How to get jobs with a unified API (with an example from BambooHR)
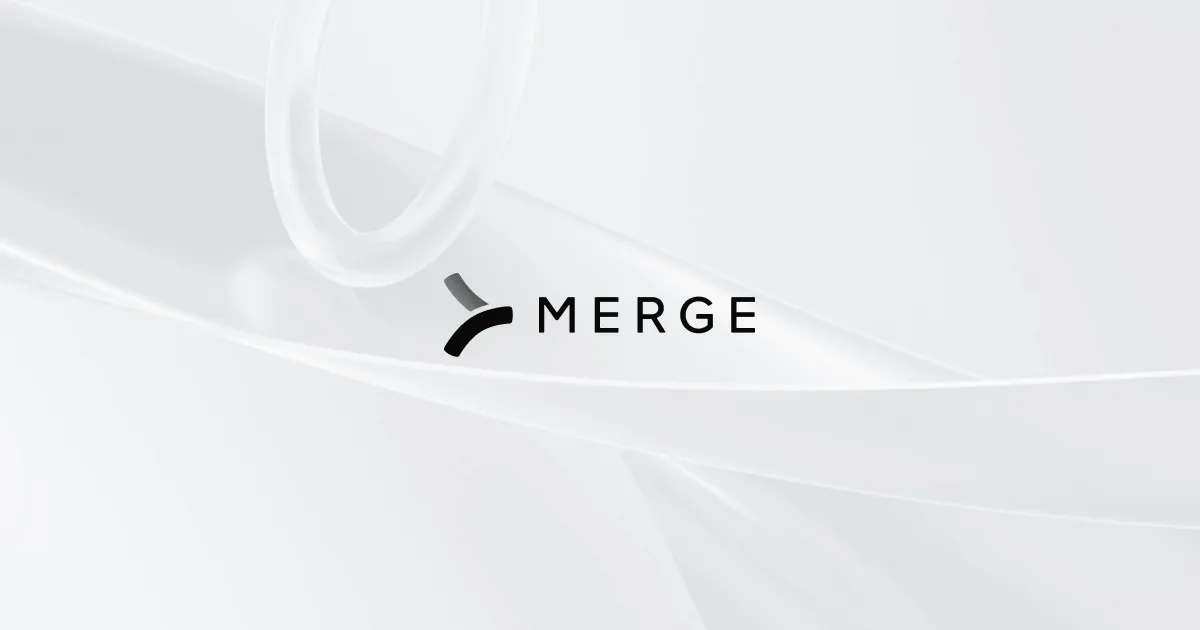
Applicant tracking systems (ATSs) are software that helps hiring professionals manage the hiring process. Instead of sifting through stacks of applications, ATSs filter and categorize applications based on keywords, skills, work experience, and education.
Because of the valuable data in ATSs, companies often want to integrate their ATS with other software programs. One of the most common use cases for such an integration is the retrieval of jobs data. This data can be used to update job postings on your company's website or app in real time to ensure that potential applicants always have access to the most current opportunities.
In this article, you'll see how you can use Merge's unified API to easily get jobs from BambooHR, a popular HRIS with an ATS component.
Note: This tutorial uses BambooHR because you can follow this tutorial using its free trial. However, you can easily adapt these steps to integrate with any of the other ATSs Merge supports, including dedicated ATSs such as Greenhouse and ApplicantStack, as well as HRIS solutions with an ATS component, such as Sage HR.
You can find the code for this tutorial in this GitHub repository.
Prerequisites
Before you get started, make sure you have the following installed to follow along with this tutorial:
- Python 3.10 or later
- A Merge account. You can sign up for free if you don't have one.
- A BambooHR account. You can sign up for a free trial and use BambooHR's trial data for this tutorial.
- A text editor or integrated development environment (IDE) of your choice where you'll write and run your Python code. Popular options include Visual Studio Code and PyCharm.
This tutorial will use Visual Studio Code. If you want to install it, open your shell or terminal and run the following command to install virtualenv: <code class="blog_inline-code">pip3 install virtualenv</code>
Create a directory for your project named merge-bamboohr, navigate to it using your shell or terminal, and create a virtual environment named merge_bamboohr.
<code class="blog_inline-code">mkdir merge-bamboohr && cd merge-bamboohr
virtualenv merge_bamboohr</code>
Activate the virtual environment using the appropriate command for your operating system.
