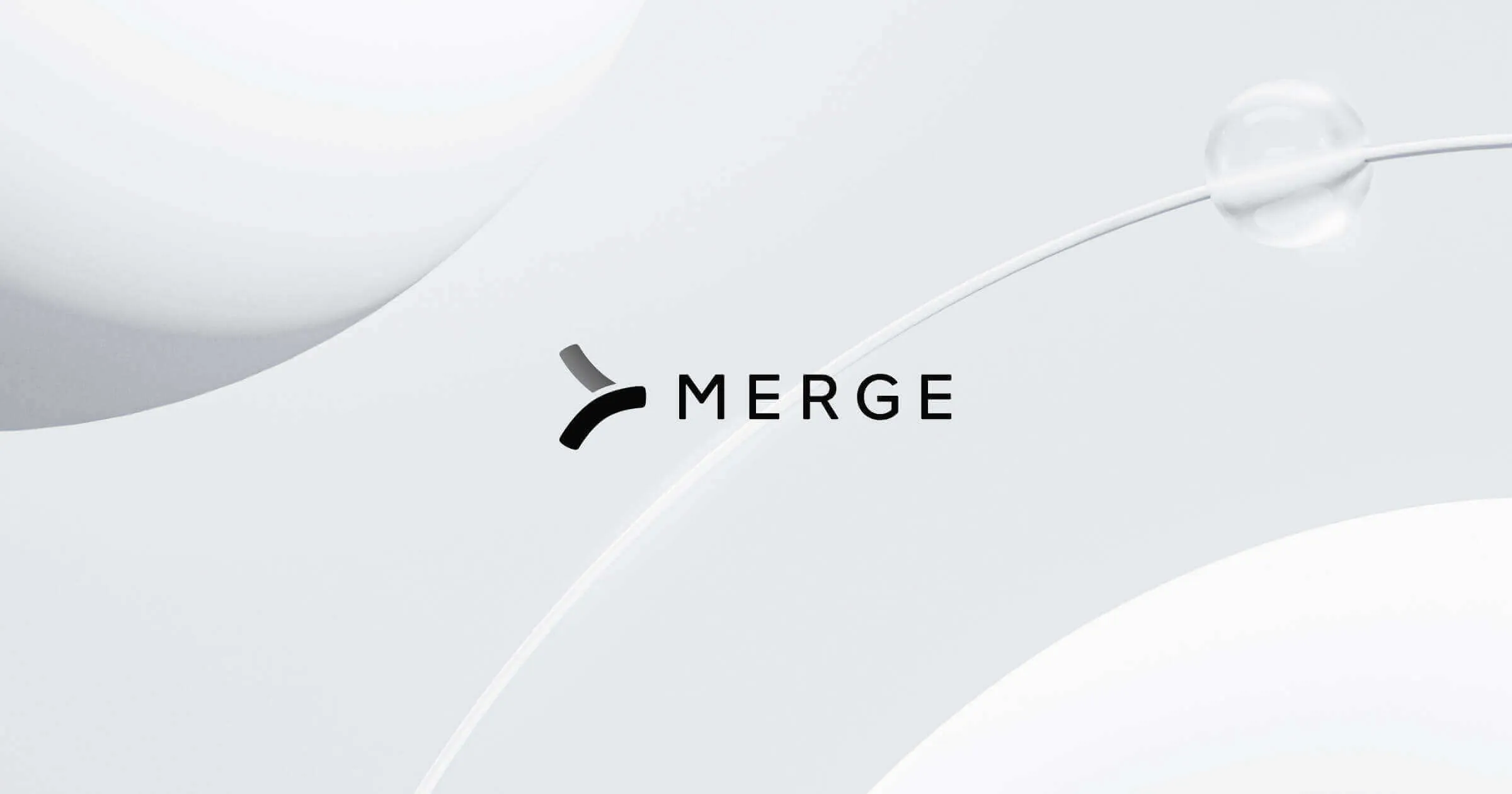
Factorial is an advanced HRIS solution that's engineered to optimize and automate critical HR tasks such as employee data management, time tracking, and benefits administration. The platform also incorporates an API, empowering developers to extract vital employee data into their own systems or applications for personalized management and reporting.
To help you get employees from Factorial using Python, we'll cover every step you need to follow below.
Authentication configuration
Before you can pull data from the Factorial API, you need to set up OAuth 2.0 authentication. This integration uses the authorization code grant type.
Firstly, direct your users to the authorization URL <code class="blog_inline-code">https://api.factorialhr.com/oauth/authorize</code> to start the OAuth flow. Once the user completes the authorization flow, the Factorial API will redirect the user to the redirect URL that you provided. The redirect URL will include the authorization code as a query parameter, <code class="blog_inline-code">code</code>, which you will then use to request an access token.
To request the access token, you need to hit the token URL <code class="blog_inline-code">https://api.factorialhr.com/oauth/token</code> using your client ID and secret provided by Factorial. Include these credentials, along with other required parameters, in the URL as query parameters.
The request header should follow this format: <code class="blog_inline-code">Authorization: Bearer {ACCESS-TOKEN}</code>.
Here's where to find important information in the token URL response:
- The <code class="blog_inline-code">access token</code> is in the access_token key.
- The <code class="blog_inline-code">expires_in</code> value tells you how long the token will last.
- The refresh token is in the <code class="blog_inline-code">refresh_token</code> key.
How to get employees from Factorial
The code below will help you pull employee data from the Factorial API by setting up an OAuth flow, getting an access token and using it to make an authorized request to the Factorial API.
First, define your client ID, client secret, and redirect URL:
Then establish the authorization URL and token URL:
Once the user navigates to the authorization URL and authorizes, they're sent to the redirect URL with code as a query parameter. Get the authorization code from the query parameter:
Request the access token:
Get the access token and refresh token from the response:
Use the access token to make authorized API requests:
Print the response:
Remember to replace <code class="blog_inline-code">'your-client-id'</code>, <code class="blog_inline-code">'your-client-secret'</code>, <code class="blog_inline-code">'your-redirect-url'</code>, and <code class="blog_inline-code">'auth-code-from-query-param'</code> with your actual Client ID, Client Secret, Redirect URL, and Authorization code respectively.
You should see the list of employees from Factorial as the output.
Final thoughts
It’s worth remembering that your clients might be using other HRIS solutions, whether that’s Workday, Namely, UKG, etc.
You can offer customer-facing integrations with all of the HRIS solutions your clients use by connecting to Merge's HRIS Unified API.
Learn more about Merge’s Ticketing Unified API, along with the other features and capabilities provided by the platform, by scheduling a demo with one of our integration experts.