How to fetch deal pipelines from HubSpot using Python
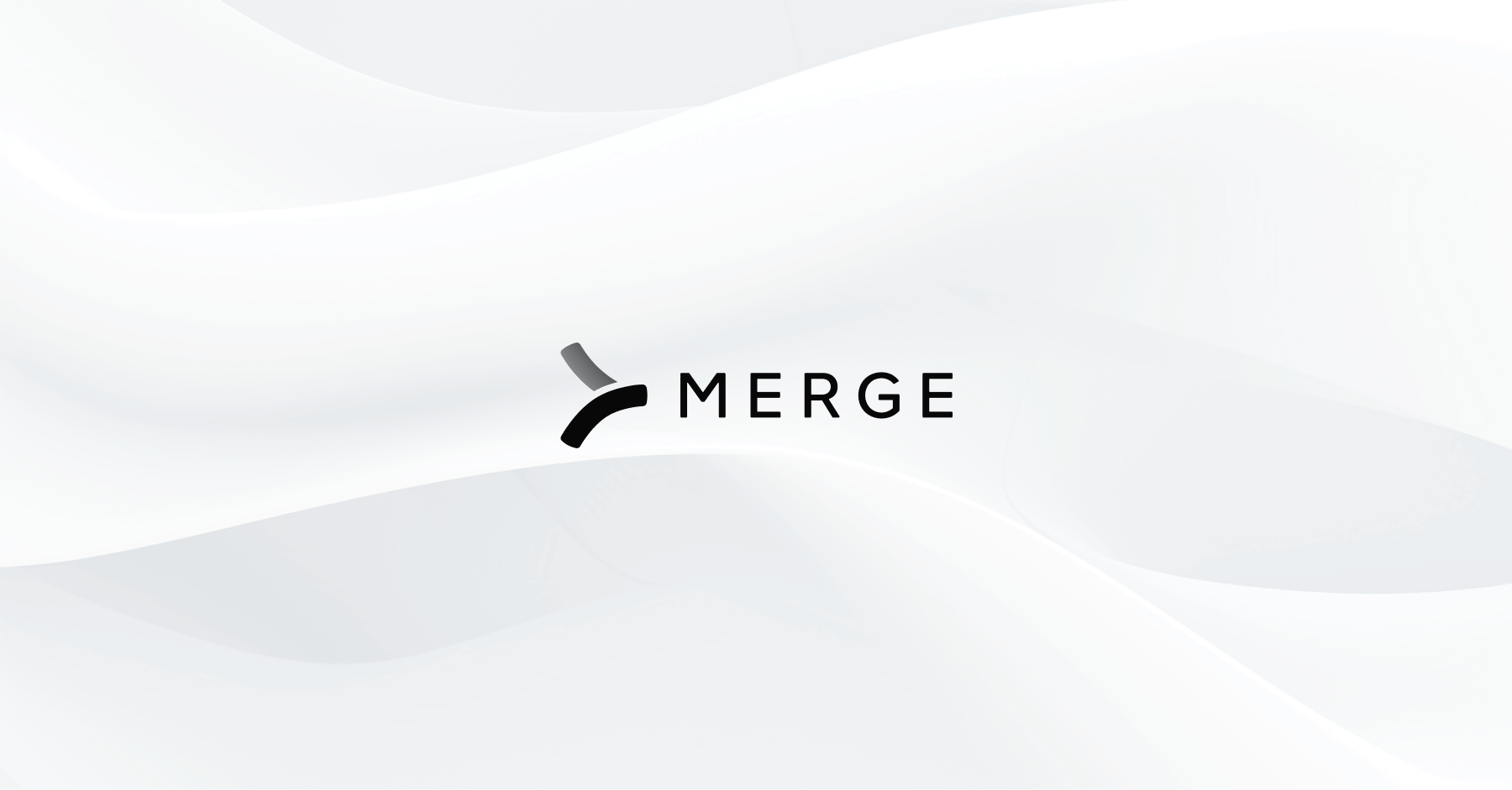
HubSpot is a Customer Relationship Management (CRM) solution for marketing, sales, and customer service needs. It equips teams with tools for everything from social media marketing and content management to lead capture and reporting. It's essentially a comprehensive toolkit to help businesses attract, engage with, and delight customers more effectively.
HubSpot also offers an API that developers can leverage to integrate its suite of services into their applications. This integration facilitates the extraction of data for analysis or customization, enhancing what sales, marketing, and customer success can do.
In our experience, an integration developers find useful involves pulling Deal Pipelines from a customer's HubSpot system. This extraction can provide valuable insights into the customer's sales process, opening up more data-driven decision making. You can also use this integration to boost overall efficiency of marketing operations.
In this article, we’re going to dive into the following key areas:
- Setting up secure authentication for the HubSpot API
- Extracting structured data from HubSpot, specifically using fetch requests to retrieve Deal Pipelines
- Connecting with many third-party CRM platforms using a single API
{{blog-cta-100+}}
Authentication
Hubspot's API supports two methods of authentication: OAuth and Basic Authentication. We will walk through the Basic Authentication method, which is accomplished using an API key -- a unique identifier that authorizes your application to access the HubSpot data.
This API key is included in the header of your HTTP request in the format <code class='blog_inline-code'>Authorization: Bearer {API-KEY}</code>, where <code class='blog_inline-code'>{API-KEY}</code> is the actual key provided by HubSpot.
Fetching deal pipelines with the Hubspot API
Now let's jump into actually getting Deal Pipelines from Hubspot!
This Python script uses the <code class='blog_inline-code'>requests</code> library to send a GET request to the mentioned API endpoint. The API key is included in the header.
The function <code class='blog_inline-code'>fetch_deal_pipelines</code> sends a GET request to the provided URL and parses the JSON response to extract the deal pipelines. It also checks for the existence of a next page using the cursor in the response. If a next page exists, it recursively fetches the deal pipelines from the next page by calling itself with the next page's URL. The deal pipelines from all pages are concatenated and returned.
For reference, this is example of an individual item returned by this API Endpoint:
Leverage a unified CRM API for seamless integrations
In this article, we walked through the process of leveraging Python to connect with HubSpot and retrieve deal pipelines. But what if your clients use a variety of CRM systems?
You can integrate your product with all of your clients' CRM solutions to sync deal pipelines—among other types of data—by leveraging Merge, the leading Unified API solution.
Simply build to Merge's CRM Unified API to offer 20+ CRM integrations. You'll also be able to access Merge's robust Integrations Management features to identify and troubleshoot issues quickly and successfully.
You can learn more about Merge by scheduling a demo with one of our integration experts.