For Windows, use the following: <code class="blog_inline-code">merge_bamboohr\Scripts\activate</code>
For macOS and Linux, use the following: <code class="blog_inline-code">source merge_bamboohr/bin/activate</code>
Lastly, install the Python libraries that will be used in the code. Open your shell or terminal and run the following commands:<code class="blog_inline-code">pip3 install pandaspip3 install MergePythonClient==0.2.4</code>
Pandas will be used to efficiently handle and manipulate tabular data, specifically the jobs data that you will retrieve from BambooHR. The MergePythonClient v0.2.4 will be used to interact with the Merge unified API.
Configure the Authentication between Merge and BambooHR
To integrate Merge with BambooHR, you need to configure the authentication between these tools.
Log in to your Merge account, where you'll see the Merge dashboard.
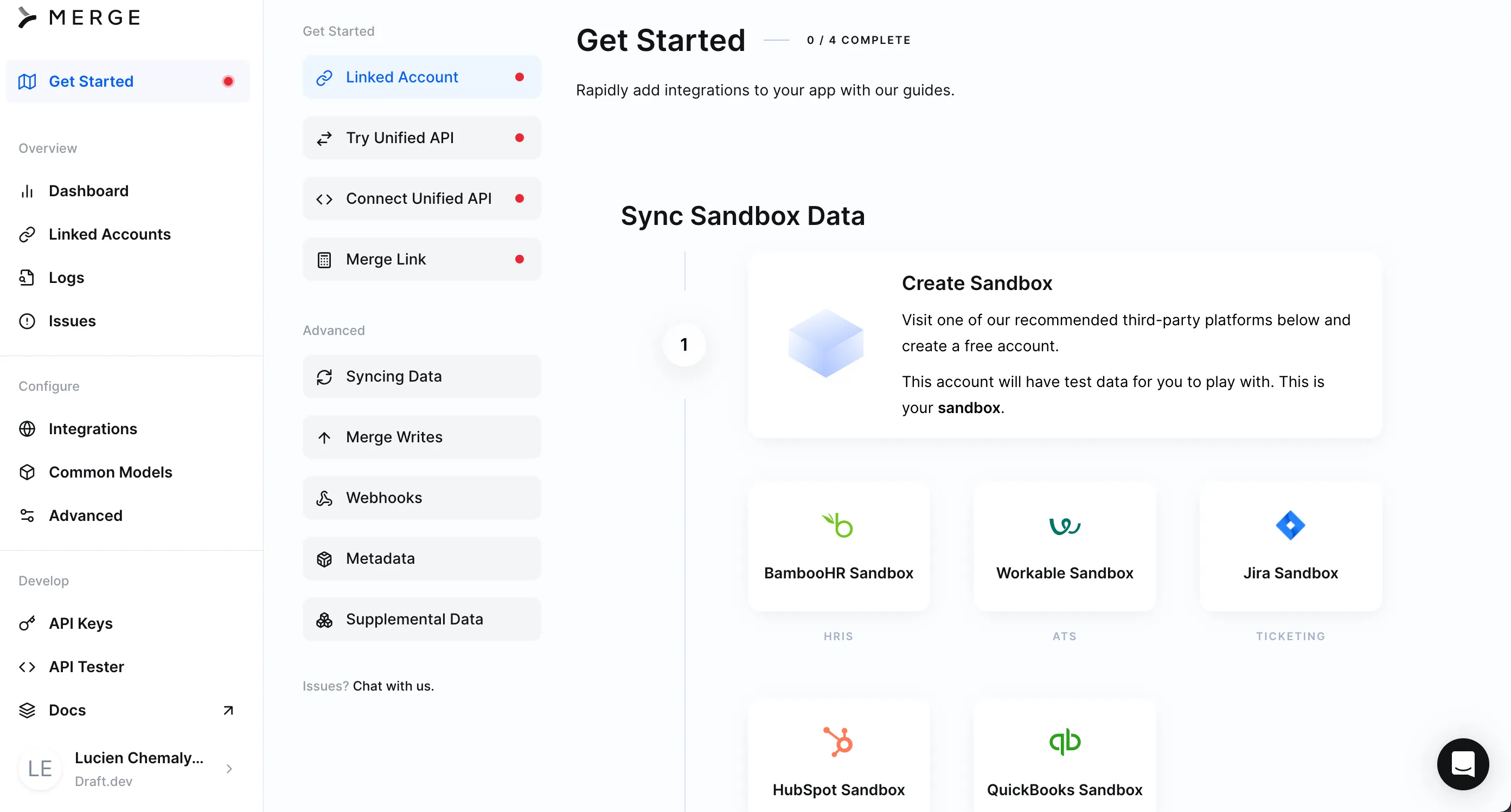
Click on Linked Accounts in the left navigation bar and then on Test Linked Accounts.
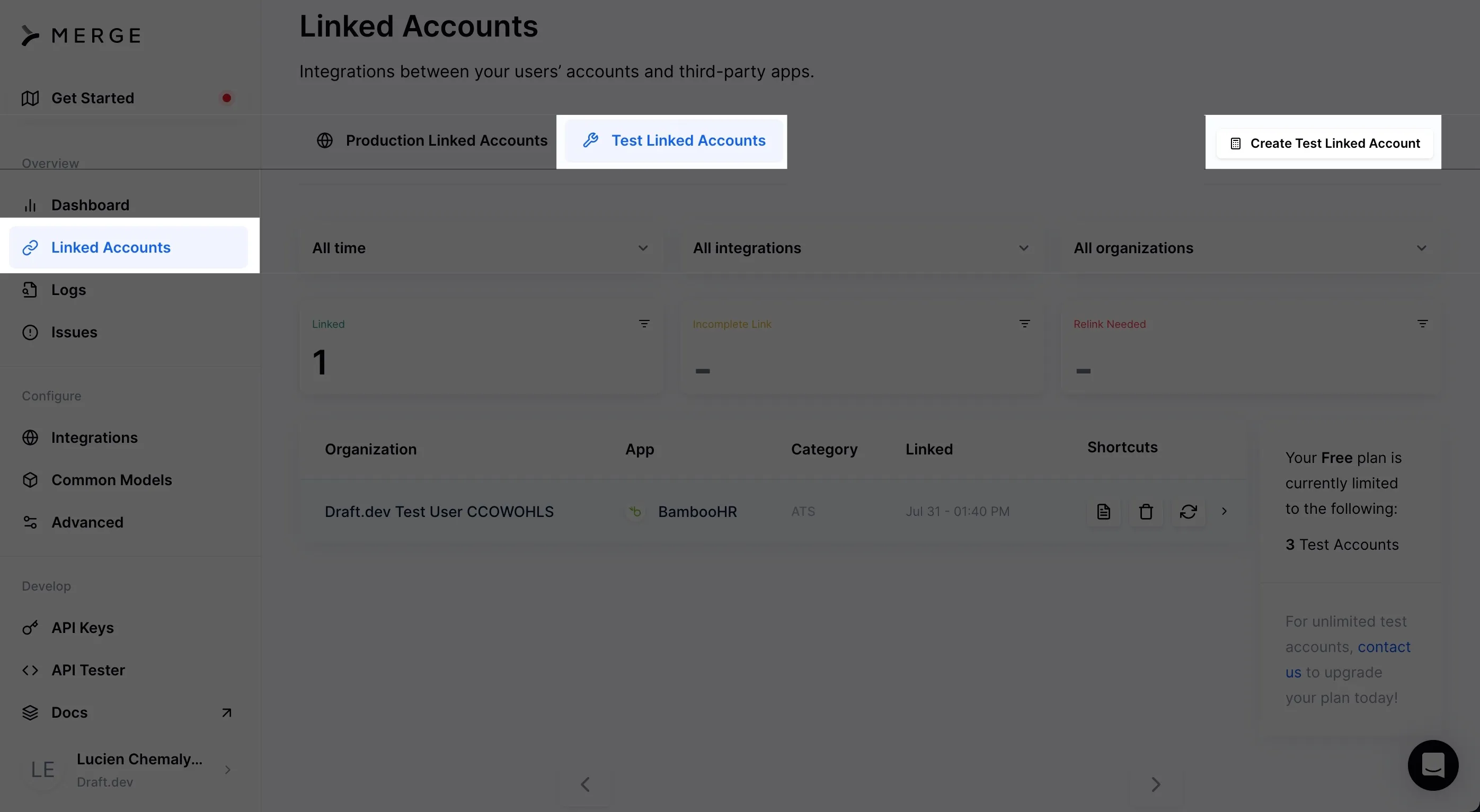
Click on Create Test Linked Account. In the pop-up, select the Applicant Tracking System category and then BambooHR from the list. Click Continue.
Next, you'll be asked how you would like to authenticate. Select the recommended option of authenticating through BambooHR's website and click Next.

Next, you will be asked to enter your BambooHR subdomain. You can find this information in the URL when you're logged into your BambooHR account. It should look like the following: {subdomain}.bamboohr.com.
Type in your subdomain and click Submit.
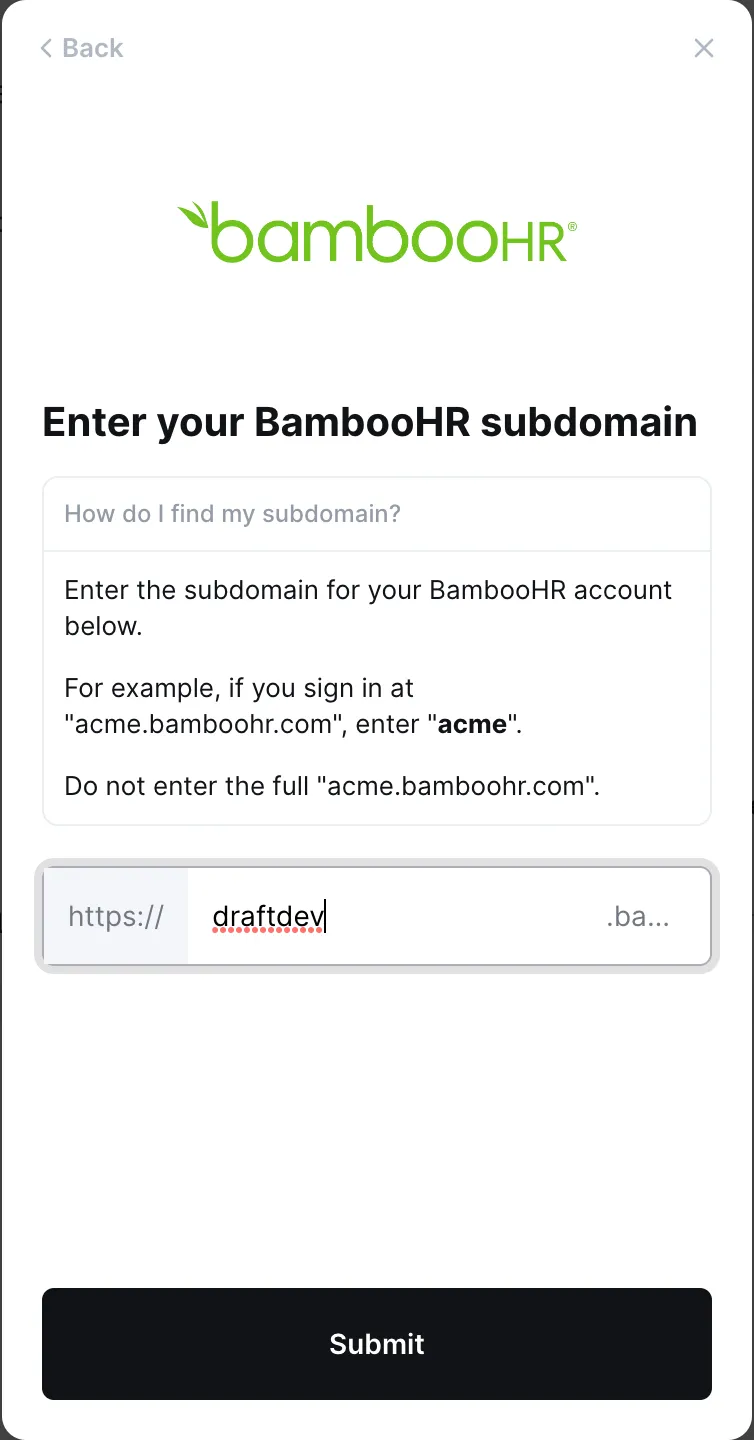
Follow the instructions to finish the setup. You should now see the linked account on your Merge dashboard.

Data for production links are synced automatically. However, since this is a test linked account, you need to sync the data manually. To do so, click on the Force resync icon next to the trash can icon.
Get Merge Keys
Before writing your code, you need to get your account token and your Merge access key. You will use these keys in your code to authenticate with your Merge account and get data from the linked account.
In your Merge dashboard, go to your Linked Accounts and open the test linked account that you just created. On the right side, you should see Account Token.
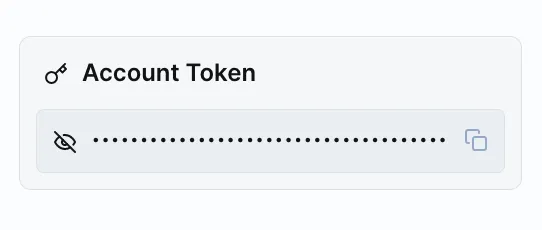
Copy and safely store this key for later use.
Next, click on API Keys in the left navigation bar. Copy Default Test Key, which is under Test Access Keys, and safely store it for later use.
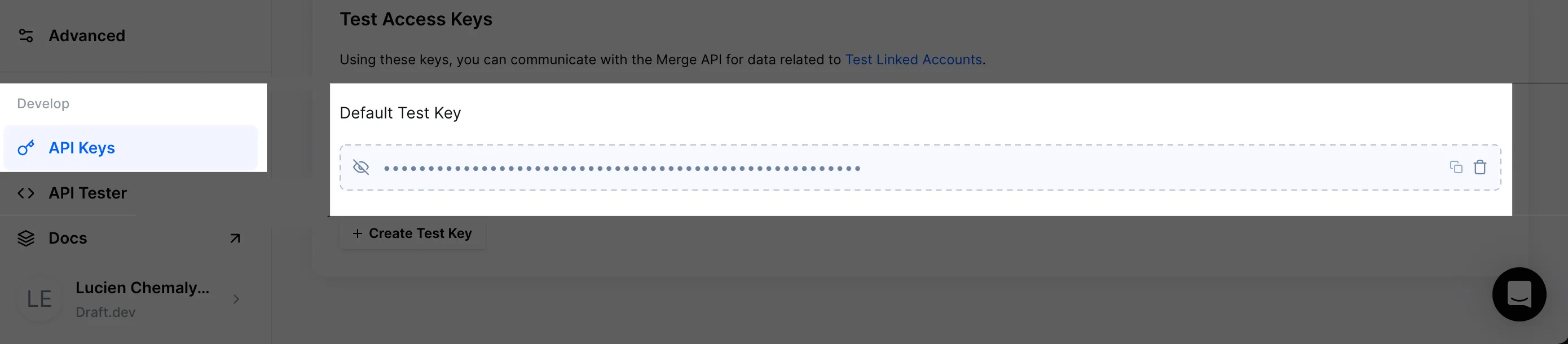
Fetch Jobs from BambooHR
You're now ready to write your Python code to fetch jobs data from BambooHR.
Create a new file named bamboohr_jobs.py and add the following code to it:
This code uses the Merge ATS API to retrieve jobs and write the results to a CSV file.
It starts by creating the Merge client and then calls the jobs method that is in the ats product type, after which it parses the output as a list with the list() function. If the process is successful, job details are written to bamboohr_jobs_results.csv with columns Name, Status, and Created At. If there's an exception, an error message will be printed.
Note: Replace 'YOUR_MERGE_API_KEY' and 'YOUR_MERGE_LINKED_ACCOUNT_TOKEN' with the Merge API key and linked account key you obtained in the previous section.
Run your code from the terminal or shell with the following command:<code class="blog_inline-code">python3 bamboohr_jobs.py</code>
After the code finishes running, you should see a new CSV file named bamboohr_jobs_results.csv. If you used BambooHR's sample data, the content of the file should be similar to the following:
Name,Status,Created At
Customer Success Advocate,Filled,2023-03-30T21:25:15Z
IT Security Engineer,OPEN,2023-08-02T20:20:12Z
Account Executive,On Hold,2023-03-30T21:31:31Z
Videographer,DRAFT,2023-07-31T04:40:38Z
General Application,OPEN,2023-07-08T21:43:35Z
Software Engineer,OPEN,2023-08-02T20:22:25Z
Marketing Manager,OPEN,2023-08-02T16:05:02Z
Financial Analyst,OPEN,2023-08-02T20:21:18Z
Filter Jobs
If you want to filter the jobs that have an OPEN status, you can do so by adding a query parameter.
Create a new file named bamboohr_jobs_open.py. Use the same code as before but add the status parameter to the list() method and name your CSV file bamboohr_jobs_open_results.csv. Your code should look like this:
Run your code from the terminal or shell with the following command:<code class="blog_inline-code">python3 bamboohr_jobs_open.py</code>
After the code finishes running, you should see a new CSV file named bamboohr_jobs_open_results.csv. The content of the file should be similar to the following:
Name,Status,Created At
IT Security Engineer,OPEN,2023-08-02T20:20:12Z
General Application,OPEN,2023-07-08T21:43:35Z
Software Engineer,OPEN,2023-08-02T20:22:25Z
Marketing Manager,OPEN,2023-08-02T16:05:02Z
Financial Analyst,OPEN,2023-08-02T20:21:18Z
Conclusion
As you saw in this tutorial, Merge's unified API makes it very simple to get jobs from an ATS like BambooHR. You can also use the same integration to pull other data from BambooHR, such as activities, applications, jobs, attachments, departments, and more.
Merge also offers integrations with several other ATS systems as well as HR, payroll, CRM, ticketing, and accounting systems. You can sign up for a free trial to explore everything Merge has to offer.